Python math.pi Attribute
- Syntax
-
Example 1: Use
math.pi
to Get the Value ofπ
-
Example 2: Use
math.pi
to Get the Circumference and Area of a Circle -
Example 3: Use
math.pi
to Convert Degrees to Radians -
Example 4: Use
math.pi
to Convert Radians to Degrees
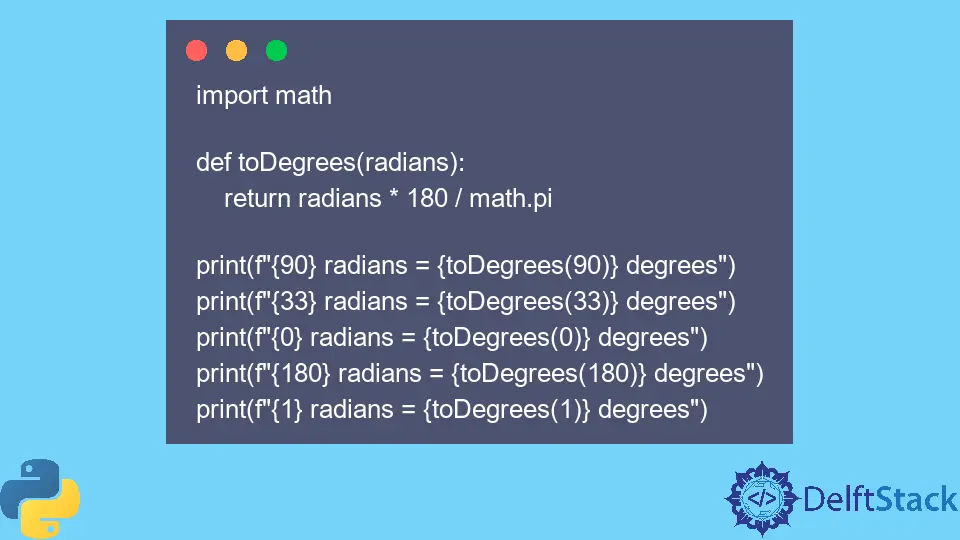
The Python programming language offers a module, math
, that implements a torrent of mathematical methods such as trigonometry, inverse trigonometry and logarithmic functions.
In mathematics, the number π or 3.14159
or 22 / 7
is a mathematical constant that refers to the ratio of a circle’s circumference to its diameter. It is an irrational and non-terminating number used to calculate perimeters, surface areas, and volumes of various shapes such as circles, cylinders, cones, etc.
This article will discuss the pi
value available in the math
module.
Syntax
math.pi
Parameters
Since it is a variable, it does not accept any parameters.
Returns
Since it is a variable, it does not return anything like a method. It stores the value of the mathematical constant π
.
Example 1: Use math.pi
to Get the Value of π
import math
print(math.pi)
Output:
3.141592653589793
Example 2: Use math.pi
to Get the Circumference and Area of a Circle
import math
def area(r):
return math.pi * r * r
def circumference(r):
return 2 * math.pi * r
r = 25
print(f"Circumference: {circumference(r)} units")
print(f"Area: {area(r)} sq. units")
Output:
Circumference: 157.07963267948966 units
Area: 1963.4954084936207 sq. units
The Python code above calculates the circumference and area of a circle with a radius r
. The formula for circumference is 2 * π * r
, and for the area is π * r * r
.
Example 3: Use math.pi
to Convert Degrees to Radians
import math
def toRadians(degrees):
return degrees * math.pi / 180
print(f"{90} degrees = {toRadians(90)} radians")
print(f"{180} degrees = {toRadians(180)} radians")
print(f"{0} degrees = {toRadians(0)} radians")
print(f"{45} degrees = {toRadians(45)} radians")
print(f"{270} degrees = {toRadians(270)} radians")
Output:
90 degrees = 1.5707963267948966 radians
180 degrees = 3.141592653589793 radians
0 degrees = 0.0 radians
45 degrees = 0.7853981633974483 radians
270 degrees = 4.71238898038469 radians
The formula to convert degrees to radians is as follows.
radians = degrees * (π / 180)
The Python code above defines a method, toRadians()
, that accepts an angle in degrees and, using the formula discussed above, it converts it to radians.
Example 4: Use math.pi
to Convert Radians to Degrees
import math
def toDegrees(radians):
return radians * 180 / math.pi
print(f"{90} radians = {toDegrees(90)} degrees")
print(f"{33} radians = {toDegrees(33)} degrees")
print(f"{0} radians = {toDegrees(0)} degrees")
print(f"{180} radians = {toDegrees(180)} degrees")
print(f"{1} radians = {toDegrees(1)} degrees")
Output:
90 radians = 5156.620156177409 degrees
33 radians = 1890.7607239317167 degrees
0 radians = 0.0 degrees
180 radians = 10313.240312354817 degrees
1 radians = 57.29577951308232 degrees
The formula to convert radians to degrees is as follows.
degrees = (180 / π) * radians
The Python code above defines a method, toDegrees()
, that accepts an angle in radians and transforms the input into degrees using the formula we just discussed above.