Python math.sin() Method
-
Syntax of Python
math.sin()
Method -
Example 1: Use the
math.sin()
Method in Python -
Example 2: Use the
math.sin()
Method to Get Sine of Degrees -
Example 3: Errors When Using the
math.sin()
Method
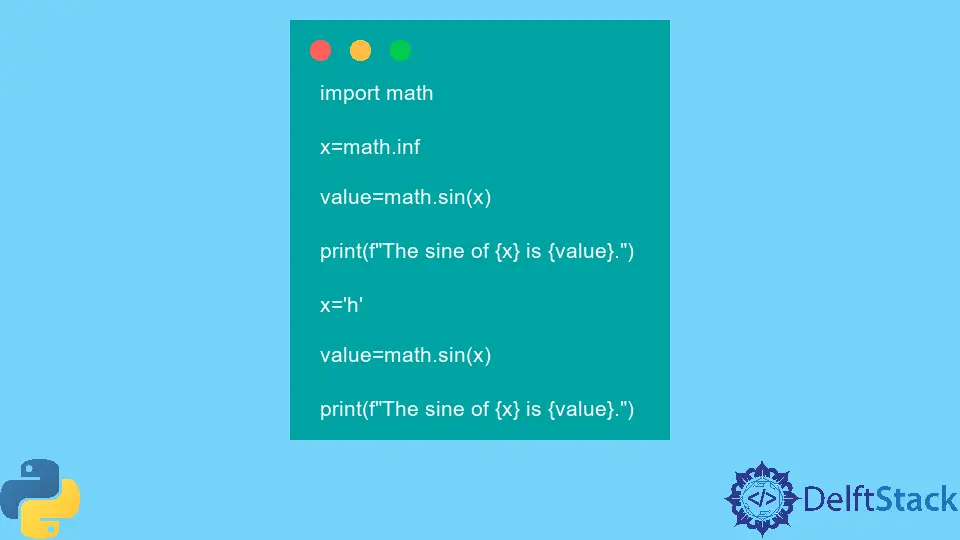
Python math.sin()
method is an efficient way of calculating the sine of a number in radians. The input parameter must be in radians.
Syntax of Python math.sin()
Method
math.sin(x)
Parameters
x |
Any positive or negative radian value to be operated on. |
Return
This method returns a float value, from -1 to 1, representing the sine of x
in radians.
Example 1: Use the math.sin()
Method in Python
import math
x = 1.654
value = math.sin(x)
print(f"The sine of {x} is {value}.")
x = 0
value = math.sin(x)
print(f"The sine of {x} is {value}.")
x = -34.5
value = math.sin(x)
print(f"The sine of {x} is {value}.")
x = math.pi
value = math.sin(x)
print(f"The sine of {x} is {value}.")
Output:
The sine of 1.654 is 0.996540570833053.
The sine of 0 is 0.0.
The sine of -34.5 is -0.057487478104924564.
The sine of 3.141592653589793 is 1.2246467991473532e-16.
Note that the arguments can be in integers or floats. The values may either be positive or negative.
Example 2: Use the math.sin()
Method to Get Sine of Degrees
import math
print("The sine of 30 degrees is ", math.sin(math.radians(30)))
Output:
The sine of 30 degrees is 0.49999999999999994
We use these methods in mathematical computations related to geometry and have a certain application in astronomical computations.
Example 3: Errors When Using the math.sin()
Method
import math
x = math.inf
value = math.sin(x)
print(f"The sine of {x} is {value}.")
x = "h"
value = math.sin(x)
print(f"The sine of {x} is {value}.")
Output:
Traceback (most recent call last):
File "main.py", line 5, in <module>
value=math.sin(x)
ValueError: math domain error
Traceback (most recent call last):
File "main.py", line 9, in <module>
value=math.sin(x)
TypeError: must be real number, not str
Note that a TypeError
exception may occur if the argument value is not a number.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn