Python math.gcd() Method
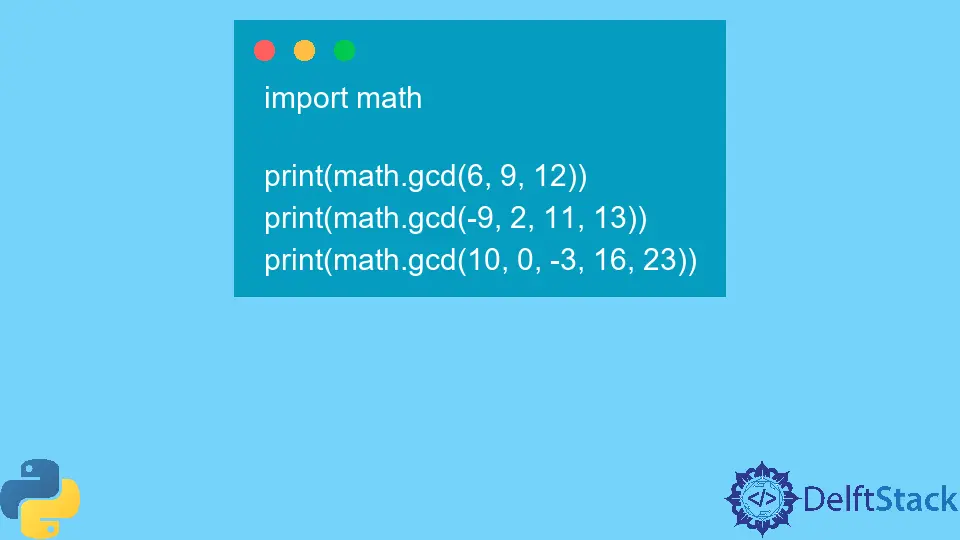
Python math.gcd()
method is an efficient way to get the greatest common divisor of specified numbers.
Note that the GCD is the largest common divisor, which divides the specified numbers without the remainder. Therefore, GCD is also addressed as the highest common factor.
Syntax
math.gcd(x, y)
Parameters
x |
A positive or negative number. |
y |
A positive or negative number. |
Returns
This method’s return type is an absolute/positive integer value representing the GCD of given parameters x
and y
.
Example Codes
Following are a few example codes demonstrating various inputs.
Use the math.gcd()
Method With One & Two Numbers
Example Code:
import math
x = 6
y = 4
value = math.gcd(x, y)
print(f"The GCD of {x} and {y} are {value}")
x = -200
y = -86
value = math.gcd(x, y)
print(f"The GCD of {x} and {y} is {value}")
x = 0
y = 0
value = math.gcd(x, y)
print(f"The GCD of {x} and {y} is {value}")
x = 3
value = math.gcd(x)
print(f"The GCD of {x} is {value}")
Output:
The GCD of 6 and 4 are 2
The GCD of -200 and -86 is 2
The GCD of 0 and 0 is 0
The GCD of 3 is 3
Note that it is necessary to enter the arguments in this method; otherwise, TypeError
occurs.
Use the math.gcd()
Method With n
Numbers
Example Code:
import math
print(math.gcd(6, 9, 12))
print(math.gcd(-9, 2, 11, 13))
print(math.gcd(10, 0, -3, 16, 23))
Output:
3
1
1
Note that newer versions of Python support multiple arguments to be entered in the math.gcd()
method. Here, TypeError
will occur if the installed Python version doesn’t support more than two arguments for this method.
Demonstrate Errors While Using the math.gcd()
Method
Example Code:
import math
x = 6.98
y = 4.0
value = math.gcd(x, y)
print(f"The GCD of {x} and {y} is {value}")
x = math.inf
y = 4.0
value = math.gcd(x, y)
print(f"The GCD of {x} and {y} is {value}")
x = "a"
y = "b"
value = math.gcd(x, y)
print(f"The GCD of {x} and {y} is {value}")
Output:
Traceback (most recent call last):
File "main.py", line 7, in <module>
value= math.gcd(x,y)
TypeError: 'float' object cannot be interpreted as an integer
Traceback (most recent call last):
File "main.py", line 15, in <module>
value= math.gcd(x,y)
TypeError: 'float' object cannot be interpreted as an integer
Traceback (most recent call last):
File "main.py", line 23, in <module>
value= math.gcd(x,y)
TypeError: 'str' object cannot be interpreted as an integer
Note that the above-stated errors can occur when you use the math.gcd()
method. For example, TypeError
might arise if the Python version in your system doesn’t support the argument size.
Use the math.gcd()
Method With User’s Input
Example Code:
import math
x = int(input("Enter the first number: "))
y = int(input("Enter the second number: "))
value = math.gcd(x, y)
print(f"The greatest common divisor of {x} and {y} is {value}")
Output:
Enter the first number: 650
Enter the second number: 90
The greatest common divisor of 650 and 90 is 10
The above code is dynamic and allows users to find the GCD of any number they want.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn