Python Math.fmod() Method
-
Syntax of Python
math.fmod()
Method -
Example Code: Use of the
math.fmod()
Method in Python -
Example Code: Cause of the
ValueError: math domain error
When Using themath.fmod()
Method -
Example Code: Cause of the
TypeError
When Using themath.fmod()
Method -
Example Code: Difference Between the
%
Operator and themath.fmod()
Method
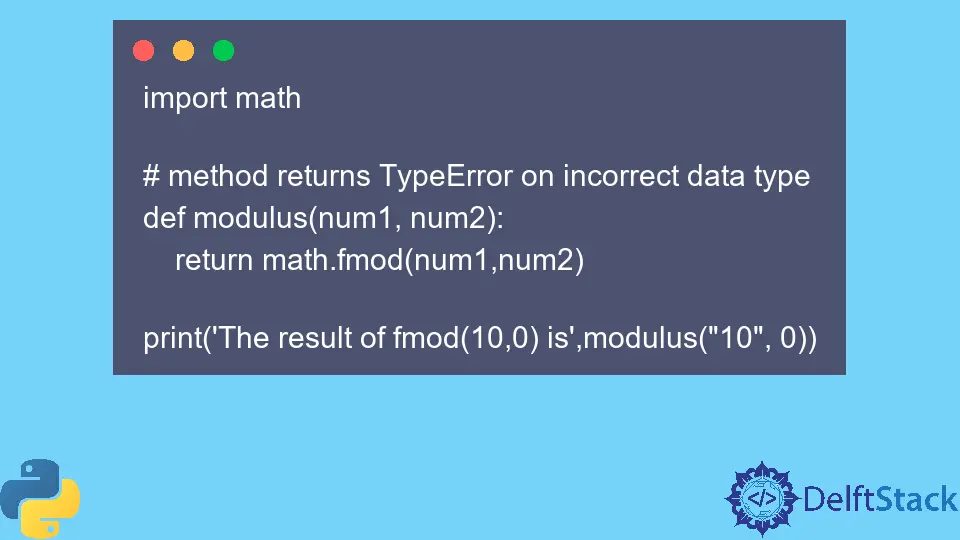
In Python, the math
module provides the fmod()
method to find the remainder of two numbers- the numerator and denominator. These numbers can be positive/negative integers or float.
The math.fmod()
method was introduced in Python version 1.6 and is part of theoretical and representation functions in Python documentation.
The operator %
is similar to the math.fmod()
method, which returns different results when one of the numbers is non-positive. Programmers are encouraged to use the math.fmod()
method when finding the modulus in float numbers.
Syntax of Python math.fmod()
Method
math.fmod(number1, number2)
Parameters
number1 |
This is a required parameter. It can be an integer or float and act as a numerator in the division. |
number2 |
This is a required parameter. It can be an integer or float and act as a denominator in the division. |
Return
This method returns the float value as a remainder after dividing the two numbers.
Example Code: Use of the math.fmod()
Method in Python
To use the method math.fmod()
, we pass two required parameters: numerator and denominator. These parameters output a remainder in the form of a float value.
These parameters can be positive or negative and even can be integer or float numbers. Remember, these parameters cannot hold zero simultaneously, or the ValueError
exception is returned.
The ValueError
exception can also occur if the denominator is zero.
# import math module to use the math.fmod() method
import math
# positive and negative numbers are used as a parameter to test the behavior of the method
print("The result of fmod(11,6) is", math.fmod(11, 6))
print("The result of fmod(-20,4) is", math.fmod(-20, 4))
print("The result of fmod(7,-2) is", math.fmod(7, -2))
print("The result of fmod(0,6) is", math.fmod(0, 6))
print("The result of fmod(22.1,3.4) is", math.fmod(22.1, 3.4))
print("The result of fmod(22.1,3.4) is", math.fmod(14, 7.1))
Output:
The result of fmod(11,6) is 5.0
The result of fmod(-20,4) is -0.0
The result of fmod(7,-2) is 1.0
# output always zero, if the numerator is zero
The result of fmod(0,6) is 0.0
The result of fmod(22.1,3.4) is 1.700000000000002
The result of fmod(22.1,3.4) is 6.9
Example Code: Cause of the ValueError: math domain error
When Using the math.fmod()
Method
In Python, we use the method math.fmod()
to find the remainder of two numbers. When we pass zero as the denominator value, the method math.fmod()
returns the ValueError
exception.
Note that we cannot pass zero as the second parameter to this method, or the method will throw an exception.
import math
# modulus method returns the ValueError if there is zero in denominator
def modulus(num1, num2):
return math.fmod(num1, num2)
print("The result of fmod(10,0) is", modulus(10, 0))
Output:
# exception as output, when number zero is passed to the second parameter.
ValueError: math domain error
Example Code: Cause of the TypeError
When Using the math.fmod()
Method
As we know, the math.fmod()
method finds the remainder of the number, so the two required parameters cannot accept data types other than the number. When we pass a string (which is not a number), the method math.fmod()
returns the TypeError
exception.
To avoid such a situation, we must carefully select and pass the correct parameters (a number) to the method.
import math
# method returns TypeError on incorrect data type
def modulus(num1, num2):
return math.fmod(num1, num2)
print("The result of fmod(10,0) is", modulus("10", 0))
Output:
# returns this exception when one of the parameters is a string.
TypeError: must be real number, not str
Example Code: Difference Between the %
Operator and the math.fmod()
Method
In Python, a similar operator calculates the remainder of the number, known as the %
modulus operator. This is a built-in operator in Python and is not a function.
We take two positive integer numbers to show the similarity between the %
modulus operator and the math.fmod()
method. The result of the two operations shows the same results.
However, we can see the difference between the operations when we pass one positive integer with the negative integer. Further, the difference can be seen when we give zero in the denominator.
The math.fmod()
method always returns zero when there is a number with zero value in the denominator, unlike the %
modulus, which shows ValueError
. It cannot solve the zero value in the denominator.
We use the math.fmod()
method with float numbers and the %
modulus operator when working with integer values.
import math
# method returns output for fmod() method only
def modulus(num1, num2):
return math.fmod(num1, num2)
print("The result of fmod(10,0) is ", modulus(10, 3))
print("The result of 10 % 0 is ", 10 % 3)
print("The result of fmod(-11,6) is ", modulus(-11, 6))
print("The result of -11 % 6 is ", -11 % 6)
print("The result of fmod(-19,-5) is ", modulus(-19, -5))
print("The result of -19 % -5 is ", -19 % -5)
print("The result of fmod(0,9) is ", modulus(0, 9))
print("The result of 0 % 9 is ", 0 % 9)
# number zero cannot be used in the denominator for the % modulus operator
print("The result of fmod(4,0) is ", modulus(4, 0))
# prints the ValueError exception on the screen
print("The result of 4 % 0 is ", 4 % 0)
Output:
The result of fmod(10,0) is 1.0
The result of 10 % 0 is 1
# difference shows when there is a non-positive number involved in the division
The result of fmod(-11,6) is -5.0
The result of -11 % 6 is 1
The result of fmod(-19,-5) is -4.0
The result of -19 % -5 is -4
The result of fmod(0,9) is 0.0
The result of 0 % 9 is 0
Traceback (most recent call last):
File "C:\Users\lenovo\.spyder-py3\temp.py", line 18, in <module>
print('The result of fmod(4,0) is ',modulus(4, 0))
File "C:\Users\lenovo\.spyder-py3\temp.py", line 4, in modulus
return math.fmod(num1,num2)
ValueError: math domain error