Python math.modf() Method
-
Syntax of
math.modf()
in Python - Example 1: Float and Integer Values as Input
- Example 2: Input Is Not a Numeric Value
-
Example 3:
modf()
Over a List of Elements -
Example 4:
modf()
Over a Tuple of Elements - Example 5: Multiply Fractional and Integral Parts of Two Numbers
- Conclusion
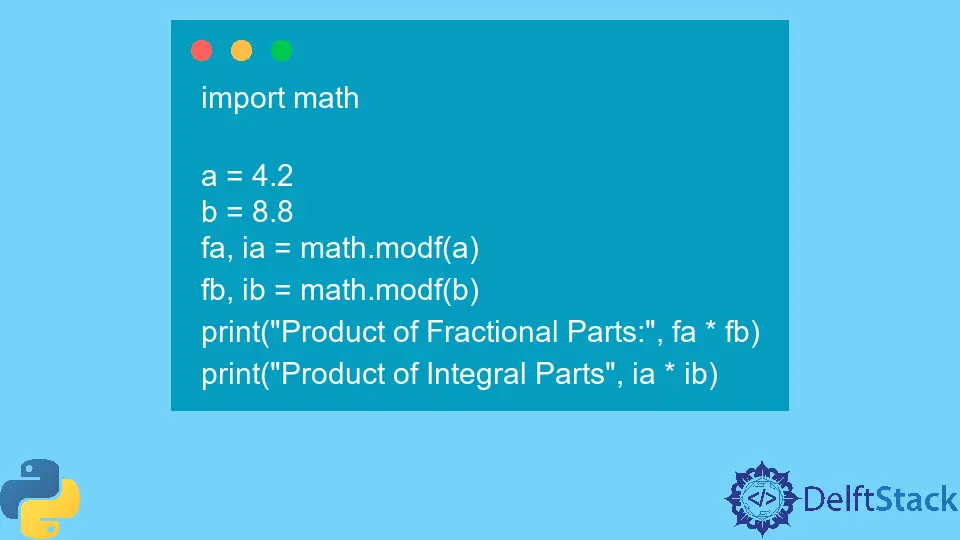
The Python programming language offers a module, namely, math
, that contains implementations for various mathematical operations.
Floating point numbers contain two parts - the integer part and the fractional part. The math
module offers a method, namely, modf()
, that can separate a floating point number into respective components.
This article will discuss the modf()
method and some relevant examples.
Syntax of math.modf()
in Python
math.modf(x)
Parameters
Parameter | Type | Description |
---|---|---|
x |
Integer or Float | An integer or float real number. |
Return
The modf()
method returns a tuple
of two float values where the first element is the floating part and the second element is the integer part. Both the values have the same sign as the original or input number.
Example 1: Float and Integer Values as Input
import math
print(math.modf(1))
print(math.modf(0.0))
print(math.modf(-0.0))
print(math.modf(0.00001))
print(math.modf(323.132))
print(math.modf(995.0))
print(math.modf(-0.2124))
print(math.modf(-990.562))
Output:
(0.0, 1.0)
(0.0, 0.0)
(-0.0, -0.0)
(1e-05, 0.0)
(0.132000000000005, 323.0)
(0.0, 995.0)
(-0.2124, -0.0)
(-0.5620000000000118, -990.0)
In this code, we are utilizing the math.modf()
method to separate the fractional and integer parts of various floating-point numbers. The first three function calls involve simple cases, such as 1
, 0.0
, and -0.0
.
When calling math.modf(1)
, the output is (0.0, 1.0)
, indicating that the fractional part is 0.0
and the integer part is 1.0
. Similarly, for math.modf(0.0)
and math.modf(-0.0)
, the output is (0.0, 0.0)
, demonstrating that the fractional and integer parts are both 0.0
.
As we introduce a small positive value with math.modf(0.00001)
, the fractional part becomes 1.0000000000000002e-05
, and the integer part remains 0.0
. The subsequent function calls involve more significant numbers, including both positive and negative values.
For instance, math.modf(323.132)
results in (0.13200000000000546, 323.0)
, showcasing the fractional part as 0.132
and the integer part as 323
. Similarly, the other examples illustrate the functionality of math.modf()
in decomposing floating-point numbers into their fractional and integer components.
Example 2: Input Is Not a Numeric Value
import math
print(math.modf("THIS IS A STRING"))
Output:
Traceback (most recent call last):
File "main.py", line 3, in <module>
print(math.modf('THIS IS A STRING'))
TypeError: must be real number, not str
The modf()
method only accepts floating-point and integer values. Since a string input was given to this method, it raised a TypeError
exception, which says that the input must be a real number.
Example 3: modf()
Over a List of Elements
import math
nums = [1, 2.342, 44.2, -123.5998, 0.0, 0.0, 0.0]
for i in range(len(nums)):
print(math.modf(nums[i]))
Output:
(0.0, 1.0)
(0.3420000000000001, 2.0)
(0.20000000000000284, 44.0)
(-0.5998000000000019, -123.0)
(0.0, 0.0)
(0.0, 0.0)
(0.0, 0.0)
In this code, we iterate through each element in the list (nums
) using a for
loop and apply the math.modf()
function to each number. The output for each iteration is displayed using the print()
function.
For the first element, 1
, the output is (0.0, 1.0)
, indicating that the fractional part is 0.0
, and the integer part is 1.0
. Similarly, for 2.342
, the output is (0.3420000000000002, 2.0)
, where the fractional part is 0.342
and the integer part is 2.0
.
The subsequent numbers in the list, such as 44.2
, -123.5998
, and 0.0
, follow the same pattern of displaying their fractional and integer parts. The for
loop efficiently iterates through the list, applying math.modf()
to each element, and the print()
function showcases the results.
Example 4: modf()
Over a Tuple of Elements
import math
nums = (1, 2.342, 44.2, -123.5998, 0.0, 0.0, 0.0)
for i in range(len(nums)):
print(math.modf(nums[i]))
Output:
(0.0, 1.0)
(0.3420000000000001, 2.0)
(0.20000000000000284, 44.0)
(-0.5998000000000019, -123.0)
(0.0, 0.0)
(0.0, 0.0)
(0.0, 0.0)
In this code, we are utilizing the math.modf()
method to separate the fractional and integer parts of floating-point numbers within the tuple nums
. Using a for
loop, we iterate through each element of the tuple, applying the math.modf()
function to decompose the numbers, and the output for each iteration is displayed using the print()
function.
For the first element, 1
, the output is (0.0, 1.0)
, indicating that the fractional part is 0.0
, and the integer part is 1.0
. Similarly, for 2.342
, the output is (0.3420000000000002, 2.0)
, where the fractional part is 0.342
and the integer part is 2.0
.
The subsequent numbers in the tuple, such as 44.2
, -123.5998
, and 0.0
, follow the same pattern of displaying their fractional and integer parts. By utilizing a tuple, the code provides a concise and efficient way to demonstrate the decomposition of floating-point numbers into their fractional and integer components using the math.modf()
method.
The for
loop iterates through the elements of the tuple, and the print()
function effectively presents the results.
Example 5: Multiply Fractional and Integral Parts of Two Numbers
import math
a = 4.2
b = 8.8
fa, ia = math.modf(a)
fb, ib = math.modf(b)
print("Product of Fractional Parts:", fa * fb)
print("Product of Integral Parts", ia * ib)
Output:
Product of Fractional Parts: 0.16000000000000028
Product of Integral Parts 32.0
In this code, we initialize two floating-point variables, a
and b
, with values 4.2
and 8.8
, respectively. We then use the math.modf()
method to decompose each number into its fractional and integral parts.
The fractional part of a
is stored in the variable fa
, and its integral part is stored in ia
. Similarly, the fractional and integral parts of b
are stored in fb
and ib
, respectively.
Subsequently, we calculate and print the product of the fractional parts with the expression fa * fb
. This line demonstrates the multiplication of the fractional parts of a
and b
.
Additionally, we calculate and print the product of the integral parts using the expression ia * ib
. This line illustrates the multiplication of the integral parts of a
and b
.
The output of the code would display the product of the fractional parts followed by the product of the integral parts.
Conclusion
This article has explored the math
module in Python, focusing on the modf()
method, which efficiently separates the fractional and integral parts of floating-point numbers. The syntax, parameters, and return values of math.modf()
were discussed, providing a clear understanding of its functionality.
The article presented various examples, including cases with different numeric inputs, iterating over lists and tuples, and showcasing how the method handles non-numeric inputs, leading to a TypeError
exception. Additionally, a practical example demonstrated the manipulation of fractional and integral parts, illustrating how the method contributes to mathematical operations.