Python math.degrees() Method
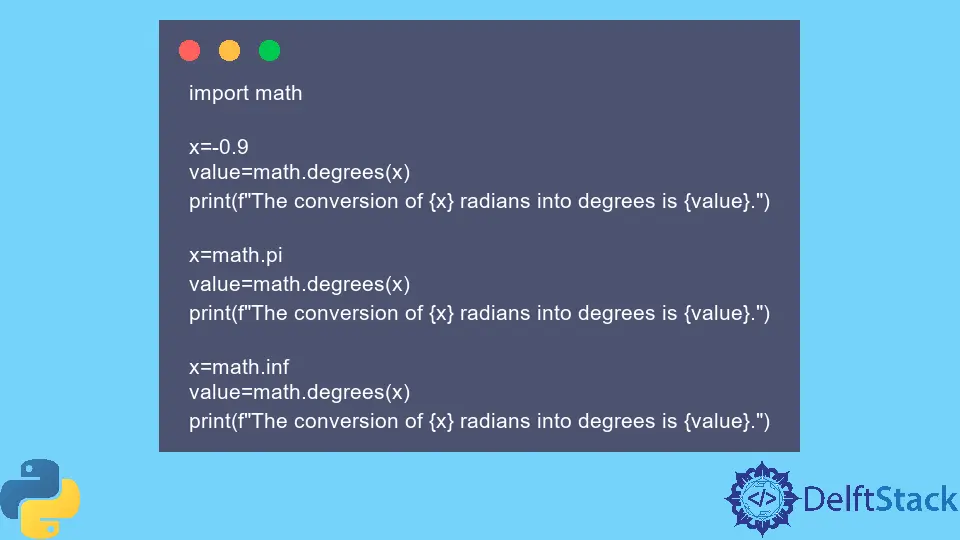
Python math.degrees()
method is an efficient way of converting the angle from radians to degrees. Note that 1 degree = pi/180 radians
.
Syntax
math.degrees(rad)
Parameters
rad |
Any angle in radians to convert into degrees. |
Returns
The return type for this method is an angle in degrees.
Examples
Correct Use of Python math.degrees()
Method
Code Snippet:
import math
x = -0.9
value = math.degrees(x)
print(f"The conversion of {x} radians into degrees is {value}.")
x = math.pi
value = math.degrees(x)
print(f"The conversion of {x} radians into degrees is {value}.")
x = math.inf
value = math.degrees(x)
print(f"The conversion of {x} radians into degrees is {value}.")
Output:
The conversion of -0.9 radians into degrees is -51.56620156177409.
The conversion of 3.141592653589793 radians into degrees is 180.0.
The conversion of inf radians into degrees is inf.
Note that the values may be either positive or negative. These methods are used in mathematical computations related to geometry and have a specific application in astronomical calculations.
TypeError
While Using Python math.degrees()
Method
Code Snippet:
import math
# entering a string
x = "Hi"
value = math.degrees(x)
print(f"The conversion of {x} radians into degrees is {value}.")
# entering a list
x = [1, 2, 3]
value = math.degrees(x)
print(f"The conversion of {x} radians into degrees is {value}.")
# entering complex numbers
x = 1 + 5j
value = math.degrees(x)
print(f"The conversion of {x} radians into degrees is {value}.")
Output:
Traceback (most recent call last):
File "main.py", line 7, in <module>
value=math.degrees(x)
TypeError: must be a real number, not str
Traceback (most recent call last):
File "main.py", line 8, in <module>
value=math.degrees(x)
TypeError: must be a real number, not a list
Traceback (most recent call last):
File "main.py", line 7, in <module>
value=math.degrees(x)
TypeError: can't convert complex to float
The above code snippets show all possible syntactic errors that might occur using the math.degrees
method.
Demonstrate the math.degrees()
Method and Its Inverse
Code Snippet:
import math
x = 7
value = math.degrees(x)
print(f"The conversion of {x} radians into degrees is {value}.")
rad = math.radians(value)
print(f"The conversion of {value} degrees into radians is {rad}.")
Output:
The conversion of 7 radians into degrees is 401.07045659157626.
The conversion of 401.07045659157626 degrees into radians is 7.0.
The angle can be either an integer (int
) or a floating point value (float
).
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn