Python math.radians() Method
-
Syntax of Python
math.radians()
Method -
Example 1: Use the
math.radians()
Method in Python -
Example 2:
TypeError
When Usingmath.radians()
Method -
Example 3: The
math.radians()
Method and Its Inverse
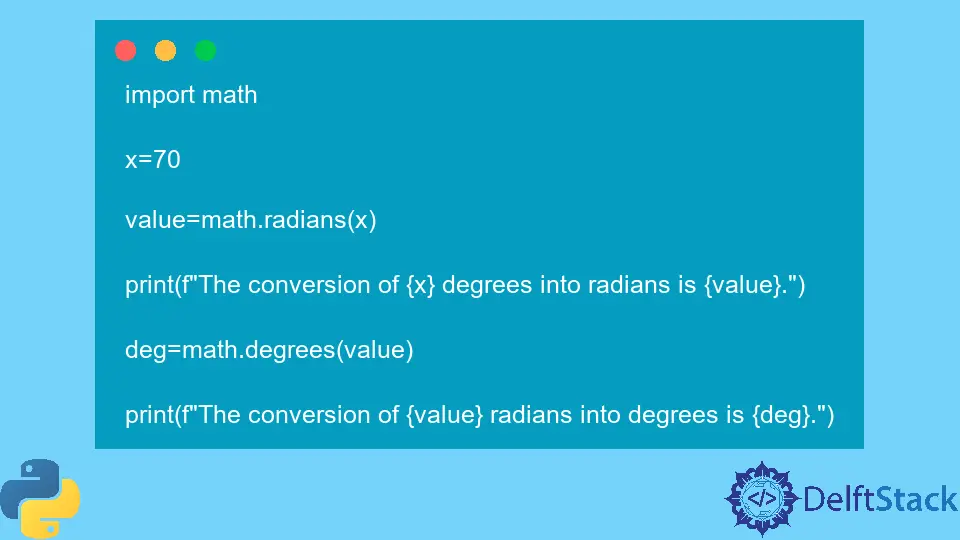
Python math.radians()
method is an efficient way of converting an angle from degrees to radians. Note that 1 degree = π/180 radians
.
Syntax of Python math.radians()
Method
math.radians(deg)
Parameters
deg
- Any angle in degrees that must be converted into radians.
Return
This method returns a floating point value representing an angle in radians.
Example 1: Use the math.radians()
Method in Python
import math
x = 70
value = math.radians(x)
print(f"The conversion of {x} degrees into radians is {value}.")
x = -109
value = math.radians(x)
print(f"The conversion of {x} degrees into radians is {value}.")
x = math.pi
value = math.radians(x)
print(f"The conversion of {x} degrees into radians is {value}.")
x = math.inf
value = math.radians(x)
print(f"The conversion of {x} degrees into radians is {value}.")
x = 0
value = math.radians(x)
print(f"The conversion of {x} degrees into radians is {value}.")
Output:
The conversion of 70 degrees into radians is 1.2217304763960306.
The conversion of -109 degrees into radians is -1.9024088846738192.
The conversion of 3.141592653589793 degrees into radians is 0.05483113556160755.
The conversion of inf degrees into radians is inf.
The conversion of 0 degrees into radians is 0.0.
Note that the values may either be positive or negative. These methods are used in mathematical computations related to geometry and have a certain application in astronomical computations.
Example 2: TypeError
When Using math.radians()
Method
import math
# entering a string
x = "Hi"
value = math.radians(x)
print(f"The conversion of {x} degrees into radians is {value}.")
# entering a list
x = [1, 2, 3]
value = math.radians(x)
print(f"The conversion of {x} degrees into radians is {value}.")
# entering complex numbers
x = 1 + 5j
value = math.radians(x)
print(f"The conversion of {x} degrees into radians is {value}.")
Output:
Traceback (most recent call last):
File "main.py", line 7, in <module>
value=math.radians(x)
TypeError: must be real number, not str
Traceback (most recent call last):
File "main.py", line 15, in <module>
value=math.radians(x)
TypeError: must be real number, not list
Traceback (most recent call last):
File "main.py", line 22, in <module>
value=math.radians(x)
TypeError: can't convert complex to float
The above code snippets show all possible syntactic errors that might occur when using the math.radians()
method.
Example 3: The math.radians()
Method and Its Inverse
import math
x = 70
value = math.radians(x)
print(f"The conversion of {x} degrees into radians is {value}.")
deg = math.degrees(value)
print(f"The conversion of {value} radians into degrees is {deg}.")
Output:
The conversion of 70 degrees into radians is 1.2217304763960306.
The conversion of 1.2217304763960306 radians into degrees is 70.0.
The angle can be either an integer or floating point value.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn