Python math.frexp() Method
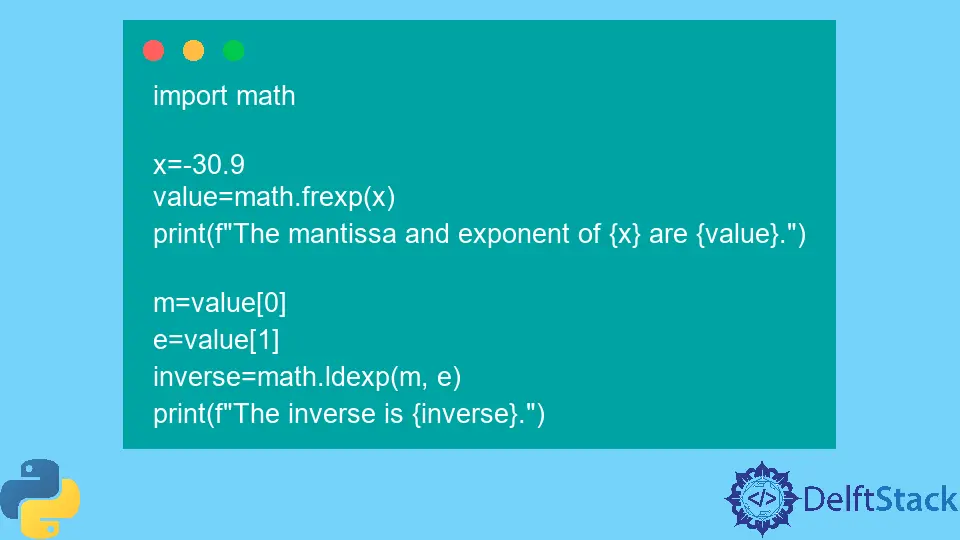
Python math.frexp()
method is used to calculate the mantissa and exponent of any number.
These components are represented as a pair (m
, e
) where m
is mantissa, and e
is the exponent. The background mathematical formula for this method is number = m * 2**e.
Syntax
math.frexp(x)
Parameters
x |
Any number, either positive or negative. |
Returns
The return type of the math.frexp()
method is a pair (m
, e
) value representing mantissa and exponent of a given number x.
Note that mantissa m
is a floating point number, and exponent e
is an integer value.
Example Codes
Using various code snippets below, let’s learn the math.frexp()
method.
Use math.frexp()
With Single Input
Example Code:
import math
x = -30.9
value = math.frexp(x)
print(f"The mantissa and exponent of {x} are {value}.")
x = 0
value = math.frexp(x)
print(f"The mantissa and exponent of {x} are {value}.")
x = 3
value = math.frexp(x)
print(f"The mantissa and exponent of {x} are {value}.")
x = math.inf
value = math.frexp(x)
print(f"The mantissa and exponent of {x} are {value}.")
Output:
The mantissa and exponent of -30.9 are (-0.965625, 5).
The mantissa and exponent of 0 are (0.0, 0).
The mantissa and exponent of 3 are (0.75, 2).
The mantissa and exponent of inf are (inf, 0).
Remember that the values may be either negative or positive. These are used in mathematical computations related to geometry and have a specific application in astronomical calculations.
Demonstrate TypeError
Using the math.frexp()
Method
Example Code:
import math
# entering a string
x = "Hi"
value = math.frexp(x)
print(f"The mantissa and exponent of {x} are {value}.")
# entering a list
x = [1, 2, 3]
value = math.frexp(x)
print(f"The mantissa and exponent of {x} are {value}.")
# entering complex numbers
x = 1 + 5j
value = math.frexp(x)
print(f"The mantissa and exponent of {x} are {value}.")
Output:
Traceback (most recent call last):
File "main.py", line 7, in <module>
value=math.frexp(x)
TypeError: must be a real number, not str
Traceback (most recent call last):
File "main.py", line 15, in <module>
value=math.frexp(x)
TypeError: must be a real number, not a list
Traceback (most recent call last):
File "main.py", line 23, in <module>
value=math.frexp(x)
TypeError: can't convert complex to float
Note that if x
is 0
, then it returns (0.0, 0)
, otherwise 0.5 <= abs(m) < 1
is returned. We can use this result to pick apart the internal representation of a float number in a portable way.
Use math.frexp()
With Multiple Entries
Example Code:
import math
lists = [15, -13.76, 21]
print(f"The mantissa and exponent of {lists[0]} are {math.frexp(lists[0])}.")
print(f"The mantissa and exponent of {lists[1]} are {math.frexp(lists[1])}.")
print(f"The mantissa and exponent of {lists[2]} are {math.frexp(lists[2])}.")
Output:
The mantissa and exponent of 15 are (0.9375, 4).
The mantissa and exponent of -13.76 are (-0.86, 4).
The mantissa and exponent of 21 are (0.65625, 5).
Note that you can find the exponent and mantissa of a list by using the array notation.
the math.frexp()
Method and Its Inverse
Example Code:
import math
x = -30.9
value = math.frexp(x)
print(f"The mantissa and exponent of {x} are {value}.")
m = value[0]
e = value[1]
inverse = math.ldexp(m, e)
print(f"The inverse is {inverse}.")
Output:
The mantissa and exponent of -30.9 are (-0.965625, 5).
The inverse is -30.9.
Note that math.ldexp()
is the inverse of the method math.frexp()
.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn