How to Use the strdup Function in C
- What is the strdup Function?
- Using strdup in Your C Program
- Error Handling with strdup
- Memory Management Considerations
- Conclusion
- FAQ
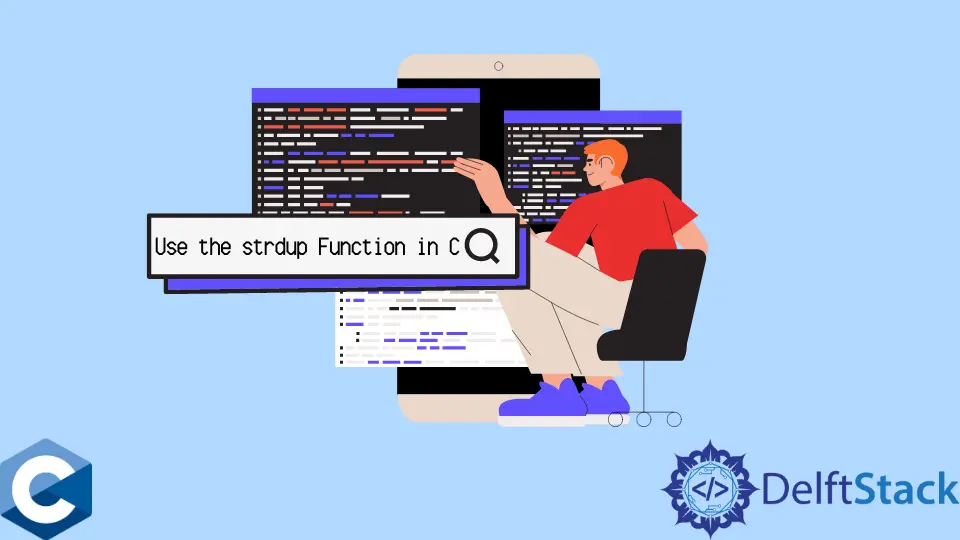
When it comes to programming in C, memory management is a crucial aspect that developers must handle with care. One function that can simplify string duplication is strdup
. This function allows you to create a new copy of a string, allocating the necessary memory automatically.
In this article, we will explore how to effectively use the strdup
function in C, providing you with clear examples and explanations. Whether you’re a beginner or looking to refresh your memory, this guide will help you understand how to leverage strdup
for your string manipulation needs.
What is the strdup Function?
The strdup
function stands for “string duplicate”. It is part of the C standard library and is declared in the header file <string.h>
. The primary purpose of strdup
is to create a duplicate of a given string. This function allocates the required memory for the new string and copies the content from the original string into this newly allocated space.
The syntax for strdup
is straightforward:
char *strdup(const char *s);
Here, s
is the string you want to duplicate. The function returns a pointer to the newly allocated string, or NULL
if the memory allocation fails.
Using strdup in Your C Program
To effectively demonstrate the use of strdup
, let’s walk through a simple C program that duplicates a string and prints both the original and duplicated strings.
Here’s a basic example:
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
int main() {
const char *original = "Hello, World!";
char *duplicate = strdup(original);
if (duplicate == NULL) {
fprintf(stderr, "Memory allocation failed\n");
return 1;
}
printf("Original: %s\n", original);
printf("Duplicate: %s\n", duplicate);
free(duplicate);
return 0;
}
In this code, we first include the necessary headers: stdio.h
for input and output functions, string.h
for the strdup
function, and stdlib.h
for memory management functions. We define a constant character pointer original
pointing to the string we want to duplicate. The strdup
function is then called to create a duplicate, which we store in the duplicate
variable.
After checking if duplicate
is NULL
, which indicates a memory allocation failure, we print both the original and duplicated strings. Finally, we free the allocated memory for the duplicate to avoid memory leaks.
Output:
Original: Hello, World!
Duplicate: Hello, World!
The output shows that both strings are identical, demonstrating that strdup
has successfully created a duplicate of the original string.
Error Handling with strdup
When using strdup
, it’s essential to implement proper error handling to ensure your program remains robust. As shown in the previous example, checking if the return value of strdup
is NULL
can help you handle memory allocation failures gracefully. Here’s a more detailed look at how to implement error handling:
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
int main() {
const char *original = "Memory Management in C";
char *duplicate = strdup(original);
if (duplicate == NULL) {
perror("Failed to duplicate string");
return EXIT_FAILURE;
}
printf("Original: %s\n", original);
printf("Duplicate: %s\n", duplicate);
free(duplicate);
return EXIT_SUCCESS;
}
In this updated code, we use perror
to print a descriptive error message if strdup
fails. This function outputs the last error encountered, providing more context on what went wrong. Additionally, using EXIT_FAILURE
and EXIT_SUCCESS
from stdlib.h
enhances the readability of the exit status.
Output:
Original: Memory Management in C
Duplicate: Memory Management in C
By implementing error handling in this way, you can create more resilient applications that can handle unexpected situations without crashing.
Memory Management Considerations
When using strdup
, it is crucial to remember that the function dynamically allocates memory for the new string. This means you must free that memory when it is no longer needed to prevent memory leaks. Failing to do so can lead to increased memory usage and potentially crash your application over time.
Here’s a reminder of how to manage memory effectively:
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
int main() {
const char *greeting = "Goodbye, World!";
char *copy = strdup(greeting);
if (copy == NULL) {
perror("Memory allocation failed");
return EXIT_FAILURE;
}
printf("Greeting: %s\n", greeting);
printf("Copy: %s\n", copy);
free(copy);
return EXIT_SUCCESS;
}
In this program, after duplicating the string, we ensure to free the allocated memory with free(copy)
before the program exits. This practice is vital for maintaining optimal performance and resource management in C applications.
Output:
Greeting: Goodbye, World!
Copy: Goodbye, World!
By following these memory management guidelines, you can ensure that your use of strdup
is both effective and safe.
Conclusion
The strdup
function is a powerful tool in C for duplicating strings while handling memory allocation automatically. By understanding how to use strdup
, implement error handling, and manage memory effectively, you can enhance your programming skills and create more efficient C applications. Remember to always free any dynamically allocated memory to maintain performance and prevent memory leaks. With this knowledge, you’re well-equipped to handle string duplication in your C programs.
FAQ
-
What does strdup do in C?
strdup duplicates a given string, allocating memory for the new string automatically. -
Is strdup part of the C standard library?
Yes, strdup is included in the C standard library and is declared in the<string.h>
header file. -
How do I handle memory allocation failures with strdup?
Always check if the return value of strdup is NULL to determine if memory allocation failed. -
Do I need to free the memory allocated by strdup?
Yes, you must free the memory using the free function to avoid memory leaks. -
Can I use strdup with any string?
Yes, you can use strdup with any null-terminated string.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook