Python math.isclose() Method
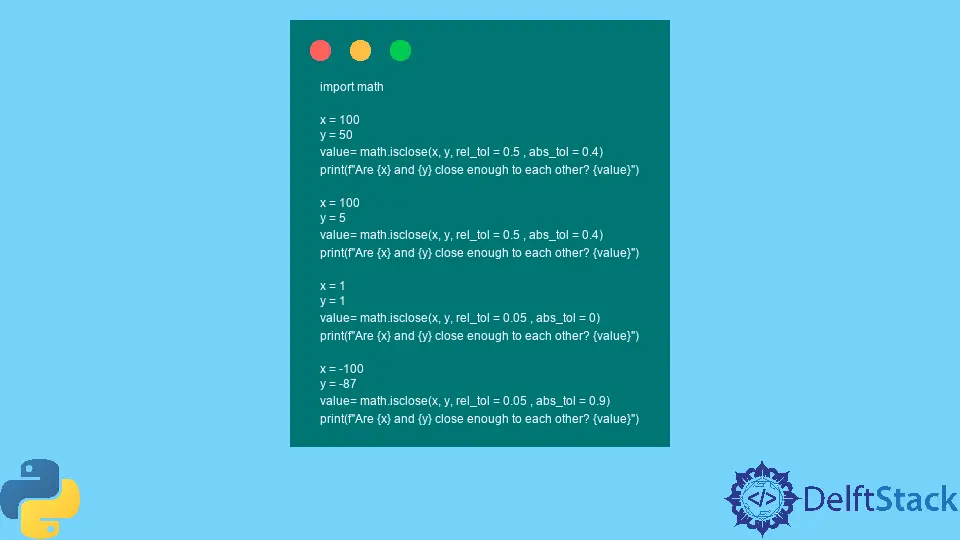
Python math.isclose()
method is an efficient way to find whether the two specified values are close to each other or not. To measure the closeness, it uses relative and absolute tolerances.
The math.isclose()
uses the following formula to compare the specified two values:
abs(x-y) <= max(rel_tol * max(abs(x), abs(y)), abs_tol)
Syntax
math.isclose(x, y) # with default tolerances
math.isclose(x, y, rel_tol, abs_tol) # without default tolerances
Parameters
x |
A positive or negative number. |
y |
A positive or negative number. |
rel_tol |
(Optional) The maximum difference for being considered close relative to the magnitude of x and y . The default value is 1e-09. |
abs_tol |
(Optional) The maximum difference for being considered close , regardless of the magnitude of x and y . The default value is 0. |
Returns
The math.isclose()
returns True
if x
and y
are close to each other in terms of the specified conditions; otherwise, False
.
Example Codes
Let’s learn the use of the math.isclose()
method with/without default tolerances.
Use the math.isclose()
Method With Custom Tolerances
Example Code:
import math
x = 100
y = 50
value = math.isclose(x, y, rel_tol=0.5, abs_tol=0.4)
print(f"Are {x} and {y} close enough to each other? {value}")
x = 100
y = 5
value = math.isclose(x, y, rel_tol=0.5, abs_tol=0.4)
print(f"Are {x} and {y} close enough to each other? {value}")
x = 1
y = 1
value = math.isclose(x, y, rel_tol=0.05, abs_tol=0)
print(f"Are {x} and {y} close enough to each other? {value}")
x = -100
y = -87
value = math.isclose(x, y, rel_tol=0.05, abs_tol=0.9)
print(f"Are {x} and {y} close enough to each other? {value}")
Output:
Are 100 and 50 close enough to each other? True
Are 100 and 5 close enough to each other? False
Are 1 and 1 close enough to each other? True
Are -100 and -87 close enough to each other? False
Note that for the entered values to be considered close
, the difference between the two numbers must be smaller than at least one of the tolerances.
Use the math.isclose()
Method With Default Tolerances
Example Code:
import math
x = 49.5
y = 50
value = math.isclose(x, y)
print(f"Are {x} and {y} close enough to each other? {value}")
x = -32
y = -3
value = math.isclose(x, y)
print(f"Are {x} and {y} close enough to each other? {value}")
x = -3
y = -3
value = math.isclose(x, y)
print(f"Are {x} and {y} close enough to each other? {value}")
x = 49.501234567892
y = 49.501234567891
value = math.isclose(x, y)
print(f"Are {x} and {y} close enough to each other? {value}")
x = math.inf
y = 4
value = math.isclose(x, y)
print(f"Are {x} and {y} close enough to each other? {value}")
Output:
Are 49.5 and 50 close enough to each other? False
Are -32 and -3 close enough to each other? False
Are -3 and -3 close enough to each other? True
Are 49.501234567892 and 49.501234567891 close enough to each other? True
Are inf and 4 close enough to each other? False
Note that the default tolerance of this method is 1e-09
, which ensures that the two entered values are identical within about 9
decimal digits.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn