Python math.tan() Method
-
Syntax of Python
math.tan()
Method -
Example 1: Use the
math.tan()
Method in Python -
Example 2: Use the
math.tan()
Method to Get the Tangent of Degrees -
Example 3: Errors When Using the
math.tan()
Method -
Example 4: Use Trigonometric Functions With the
math.tan()
Method
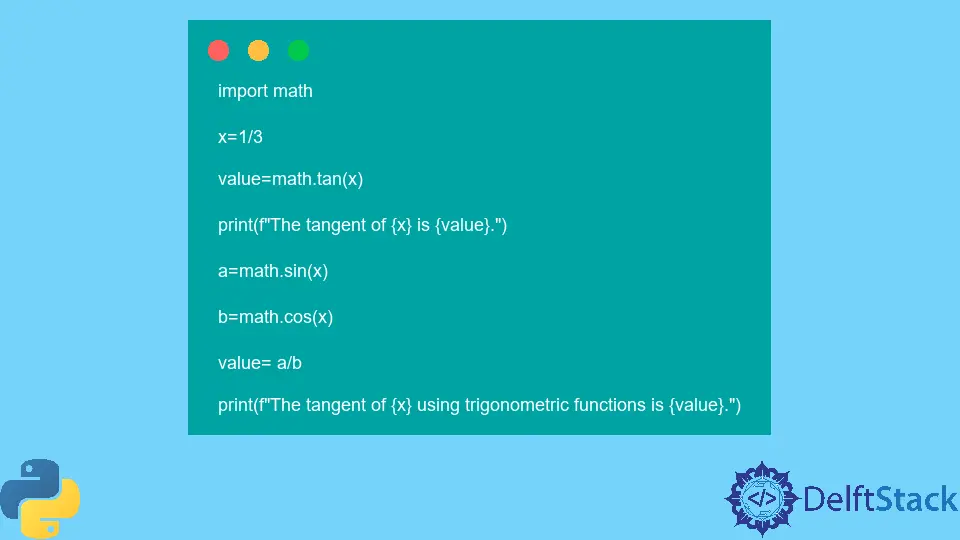
Python math.tan()
method is an efficient way of calculating the tangent of a number in radians. The input parameter must be in radians.
Syntax of Python math.tan()
Method
math.tan(x)
Parameters
x |
Any positive or negative radian value to be operated on. |
Return
This method returns a float value representing the tangent of x
in radians.
Example 1: Use the math.tan()
Method in Python
import math
x = 90
value = math.tan(x)
print(f"The tangent of {x} is {value}.")
x = 0
value = math.tan(x)
print(f"The tangent of {x} is {value}.")
x = -34.5
value = math.tan(x)
print(f"The tangent of {x} is {value}.")
x = math.pi
value = math.tan(x)
print(f"The tangent of {x} is {value}.")
Output:
The tangent of 90 is -1.995200412208242.
The tangent of 0 is 0.0.
The tangent of -34.5 is 0.057582706804865574.
The tangent of 3.141592653589793 is -1.2246467991473532e-16.
Note that the arguments can be in integers or floats. The values may either be positive or negative.
Example 2: Use the math.tan()
Method to Get the Tangent of Degrees
import math
print("The tangent of 30 degrees is ", math.tan(math.radians(30)))
Output:
The tangent of 30 degrees is 0.5773502691896257
We use these methods in mathematical computations related to geometry and have a certain application in astronomical computations.
Example 3: Errors When Using the math.tan()
Method
import math
x = math.inf
value = math.tan(x)
print(f"The tangent of {x} is {value}.")
x = "h"
value = math.tan(x)
print(f"The tangent of {x} is {value}.")
Output:
Traceback (most recent call last):
File "main.py", line 5, in <module>
value=math.tan(x)
ValueError: math domain error
Traceback (most recent call last):
File "main.py", line 9, in <module>
value=math.tan(x)
TypeError: must be real number, not str
Note that a TypeError
exception may occur if the argument value is not a number.
Example 4: Use Trigonometric Functions With the math.tan()
Method
import math
x = 1 / 3
value = math.tan(x)
print(f"The tangent of {x} is {value}.")
a = math.sin(x)
b = math.cos(x)
value = a / b
print(f"The tangent of {x} using trigonometric functions is {value}.")
Output:
The tangent of 0.3333333333333333 is 0.34625354951057546.
The tangent of 0.3333333333333333 using trigonometric functions is 0.34625354951057546.
Note that tan(θ)
is equal to sin(θ)
divided by cosine(θ)
.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn