Python math.log1p() Method
- Syntax
-
Example 1: Natural Logarithm of a
number + 1
-
Example 2: If Input Less Than or Equal to
-1
- Example 3: If Input Is Not a Number
- Example 4: If Input Is Infinity
-
Example 5: If Input Is
NaN
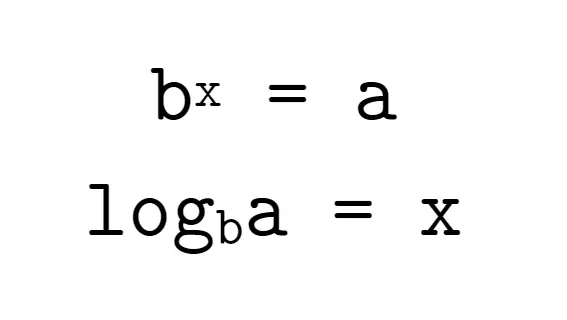
The Python programming language offers a library, math
, that contains implementation for various mathematical operations such as trigonometric functions and logarithmic functions.
Logarithm refers to the inverse function of exponentiation. In simple words, the logarithm of a number a
is the exponent or number (x
) to which another number, the base b
, should be raised to make that number a
.
In this article, we will discuss a method, log1p()
, available in the math
module that computes the logarithm of number + 1
.
Syntax
math.log1p(x)
Parameters
Type | Description | |
---|---|---|
x |
Float | A numeric value that must be greater than -1 . |
Return
The log()
method returns the natural logarithm of x + 1
or logarithm to the base of the mathematical constant e
.
By default, it adds 1
to the number, which means that 0
turns to 1
, and -0.5
turns to 0.5
. Simply put, it calculates math.log(x + 1)
.
Example 1: Natural Logarithm of a number + 1
import math
print(math.log1p(0))
print(math.log1p(1))
print(math.log1p(-0.99999999999999))
print(math.log1p(99999))
print(math.log1p(2352.579))
Output:
0.0
0.6931471805599453
-32.236990899346836
11.512925464970229
7.763692427305668
The Python code above computes the natural logarithm value of x + 1
for 0
, 1
, -0.99999999999999
, 99999
, and 2352.579
. 0
and -0.99999999999999
are valid inputs here because 1
will be added to it.
Hence, they will translate to 1
and 9.992007221626409e-15
, respectively.
Example 2: If Input Less Than or Equal to -1
import math
print(math.log1p(-1))
Output:
Traceback (most recent call last):
File "main.py", line 3, in <module>
print(math.log1p(-1))
ValueError: math domain error
The Python code above takes -1
as input, and since -1
lies outside the domain, the log1p()
method raises a ValueError
.
import math
print(math.log1p(-35.54252))
Output:
Traceback (most recent call last):
File "main.py", line 3, in <module>
print(math.log1p(-35.54252))
ValueError: math domain error
The Python code above takes a value less than -1
as an input, and such values are outside the log1p()
method’s domain. Hence, it raises a ValueError
.
Example 3: If Input Is Not a Number
import math
print(math.log1p("this is a string"))
Output:
Traceback (most recent call last):
File "main.py", line 3, in <module>
print(math.log1p("this is a string"))
TypeError: must be real number, not str
The Python code above takes a string as an input. Since, the log1p()
method expects a number, it raises a TypeError
.
Example 4: If Input Is Infinity
import math
print(math.log1p(math.inf))
Output:
inf
Example 5: If Input Is NaN
import math
print(math.log1p(math.nan))
Output:
nan