Python math.nan Attribute
-
Syntax of Python
math..nan
Method -
Example 1: Use
math.nan
to Get the Value ofnan
-
Example 2: Use
math.nan
to Check if a Value IsNaN
-
Example 3: Use
math.nan
With Arithmetic Operations to Check if a Value IsNaN
- Conclusion
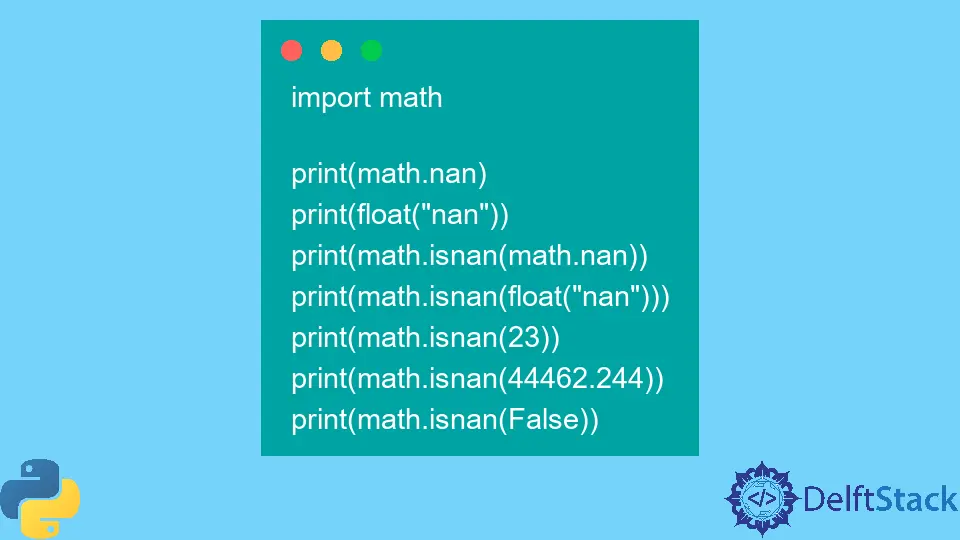
The Python programming language offers a library, math
, that contains implementation for various mathematical operations such as trigonometric functions and logarithmic functions.
In computer science, a NaN
or Not a Number
is a numeric value that can’t be defined or represented. For example, zero divided by zero is undefined; hence, it is considered a NaN
.
This article will discuss the NaN
value in the math
module.
Syntax of Python math..nan
Method
math.nan
Parameters
Since it is a variable, it does not accept any parameters.
Returns
Since it is a variable, it does not return anything like a method. It stores the floating point Not a Number
or NaN
value.
Its value is equivalent to float("nan")
, but the two are not equal.
Example 1: Use math.nan
to Get the Value of nan
import math
print(math.nan)
Output:
nan
In this simple code, we utilize the math
module. Here, we specifically use the nan
attribute from the math
module.
The print(math.nan)
line is a concise way to output the special floating-point value NaN
(Not a Number) to the console. Essentially, NaN
represents a result that is undefined in the realm of numerical operations.
So, in this case, we are using the print
function to display the value of NaN
provided by the math
module. This can be useful when working with mathematical computations where certain operations might lead to indeterminate results, allowing us to recognize and handle such situations in our code.
Example 2: Use math.nan
to Check if a Value Is NaN
import math
print(math.nan)
print(float("nan"))
print(math.isnan(math.nan))
print(math.isnan(float("nan")))
print(math.isnan(23))
print(math.isnan(44462.244))
print(math.isnan(False))
Output:
nan
nan
True
True
False
False
False
In this code, we explore the representation and functionality of the special floating-point value NaN
(Not a Number) using the math
module. First, we print the NaN
value directly using print(math.nan)
.
Next, we employ the float("nan")
expression to create another instance of NaN
and print it. The math.isnan()
function is then applied to both math.nan
and float("nan")
separately, returning True
in both cases, indicating that these values are indeed NaN
.
Subsequently, we test the isnan
function with other non-NaN
values like integers, floats, and a Boolean (False
). As expected, the function correctly identifies that these values are not NaN
, returning False
for each case.
This series of operations demonstrates how the math.isnan()
function efficiently checks whether a given value is the special NaN
in a variety of scenarios.
Example 3: Use math.nan
With Arithmetic Operations to Check if a Value Is NaN
import math
nan_value = math.nan
result_addition = nan_value + 5
result_subtraction = nan_value - 10
result_multiplication = nan_value * 2
result_division = nan_value / 3
print("Result of Addition:", result_addition)
print("Result of Subtraction:", result_subtraction)
print("Result of Multiplication:", result_multiplication)
print("Result of Division:", result_division)
Output:
Result of Addition: nan
Result of Subtraction: nan
Result of Multiplication: nan
Result of Division: nan
In this code, we assign math.nan
to the variable nan_value
. Following that, we perform several arithmetic operations with nan_value
, including addition, subtraction, multiplication, and division.
Each result is then printed to the console. As expected, the output for each operation is NaN
, reflecting the behavior defined by the IEEE 754 standard for floating-point arithmetic.
This example illustrates that arithmetic operations involving NaN
consistently yield NaN
as a result, reinforcing the handling of undefined or indeterminate values in numerical computations.
Conclusion
In conclusion, the math
module in Python provides a comprehensive set of mathematical functions, including the representation of a special numeric value known as NaN
or Not a Number
. This value is particularly useful in scenarios where certain mathematical operations cannot be defined or represented.
The math.nan
variable serves as a convenient way to access this NaN
value within the module. Through the examples presented, we’ve demonstrated its straightforward usage, from obtaining the NaN
value itself to checking if a given value is NaN
and even exploring its behavior in arithmetic operations.
As seen in the outputs, NaN
is appropriately handled in these operations, aligning with the IEEE 754 standard for floating-point arithmetic. Understanding and effectively utilizing math.nan
can enhance the precision and robustness of numerical computations in Python.