How to Convert String to Lowercase in C
-
Using the Standard Library Function
tolower()
- Use the Custom Function to Convert String to Lowercase in C
- Using Pointer Arithmetic
- Conclusion
- FAQ
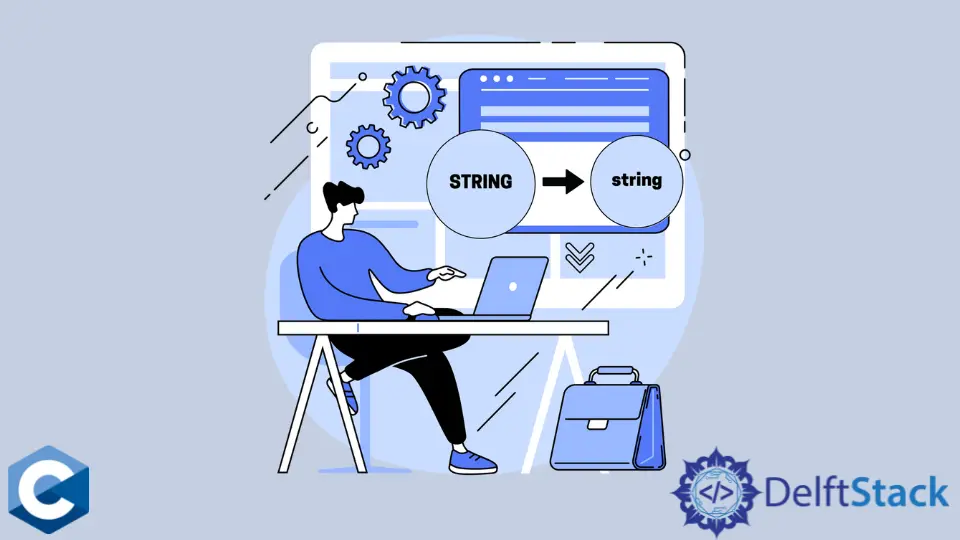
In the world of programming, manipulating strings is a fundamental skill. If you’re working in C, one common task is converting strings to lowercase. This can be particularly useful for tasks like case-insensitive comparisons or simply formatting output.
In this article, we’ll explore various methods to convert a string to lowercase in C, ensuring that you have a solid grasp of the techniques available. Whether you’re a beginner or looking to brush up on your skills, this guide will provide clear explanations and examples to help you understand how to achieve this efficiently.
Using the Standard Library Function tolower()
One of the simplest ways to convert a string to lowercase in C is by using the standard library function tolower()
. This function is part of the ctype.h
library, which provides character handling functions. By iterating through each character of the string and applying tolower()
, you can easily convert the entire string to lowercase.
Here’s how you can do it:
#include <stdio.h>
#include <ctype.h>
void toLowerCase(char *str) {
for (int i = 0; str[i]; i++) {
str[i] = tolower(str[i]);
}
}
int main() {
char str[] = "Hello, World!";
toLowerCase(str);
printf("Lowercase: %s\n", str);
return 0;
}
Output:
Lowercase: hello, world!
In this code, we include the necessary headers and define a function called toLowerCase()
. This function takes a string as input and iterates over each character. The tolower()
function checks if the character is uppercase and converts it to lowercase if necessary. Finally, we print the modified string. This method is efficient and leverages the built-in capabilities of C.
Use the Custom Function to Convert String to Lowercase in C
A more flexible solution would be to implement a custom function that takes the string variable as the argument and returns the converted lowercase string at a separate memory location. This method is essentially the decoupling of the previous example from the main
function.
In this case, we created a function toLower
that takes char*
, where a null-terminated string is stored and a size_t
type integer denoting the string’s length. The function allocates the memory on the heap using the calloc
function; thus the caller is responsible for deallocating the memory before the program exits.
#include <ctype.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
char *toLower(char *str, size_t len) {
char *str_l = calloc(len + 1, sizeof(char));
for (size_t i = 0; i < len; ++i) {
str_l[i] = tolower((unsigned char)str[i]);
}
return str_l;
}
int main() {
char *str = "THIS STRING LITERAL IS ARBITRARY";
printf("%s\n", str);
size_t len = strlen(str);
char *lower = toLower(str, len);
printf("%s", lower);
free(lower);
exit(EXIT_SUCCESS);
}
Output:
THIS STRING LITERAL IS ARBITRARY
this string literal is arbitrary
Using Pointer Arithmetic
For those who enjoy exploring different programming paradigms, pointer arithmetic offers another way to convert a string to lowercase in C. This method can be slightly more complex, but it provides a deeper understanding of how strings are represented in memory.
Here’s how you can implement it:
#include <stdio.h>
#include <ctype.h>
void pointerToLowerCase(char *str) {
char *ptr = str;
while (*ptr) {
*ptr = tolower(*ptr);
ptr++;
}
}
int main() {
char str[] = "Hello, World!";
pointerToLowerCase(str);
printf("Lowercase: %s\n", str);
return 0;
}
Output:
Lowercase: hello, world!
In this implementation, we use a pointer ptr
to traverse the string. The loop continues until we reach the null terminator. The tolower()
function is applied directly to the character pointed to by ptr
, and then the pointer is incremented to move to the next character. This method showcases the flexibility of pointers in C and can be particularly useful in more advanced programming scenarios.
Conclusion
Converting a string to lowercase in C is a crucial skill for any programmer. Whether you choose to use the standard library functions, create your own custom solution, or experiment with pointer arithmetic, the methods outlined in this article will equip you with the knowledge to handle string manipulation effectively. As you continue to explore C programming, mastering these techniques will enhance your ability to write robust and efficient code.
FAQ
-
What is the purpose of converting strings to lowercase in C?
Converting strings to lowercase is useful for case-insensitive comparisons and formatting output. -
Can I convert strings to uppercase in C?
Yes, you can use thetoupper()
function from thectype.h
library to convert strings to uppercase. -
Is it necessary to include
ctype.h
for string manipulation?
It is not strictly necessary, but includingctype.h
gives you access to helpful functions liketolower()
andtoupper()
. -
Can I convert only a part of the string to lowercase?
Yes, you can modify specific indices of the string by applying the conversion functions only to those characters. -
Are there any limitations to the methods discussed?
The methods discussed work well with ASCII characters. For strings containing non-ASCII characters, additional handling may be required.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook