Python math.asin() Method
-
Syntax of Python
math.asin()
Method -
Example Codes: Use
math.asin()
to Compute Arcsine of a Value -
Example Codes: Use
math.asin()
to Compute Arcsine and Cosine - Conclusion
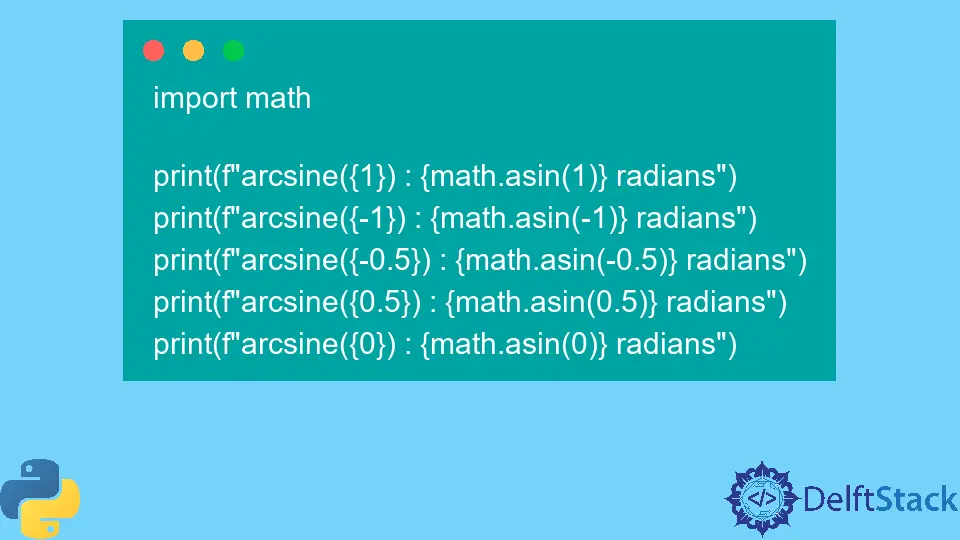
Python has an in-built library math module that contains utilities to perform many mathematical operations such as approximation, estimation, trigonometric operations, inverse trigonometric operations, lowest common multiple, greatest common divisor, factorial, permutation, etc.
The inverse of sine is known as arcsine or sin⁻¹θ
. Theoretically, the sine of an angle θ
is the ratio of its opposite side and the hypotenuse, and the domain and range of arcsine are [-1, 1]
and [−π/2, π/2]
, respectively.
sin(θ) = (opposite side) / (hypotenuse)
arcsine(θ) = sin⁻¹((opposite side) / (hypotenuse))
This article will discuss the asin()
method from the math module that helps calculate arcsine for angles.
Syntax of Python math.asin()
Method
math.asin(x)
Parameters
Parameters | Type | Explanation |
---|---|---|
x |
Integer | A value between -1 and 1 , both inclusive. |
Returns
The asin()
method returns the inverse of the sine or arcsine of x
in radians. The result is between the range −π/2
and π/2
, both inclusive.
Example Codes: Use math.asin()
to Compute Arcsine of a Value
The math.asin()
function in Python is a valuable tool for computing the arcsine (inverse sine) of a specified value. When using this function, one provides a numeric input, typically representing a sine value.
import math
print(f"arcsine({1}) : {math.asin(1)} radians")
print(f"arcsine({-1}) : {math.asin(-1)} radians")
print(f"arcsine({-0.5}) : {math.asin(-0.5)} radians")
print(f"arcsine({0.5}) : {math.asin(0.5)} radians")
print(f"arcsine({0}) : {math.asin(0)} radians")
Output:
arcsine(1) : 1.5707963267948966 radians
arcsine(-1) : -1.5707963267948966 radians
arcsine(-0.5) : -0.5235987755982989 radians
arcsine(0.5) : 0.5235987755982989 radians
arcsine(0) : 0.0 radians
In this code, we use the math.asin()
function to compute the arcsine of various values.
The first line calculates the arcsine of 1
, and we print the result. Since the arcsine of 1
corresponds to π/2
radians, the output is arcsine(1) : 1.5707963267948966 radians
.
The second line computes the arcsine of -1
, resulting in -1.5707963267948966 radians
as the output, reflecting the fact that the arcsine of -1
is -π/2
. Similarly, the third and fourth lines calculate the arcsine of -0.5
and 0.5
, respectively.
These outputs, -0.5235987755982989 radians
and 0.5235987755982989 radians
, represent angles in the range [-π/2, π/2]
corresponding to the arcsine values of -0.5
and 0.5
.
Finally, the last line computes the arcsine of 0
, yielding 0.0 radians
as expected since the arcsine of 0
is 0
. These results demonstrate how the math.asin()
function efficiently computes arcsine values for different inputs.
Example Codes: Use math.asin()
to Compute Arcsine and Cosine
We can utilize the math.asin()
in conjunction with other trigonometric functions, such as math.cos()
, which facilitates the calculation of additional trigonometric relationships.
Code Example 1:
import math
x = -1
asin = math.asin(x)
print(f"arcsine({x}):", asin)
print(f"sin({asin}):", math.sin(asin))
x = 0
asin = math.asin(x)
print(f"arcsine({x}):", asin)
print(f"sin({asin}):", math.sin(asin))
x = 1
asin = math.asin(x)
print(f"arcsine({x}):", asin)
print(f"sin({asin}):", math.sin(asin))
Output:
arcsine(-1): -1.5707963267948966
sin(-1.5707963267948966): -1.0
arcsine(0): 0.0
sin(0.0): 0.0
arcsine(1): 1.5707963267948966
sin(1.5707963267948966): 1.0
In the first code example, we set x
to -1
and compute its arcsine, storing the result in the variable asin
. We then print the arcsine and the sine of the arcsine, resulting in arcsine(-1): -1.5707963267948966
and sin(-1.5707963267948966): -1.0
, respectively.
Next, we repeat this process for x
equal to 0
, yielding arcsine(0): 0.0
and sin(0.0): 0.0
. Finally, when x
is set to 1
, we obtain arcsine(1): 1.5707963267948966
and sin(1.5707963267948966): 1.0
.
Here, in the next code example, we compute arcsine and sine for a set of values.
Code Example 2:
import math
values = [0.2, -0.8, 0.9, -0.3, 0.5]
for value in values:
arcsine_value = math.asin(value)
sine_of_arcsine = math.sin(arcsine_value)
print(f"arcsine({value}): {arcsine_value} radians")
print(f"sin(arcsine({value})): {sine_of_arcsine}\n")