Python math.isinf() Method
-
Syntax of Python
math.isinf()
Method -
Example Code 1: Use of the
math.isinf()
Method -
Example Code 2: Use of the
math.isinf()
Method - Conclusion
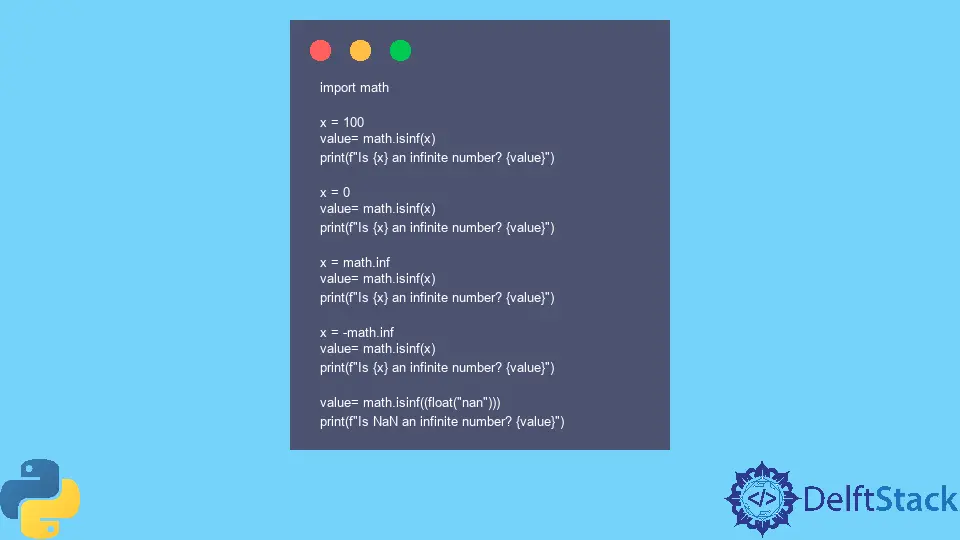
Python math.isinf()
method is an efficient way of finding whether a number is infinite or not. It returns True
if the number is infinite (it can be positive or negative infinity); otherwise, False
.
Syntax of Python math.isinf()
Method
math.isinf(x)
Parameters
Parameter | Description |
---|---|
x |
The number to check can be positive or negative. |
Returns
The return type for this method is a Boolean value. True
is returned if x
is an infinite value; otherwise, False
.
Example Code 1: Use of the math.isinf()
Method
Example Code:
import math
x = 100
value = math.isinf(x)
print(f"Is {x} an infinite number? {value}")
x = 0
value = math.isinf(x)
print(f"Is {x} an infinite number? {value}")
value = math.isinf((float("nan")))
print(f"Is NaN an infinite number? {value}")
Output:
Is 100 an infinite number? False
Is 0 an infinite number? False
Is NaN an infinite number? False
In this code, we use Python’s math.isinf()
method to check if specific numeric values are infinite. First, we evaluate whether 100
is infinite (False
).
Then, we check if 0
is infinite (False
as well). In the third part, we examine the method’s behavior with a non-numeric value, float("nan")
, confirming that it’s not an infinite number (False
).
The concise output in the console affirms these results, showcasing the effectiveness of the math.isinf()
method in accurately identifying infinite and non-infinite values.
Example Code 2: Use of the math.isinf()
Method
import math
num1 = float("inf")
result1 = math.isinf(num1)
print(f"Is {num1} an infinite number? {result1}")
num2 = float("-inf")
result2 = math.isinf(num2)
print(f"Is {num2} an infinite number? {result2}")
Output:
Is inf an infinite number? True
Is -inf an infinite number? True
In this code, we set num1
to positive infinity using float('inf')
and then use the math.isinf()
method to check if it is an infinite number. The result, displayed using an f-string, confirms that positive infinity is indeed an infinite number (True
).
Subsequently, we set num2
to negative infinity with float('-inf')
and repeat the process. The output, once again presented through an f-string, validates that negative infinity is also identified as an infinite number (True
).
The output in the console succinctly communicates these outcomes, providing clarity on the infinite nature of both positive and negative infinity. This example illustrates the straightforward application of the math.isinf()
method for accurately determining whether a given numeric value represents infinity in Python.
Conclusion
In conclusion, the Python math.isinf()
method proves to be a valuable tool for efficiently determining whether a given number is infinite. By returning a Boolean value, True
for infinite and False
for finite, the method facilitates straightforward and concise checks.
Its usage, as illustrated in the provided examples, extends to positive and negative infinity as well as non-infinite numbers. This functionality contributes to the versatility and reliability of Python when handling numerical data, ensuring clarity in discerning infinite values within a program.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn