Python math.log2() Method
- Syntax
- Example 1: Base-2 Logarithm of a Number
- Example 2: If Input Is Not a Number
-
Example 3: If Input Less Than or Equal to
0
- Example 4: If Input Is Infinity
-
Example 5: If Input Is
NaN
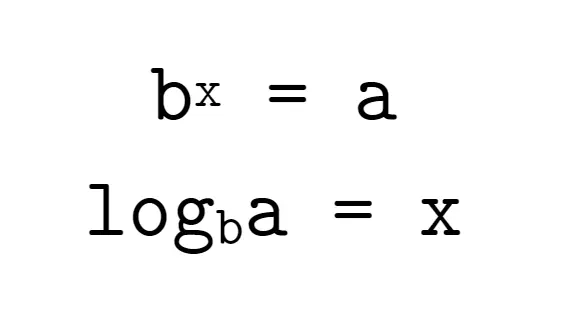
The Python programming language offers a library, math
, that contains implementation for various mathematical operations such as trigonometric functions and logarithmic functions.
Logarithm refers to the inverse function of exponentiation. Simply put, the logarithm of a number a
is the exponent or number (x
) to which another number, the base b
, should be raised to make that number a
.
In this article, we will discuss a method, log2()
, available in the math
module that computes the logarithm of a number with base 2
.
Syntax
math.log2(x)
Parameters
Type | Description | |
---|---|---|
x |
Float | A non-negative integer and non-zero value. |
Return
The log2()
method returns the logarithm to the base of 2
of a number. It is equivalent to math.log(x, 2)
and, in some cases, more accurate than it.
Example 1: Base-2 Logarithm of a Number
import math
print(math.log2(0.000001))
print(math.log2(1))
print(math.log2(0.341))
print(math.log2(99999))
print(math.log2(2352.579))
Output:
-19.931568569324174
0.0
-1.5521563556379145
16.609626047414267
11.200027454372368
The Python code above computes the base-2 logarithm value for 0.000001
, 1
, 0.341
, 99999
, and 2352.579
. Note that these values are non-negative and non-zero.
For inputs in the range (0, 1)
, the base-2 logarithm returns negative results. On the flip side, the range [1, ∞)
produces positive results.
Example 2: If Input Is Not a Number
import math
print(math.log2("this is a string"))
Output:
Traceback (most recent call last):
File "main.py", line 3, in <module>
print(math.log2("this is a string"))
TypeError: must be real number, not str
The Python code above takes a string as an input. Since, the log2()
method expects a number, it raises a TypeError
.
Example 3: If Input Less Than or Equal to 0
import math
print(math.log2(0))
Output:
Traceback (most recent call last):
File "main.py", line 3, in <module>
print(math.log2(0))
ValueError: math domain error
The Python code above takes 0
as input, and since 0
is invalid input, the log2()
method raises a ValueError
.
import math
print(math.log2(-9))
Output:
Traceback (most recent call last):
File "main.py", line 3, in <module>
print(math.log2(-9))
ValueError: math domain error
The Python code above takes a value less than 0
as input, and such values are invalid inputs for the log2()
method. Hence, it raises a ValueError
.
Example 4: If Input Is Infinity
import math
print(math.log2(math.inf))
Output:
inf
When the input is infinity, the result also tends to infinity. This can easily be verified from a logarithm graph to the base of 2
.
Example 5: If Input Is NaN
import math
print(math.log2(math.nan))
Output:
nan