Python math.trunc() Method
-
Syntax of Python
math.trunc()
Method -
Example 1: Use the
math.trunc()
Method for Negative Numbers -
Example 2: Use the
math.trunc()
Method for Positive Numbers -
Example 3: Errors When Using the
math.trunc()
Method
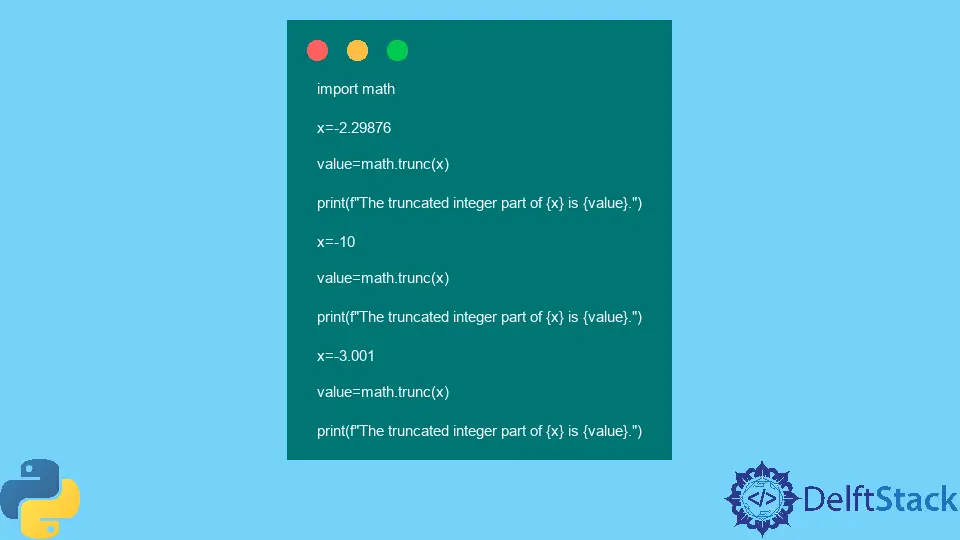
Python math.trunc()
method is an efficient way of truncating the integer part of a number. It behaves as a ceil()
function for negative x
and floor()
function for positive x
.
The same number is returned if the specified number is already an integer.
Syntax of Python math.trunc()
Method
math.trunc(x)
Parameters
x |
Any positive or negative number. |
Return
This method returns an integer representing the truncated integer part of x
.
Example 1: Use the math.trunc()
Method for Negative Numbers
import math
x = -2.29876
value = math.trunc(x)
print(f"The truncated integer part of {x} is {value}.")
x = -10
value = math.trunc(x)
print(f"The truncated integer part of {x} is {value}.")
x = -3.001
value = math.trunc(x)
print(f"The truncated integer part of {x} is {value}.")
Output:
The truncated integer part of -2.29876 is -2.
The truncated integer part of -10 is -10.
The truncated integer part of -3.001 is -3.
Note that the values returned by this method for negative numbers are equivalent to math.ceil()
.
Example 2: Use the math.trunc()
Method for Positive Numbers
import math
x = 3.45
value = math.trunc(x)
print(f"The truncated integer part of {x} is {value}.")
x = 10
value = math.trunc(x)
print(f"The truncated integer part of {x} is {value}.")
x = 132.00006
value = math.trunc(x)
print(f"The truncated integer part of {x} is {value}.")
Output:
The truncated integer part of 3.45 is 3.
The truncated integer part of 10 is 10.
The truncated integer part of 132.00006 is 132.
Note that the values returned by this method for positive numbers are equivalent to math.floor()
.
Example 3: Errors When Using the math.trunc()
Method
import math
# entering a string
x = "Hi"
value = math.trunc(x)
print(f"The truncated integer part of {x} is {value}.")
# entering a list
x = [1, 2, 3]
value = math.trunc(x)
print(f"The truncated integer part of {x} is {value}.")
# entering complex numbers
x = 1 + 5j
value = math.trunc(x)
print(f"The truncated integer part of {x} is {value}.")
# entering an infinite number
x = math.inf
value = math.trunc(x)
print(f"The truncated integer part of {x} is {value}.")
Output:
Traceback (most recent call last):
File "main.py", line 5, in <module>
value=math.trunc(x)
TypeError: type str doesn't define __trunc__ method
Traceback (most recent call last):
File "main.py", line 5, in <module>
value=math.trunc(x)
TypeError: type list doesn't define __trunc__ method
Traceback (most recent call last):
File "main.py", line 7, in <module>
value=math.trunc(x)
TypeError: type complex doesn't define __trunc__ method
Traceback (most recent call last):
File "main.py", line 7, in <module>
value=math.trunc(x)
OverflowError: cannot convert float infinity to integer
The above code snippets show all possible syntactic errors that might occur when using the math.trunc()
method.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn