Python math.gamma() Method
-
Syntax of
math.gamma()
in Python -
Example Code: Use of the
math.gamma()
Method -
Example Code: Demonstration of the
math.gamma()
Method’s Formula -
Example Code: Demonstrate
TypeError
&ValueError
Using themath.gamma()
Method -
Example Code: Find Time Complexity of the
math.gamma()
Method - Conclusion
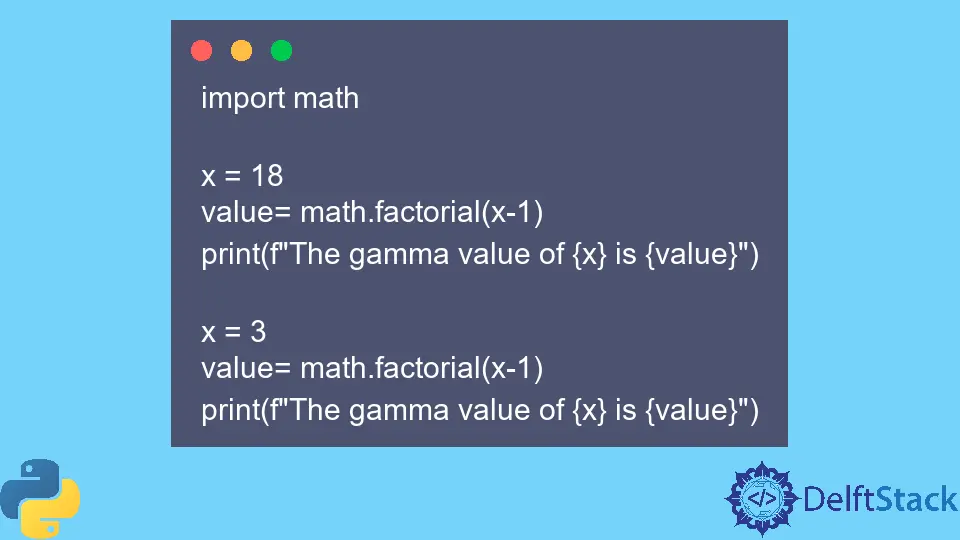
The Python math.gamma()
method numerically computes a gamma
value of the number that is passed in the function.
Syntax of math.gamma()
in Python
math.gamma(x)
Parameters
Parameter | Description |
---|---|
x |
A positive number whose gamma value is needed. |
Returns
This method’s return value is a floating point value representing the gamma
value of a number, which is equal to the factorial(x-1)
.
The following are a few code snippets to learn the use of the math.gamma()
method.
Example Code: Use of the math.gamma()
Method
import math
x = 65
value = math.gamma(x)
print(f"The gamma value of {x} is {value}")
x = 3
value = math.gamma(x)
print(f"The gamma value of {x} is {value}")
x = math.inf
value = math.gamma(x)
print(f"The gamma value of {x} is {value}")
Output:
The gamma value of 65 is 1.2688693218588412e+89
The gamma value of 3 is 2.0
The gamma value of inf is inf
In this code, we utilize Python’s math
module to calculate the gamma values for three different inputs. Firstly, we set x
to 65
and compute its gamma value using math.gamma(x)
, storing the result in the variable value
.
We then print the outcome, revealing that the gamma value for 65
is approximately 1.2688693218588412e+89
. Next, we repeat the process for x = 3
, displaying that the gamma value for 3
is 2.0
.
Lastly, we set x
to positive infinity (math.inf
) and find its gamma value, resulting in an output of infinity. This code demonstrates the versatility of the math.gamma()
method in handling different numeric inputs and computing their respective gamma values.
Note that the values entered in the function must be positive only. If the number entered is a negative integer, it will return a ValueError.
If the entered parameter is not a number, it produces a TypeError.
Example Code: Demonstration of the math.gamma()
Method’s Formula
import math
x = 18
value = math.factorial(x - 1)
print(f"The gamma value of {x} is {value}")
x = 3
value = math.factorial(x - 1)
print(f"The gamma value of {x} is {value}")
Output:
The gamma value of 18 is 355687428096000
The gamma value of 3 is 2
In this code, we set x
to 18
and use math.factorial(x - 1)
to compute its gamma value, storing the result in the variable value
. Subsequently, we print the outcome, revealing that the gamma value for 18
is 355687428096000
.
We then repeat the process for x = 3
, displaying that the gamma value for 3
is 2
. This code illustrates the application of the math.factorial()
function in numerically computing gamma values for different input values.
Note that math.gamma()
numerically computes the gamma
value factorial(x-1)
of the given x
. We have used factorial(x-1)
instead, which returns the value as an integer.
Example Code: Demonstrate TypeError
& ValueError
Using the math.gamma()
Method
import math
x = -2
print("The gamma value is ", math.gamma(x))
x = "h"
print("The gamma value is ", math.gamma(x))
Output:
Traceback (most recent call last):
File "/home/main.py", line 4, in <module>
print("The gamma value is ", math.gamma(x))
ValueError: math domain error
In this code, we set x
to -2
and attempt to calculate its gamma value using math.gamma(x)
. However, this results in a ValueError
since the method requires positive numeric input.
Subsequently, we set x
to the string "h"
and once again try to find its gamma value using math.gamma(x)
. In this case, a TypeError
occurs, as the method expects a real number, not a string.
This code demonstrates the potential errors that may arise when incorrect parameters are provided to the math.gamma()
method. Note that the following errors might occur when entering the wrong parameters.
Example Code: Find Time Complexity of the math.gamma()
Method
import math
import time
x = 3
startFact = time.time()
fact = math.factorial(x - 1)
print("The gamma value calculated using factorial is " + str(fact))
print(
"The time taken to compute the value using the factorial is "
+ str(time.time() - startFact)
)
start = time.time()
gamma = math.gamma(x)
print("The gamma value calculated using the gamma() function is " + str(gamma))
print(
"The time taken to compute the value using the function is "
+ str(time.time() - start)
)
Output:
The gamma value calculated using factorial is 2
The time taken to compute the value using the factorial is 0.0009968280792236328
The gamma value calculated using the gamma() function is 2.0
The time taken to compute the value using the function is 0.0
In this code, we employ the math
and time
modules in Python to compare the efficiency of computing gamma values using the math.factorial()
function and the math.gamma()
method. We set x
to 3
and measure the time taken to calculate the gamma value using math.factorial(x - 1)
.
The result is then printed along with the corresponding computation time. Subsequently, we repeat the process for the math.gamma()
method, calculating the gamma value for the same input.
The output provides a comparison of the two methods, indicating that the math.gamma()
function is more efficient, as reflected in the shorter computation time.
This code illustrates the time complexity difference between the two approaches for computing gamma values in Python.
Conclusion
Python’s math.gamma()
method efficiently computes the gamma value of a positive number, equivalent to factorial(x-1)
. The method is demonstrated through code snippets, showcasing its application and highlighting its speed compared to traditional factorial calculations.
Inputting negative integers raises a ValueError
, and non-numeric parameters result in a TypeError
. Overall, math.gamma()
is a valuable tool for precise and swift gamma function calculations in Python.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn