Python math.ldexp() Method
-
Syntax of
math.ldexp()
in Python -
Example Code: Use of the
math.ldexp()
Method -
Example Code: Error While Using the
math.ldexp()
Method -
Example Code: Use Multiple Entries on the
math.ldexp()
Method -
Example Code: The
math.ldexp()
Method and Its Inverse - Conclusion
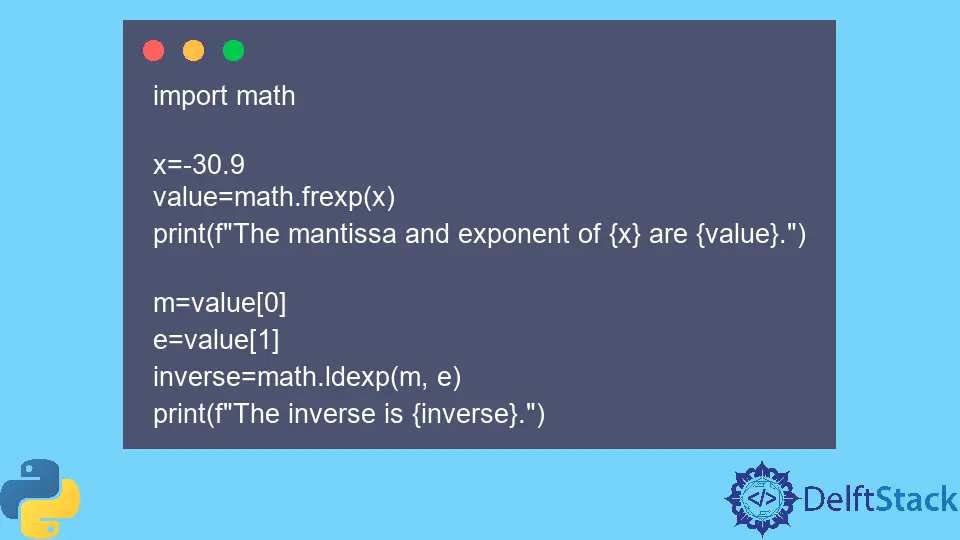
The Python math.ldexp()
method is an efficient way of converting the mantissa and exponent of any number to the original number. The method returns x * (2**i)
.
Syntax of math.ldexp()
in Python
math.ldexp(x, i)
Parameters
Parameter | Description |
---|---|
x |
Any number. Either positive or negative. |
i |
Any number. Either positive or negative. |
Returns
This method’s return type is a floating point value representing x * (2**i)
.
Example Code: Use of the math.ldexp()
Method
import math
x = -30.9
i = 2
value = math.ldexp(x, i)
print(f"The number is {value}.")
x = 0
i = 0
value = math.ldexp(x, i)
print(f"The number is {value}.")
x = 3
i = -8
value = math.ldexp(x, i)
print(f"The number is {value}.")
x = math.inf
i = 1
value = math.ldexp(x, i)
print(f"The number is {value}.")
Output:
The number is -123.6.
The number is 0.0.
The number is 0.01171875.
The number is inf.
In the given code, we are using the math.ldexp
function to calculate the result of x
times 2
to the power of i
. In the first instance, where x
is -30.9
and i
is 2
, the output is obtained by multiplying -30.9
by 2
to the power of 2
, resulting in a value of -123.6
.
In the second case, with x
as 0
and i
as 0
, the ldexp
function returns 0
, as any number multiplied by 2
to the power of 0
is itself. The third instance involves x
being 3
and i
being -8
, yielding a value of 0.01171875
, indicating the result of 3
multiplied by 2
to the power of -8
.
Lastly, when x
is set to positive infinity and i
is 1
, the output is also infinity, as any finite number multiplied by 2
to the power of 1
results in infinity. The print statements clearly display these calculated values.
Note that the values may be either positive or negative. These methods are used in mathematical computations related to geometry and have a specific application in astronomical calculations.
Example Code: Error While Using the math.ldexp()
Method
import math
x = "Hi"
i = 1
value = math.ldexp(x, i)
print(f"The number is {value}.")
x = [1, 2, 3]
i = 9
value = math.ldexp(x, i)
print(f"The number is {value}.")
x = 1 + 5j
i = 9 + 4j
value = math.ldexp(x, i)
print(f"The number is {value}.")
Output:
Traceback (most recent call last):
File "main.py", line 9, in <module>
value=math.frexp(x)
TypeError: must be a real number, not str
Traceback (most recent call last):
File "main.py", line 19, in <module>
value=math.frexp(x)
TypeError: must be a real number, not a list
Traceback (most recent call last):
File "main.py", line 28, in <module>
value=math.frexp(x)
TypeError: can't convert complex to float
In this code, we are attempting to use the math.ldexp
function, which is designed for numerical values with non-numeric data types. In the first case, when x
is a string "Hi"
and i
is 1
, the attempt to perform ldexp
results in a TypeError
, as strings are not compatible with this mathematical operation.
Similarly, in the second instance, where x
is a list [1, 2, 3]
, and i
is 9
, the code raises a TypeError
due to the list being an invalid operand for ldexp
. Lastly, in the third case, with x
as a complex number (1 + 5j
) and i
as a complex number (9 + 4j
), ldexp
encounters a TypeError
as complex numbers are not supported for this operation either.
Consequently, the code fails to execute properly in all three scenarios, highlighting the importance of using compatible data types with mathematical functions.
Note that the above-stated errors will be raised when the argument values are out of data range.
Example Code: Use Multiple Entries on the math.ldexp()
Method
import math
lists = [15, -13.76, 21]
i = [0, 1, 2]
print(f"The number is {math.ldexp(lists[0],i[0])}.")
print(f"The number is {math.ldexp(lists[1],i[1])}.")
print(f"The number is {math.ldexp(lists[2],i[2])}.")
Output:
The number is 15.0.
The number is -27.52.
The number is 84.0.
In this code, we are utilizing the math.ldexp
function to calculate the result of each element in the 'lists'
list multiplied by 2
raised to the power of the corresponding element in the 'i'
list. In the first line, lists
is defined as [15, -13.76, 21]
, and i
is set to [0, 1, 2]
.
Subsequently, we use three print statements to display the outcomes of the ldexp
operation for each pair of elements from lists
and i
. In the first print statement, the result is 15.0
, as 15
multiplied by 2
to the power of 0
is 15
.
In the second statement, the output is -27.52
, representing the result of -13.76
multiplied by 2
to the power of 1
. Lastly, in the third print statement, the value is 84.0
, corresponding to 21
multiplied by 2
to the power of 2
.
The code efficiently calculates and presents these results clearly and concisely. Note that you can find the value of any number using the mantissa and exponent method through the array notation.
Example Code: The math.ldexp()
Method and Its Inverse
import math
x = -30.9
value = math.frexp(x)
print(f"The mantissa and exponent of {x} are {value}.")
m = value[0]
e = value[1]
inverse = math.ldexp(m, e)
print(f"The inverse is {inverse}.")
Output:
The mantissa and exponent of -30.9 are (-0.965625, 5).
The inverse is -30.9.
In this code, we use the math.frexp
function to decompose the number -30.9
into its mantissa and exponent components. The frexp
function returns a tuple containing the mantissa and exponent, denoted as 'value'
in this case.
We then print the result, stating that the mantissa and exponent of -30.9
are represented by the tuple {value}
. In the subsequent lines, we extract the mantissa and exponent from the 'value'
tuple and use the math.ldexp
function to calculate the inverse of the original number.
The inverse is obtained by multiplying the mantissa and raising it to the power of the exponent. Finally, we print the inverse, indicating that it is the result of the mathematical operation.
The code effectively demonstrates the decomposition and reconstruction of a number using the frexp
and ldexp
functions, respectively. Note that math.ldexp()
is the inverse of the method math.frexp()
.
Conclusion
The math.ldexp()
method in Python serves as an efficient means of converting the mantissa and exponent of any number back to the original number using the formula x * (2**i)
. The method’s syntax involves two parameters, x
and i
, representing any positive or negative numbers.
The return type is a floating-point value representing the result of the specified calculation. The provided examples showcase the functionality of the method, demonstrating its versatility in handling various numerical scenarios, including cases where the input is a string, list, or complex number.
Additionally, the code exhibits error handling when attempting to use non-numeric data types with math.ldexp()
. The article concludes by illustrating the practical application of the method in decomposing and reconstructing a number using the math.frexp()
and math.ldexp()
functions, respectively.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn