Python math.fsum() Method
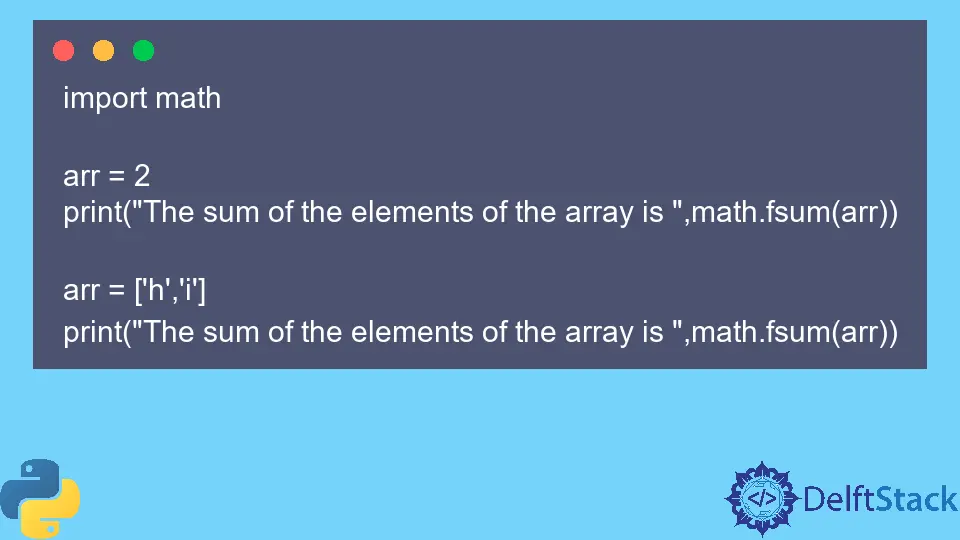
Python math.fsum()
method is used to find the sum between a specified range or an iterable. This method avoids loss of precision by constantly tracking multiple intermediate partial sums of the numbers entered.
Syntax
math.fsum(i)
Parameters
i |
A list array or tuple to sum. The iterable can have either positive or negative numbers. |
Returns
The math.fsum()
returns a floating point value representing the sum of all values of the given iterable.
Example Codes
Following are a few example codes demonstrating the use of the math.fsum()
method.
Use of the math.fsum()
Method
Example Code:
import math
arr = [1, 42, 65]
print("The sum is", math.fsum(arr))
arr = [2.5, 2.4, 3.09]
print("The sum is", math.fsum(arr))
arr = [-3, 4, 0, -9]
print("The sum is", math.fsum(arr))
arr = [math.inf]
print("The sum is", math.fsum(arr))
arr = []
print("The sum is", math.fsum(arr))
Output:
The sum is 108.0
The sum is 7.99
The sum is -8.0
The sum is inf
The sum is 0.0
Note that the values entered in the array may be either positive or negative.
Use the math.fsum()
Method With User’s Input Data
Example Code:
import math
print("Enter the elements of the array and separate the numbers with a space: ")
arr = list(map(int, input().split(" ")))
print("you entered the following elements:", arr)
print("The sum of the elements in your array is:", math.fsum(arr))
Output:
Enter the elements of the array and separate the numbers with a space:
1 2 3 4
you entered the following elements: [1, 2, 3, 4]
The sum of the elements in your array is: 10.0
Note that you can also enter a floating point number in the math.fsum()
method.
Demonstrate TypeError
Using the math.fsum()
Method
Example Code:
import math
arr = 2
print("The sum of the elements of the array is ", math.fsum(arr))
arr = ["h", "i"]
print("The sum of the elements of the array is ", math.fsum(arr))
Output:
Traceback (most recent call last):
File "main.py", line 5, in <module>
print("The sum of the elements of the array is ",math.fsum(arr))
TypeError: 'int' object is not iterable
Traceback (most recent call last):
File "main.py", line 9, in <module>
print("The sum of the elements of the array is ",math.fsum(arr))
TypeError: must be a real number, not str
Note that the parameter of this method must be iterable.
Specify the Range in the math.fsum()
Method
Example Code:
import math
value = 10
sumVal = math.fsum(range(value))
print(f"The sum of all the numbers from 0 to {value} is {sumVal}.")
Output:
The sum of all the numbers from 0 to 10 is 45.0.
In the above code, we have used the range()
function. The function processes a sequence of numbers, starting from 0
and incrementing the value by 1
, and stopping before a specified number.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn