Python math.hypot() Method
-
Syntax of
math.hypot()
Method in Python -
Example Code: Use
math.hypot()
With Two Input Values -
Example Code: Use
math.hypot()
With N-Dimensional Points -
Example Code: Use
math.hypot()
With Formulamath.sqrt((p*p)+(b*b))
-
Example Code: Find Time Complexity of the
math.hypot()
Method - Conclusion
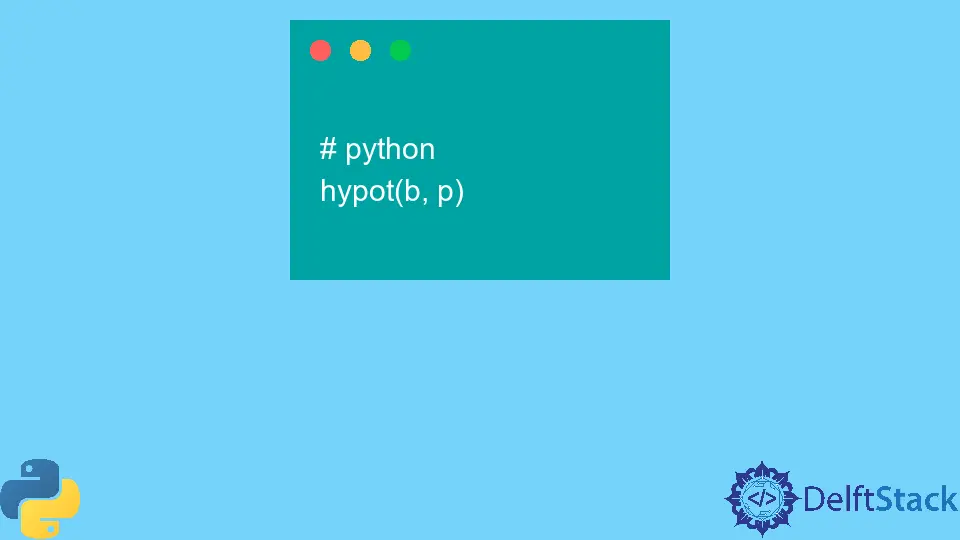
The Python math.hypot()
method is used to calculate the Euclidean norm of any input coordinate values. The Euclidian norm is the distance between the origin and the specified coordinates.
This method uses the formula sqrt(x*x + y*y)
to calculate the answer.
Syntax of math.hypot()
Method in Python
math.hypot(p, b)
Parameters
Parameter | Description |
---|---|
p |
A positive or negative number represents the perpendicular side of a right-angle triangle. |
b |
A positive or negative number represents the base side of a right-angle triangle. |
Returns
The math.hypot()
returns a floating point value representing the Euclidean norm, sqrt(x*x + y*y).
Example Code: Use math.hypot()
With Two Input Values
import math
p = 6
b = 4
value = math.hypot(p, b)
print(
f"The hypotenuse of a right-angle triangle having the perpendicular {p} and base {b} is {value}."
)
p = -10
b = -2
value = math.hypot(p, b)
print(
f"The hypotenuse of a right-angle triangle having the perpendicular {p} and base {b} is {value}."
)
p = 0
b = 0
value = math.hypot(p, b)
print(
f"The hypotenuse of a right-angle triangle having the perpendicular {p} and base {b} is {value}."
)
p = math.inf
b = math.inf
value = math.hypot(p, b)
print(
f"The hypotenuse of a right-angle triangle having the perpendicular {p} and base {b} is {value}."
)
This Python code uses the math.hypot
function to calculate the hypotenuse of right-angle triangles with different perpendicular (p
) and base (b
) values.
The first block computes the hypotenuse for a triangle with a perpendicular of 6
and a base of 4
. The second block calculates the hypotenuse for a triangle with negative values for both perpendicular (-10
) and base (-2
).
The third block computes the hypotenuse for a triangle with both perpendicular and base set to 0
. The fourth block demonstrates using math.inf
for both perpendicular and base, showcasing how the function handles infinite values.
Each block then prints the result in a formatted string, stating the hypotenuse for the given triangle parameters. The math.hypot
function calculates the Euclidean norm, providing the length of the hypotenuse based on the perpendicular and base lengths.
Output:
The hypotenuse of a right-angle triangle having the perpendicular 6 and base 4 is 7.211102550927979.
The hypotenuse of a right-angle triangle having the perpendicular -10 and base -2 is 10.19803902718557.
The hypotenuse of a right-angle triangle having the perpendicular 0 and base 0 is 0.0.
The hypotenuse of a right-angle triangle having the perpendicular inf and base inf is inf.
Example Code: Use math.hypot()
With N-Dimensional Points
Example Code:
import math
print(math.hypot(6, 9, 12))
print(math.hypot(9, 2, 11, 13))
print(math.hypot(10, 0, 3, 16, 23))
This Python code utilizes the math.hypot
function to calculate the hypotenuse for right-angle triangles with varying numbers of sides. The first line calculates the hypotenuse for a triangle with sides of lengths 6
, 9
, and 12
.
The second line computes the hypotenuse for a triangle with sides of lengths 9
, 2
, 11
, and 13
. The third line determines the hypotenuse for a triangle with sides of lengths 10
, 0
, 3
, 16
, and 23
.
Each line prints the resulting hypotenuse, demonstrating the flexibility of math.hypot
to handle multiple side lengths and provide the corresponding hypotenuse length for each set of triangle dimensions.
Output:
16.15549442140351
19.364916731037084
29.899832775452108
Note that newer versions of Python support multiple arguments to be entered in the math.hypot()
.
We can enter N-dimensional points, and the length of the vector from the point of origin to specified coordinates is returned.
Example Code: Use math.hypot()
With Formula math.sqrt((p*p)+(b*b))
Example Code:
import math
p = 6
b = 4
value = math.sqrt((p * p) + (b * b))
print(
f"The hypotenuse of a right-angle triangle having the perpendicular {p} and base {b} is {value}"
)
This Python code manually calculates the hypotenuse of a right-angle triangle using the Pythagorean theorem. It sets the perpendicular (p
) to 6
and the base (b
) to 4
. The formula for the hypotenuse is determined by the square root of the sum of the squares of the perpendicular and base lengths.
The code then prints a formatted string stating the hypotenuse for the given triangle parameters. This manual calculation mimics the behavior of the math.hypot
function and is useful for understanding the underlying mathematical concept.
Output:
The hypotenuse of a right-angle triangle having the perpendicular 6 and base 4 is 7.211102550927978
Note that the above-stated formula is the underlying working of the math.hypot()
method.
Example Code: Find Time Complexity of the math.hypot()
Method
Example Code:
import math
import math
import time
p = 3
b = 8
startHypot = time.time()
hypot = math.hypot(p, b)
print(
"The hypotenuse of the right-angle triangle using the formula is "
+ str(hypot)
+ "."
)
print(
"The time taken to compute the value using the formula is "
+ str(time.time() - startHypot)
+ "."
)
start = time.time()
hypo = math.sqrt((p * p) + (b * b))
print(
"The hypotenuse of the right-angle triangle using the equation is "
+ str(hypo)
+ "."
)
print(
"The time taken to compute the value using the equation is "
+ str(time.time() - start)
+ "."
)
This Python code compares the performance of two methods for calculating the hypotenuse of a right-angle triangle. It uses the math.hypot
function for one method and manually calculates the hypotenuse using the Pythagorean theorem for the other.
The code sets the perpendicular (p
) to 3
and the base (b
) to 8
. It measures the time taken to compute the hypotenuse using math.hypot
and prints the result along with the time taken for this method.
It then measures the time taken to compute the hypotenuse using the Pythagorean theorem manually and prints the result along with the time taken for this method. This code provides insights into the comparative efficiency of the math.hypot
function and the manual calculation approach in terms of computation time.
Output:
The hypotenuse of the right-angle triangle using the formula is 8.54400374531753.
The time taken to compute the value using the formula is 2.9325485229492188e-05.
The hypotenuse of the right-angle triangle using the equation is 8.54400374531753.
The time taken to compute the value using the equation is 5.4836273193359375e-05.
Conclusion
We’ve explored the math.hypot()
method in Python, which is designed to calculate the Euclidean norm or the distance from the origin to specified coordinates. The syntax, parameters, and return values of the method were explained, providing a clear understanding of its functionality.
The article presented examples demonstrating the use of math.hypot()
with two input values, N-dimensional points, and a comparison with the underlying formula math.sqrt((p*p) + (b*b))
. Additionally, we examined the time complexity of the math.hypot()
method compared to the manual calculation using the formula.
The examples showcased the versatility and efficiency of the math.hypot()
method in various scenarios, making it a valuable tool for mathematical computations involving Euclidean distances in Python.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn