Pandas DataFrame DataFrame.transform() Function
-
Syntax of
pandas.DataFrame.transform()
-
Example Codes:
DataFrame.transform()
-
Example Codes:
DataFrame.transform()
to Usesqrt
String as Function -
Example Codes:
DataFrame.transform()
to Pass the List of Functions -
DataFrame.apply()
vsDataFrame.transform()
Function
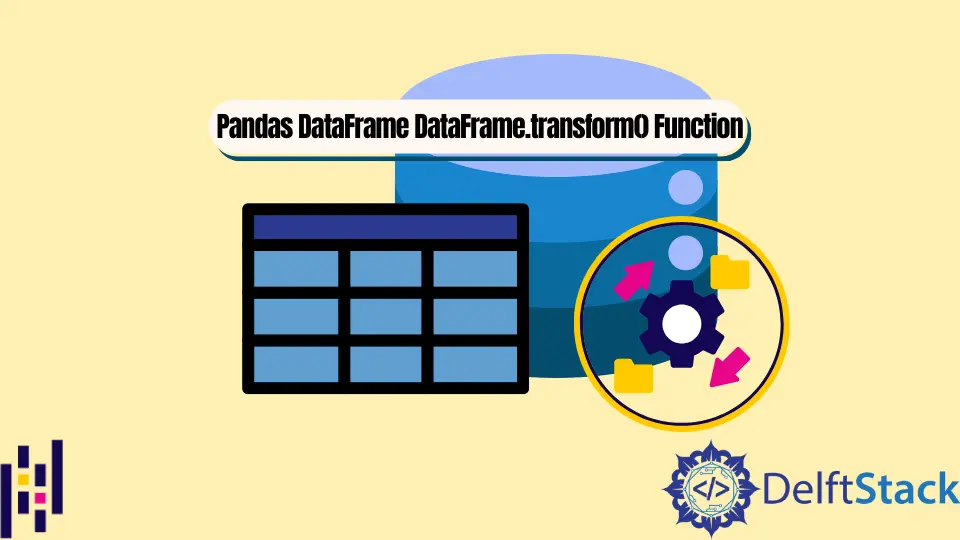
Python Pandas DataFrame.transform()
applies a function on a DataFrame
and transforms the DataFrame
. The function to be applied is passed as a parameter to the transform()
function. The transformed DataFrame
should have the same length of the axis as the original DataFrame
.
Syntax of pandas.DataFrame.transform()
DataFrame.transform(func, axis, *args, **kwargs)
Parameters
func |
It is the function to be applied to the DataFrame . It brings a change in the values of the DataFrame . It can be a function, function name string, list of functions or function names, or dictionary of axis labels. |
axis |
It is an integer or a string. It specifies the target axis either rows or columns. It can be 0 or index for rows and 1 or columns for columns. |
*args |
These are the positional arguments to pass to the function. |
**kwargs |
These are the additional keyword arguments to pass to the function. |
Return
It returns a transformed DataFrame
that has the same length as the original DataFrame
. If the returned DataFrame
has an unequal length then the function raises a ValueError
.
Example Codes: DataFrame.transform()
Let’s first try this function by adding a number to each value of the DataFrame
.
import pandas as pd
dataframe = pd.DataFrame({
'A':
{0: 6,
1: 20,
2: 80,
3: 78,
4: 95},
'B':
{0: 60,
1: 50,
2: 7,
3: 67,
4: 54}
})
print(dataframe)
The example DataFrame
is,
A B
0 6 60
1 20 50
2 80 7
3 78 67
4 95 54
5 98 34
This function has only one mandatory parameter i.e func
. Now we will use this function to add 20 to each value of the DataFrame
.
import pandas as pd
dataframe = pd.DataFrame(
{"A": {0: 6, 1: 20, 2: 80, 3: 78, 4: 95}, "B": {0: 60, 1: 50, 2: 7, 3: 67, 4: 54}}
)
dataframe1 = dataframe.transform(func=lambda x: x + 20)
print(dataframe1)
Output:
A B
0 26 80
1 40 70
2 100 27
3 98 87
4 115 74
5 118 54
The lambda
keyword is used to declare an anonymous function of addition here.
Example Codes: DataFrame.transform()
to Use sqrt
String as Function
import pandas as pd
dataframe = pd.DataFrame(
{"A": {0: 6, 1: 20, 2: 80, 3: 78, 4: 95}, "B": {0: 60, 1: 50, 2: 7, 3: 67, 4: 54}}
)
dataframe1 = dataframe.transform(func="sqrt")
print(dataframe1)
Output:
A B
0 2.449490 7.745967
1 4.472136 7.071068
2 8.944272 2.645751
3 8.831761 8.185353
4 9.746794 7.348469
5 9.899495 5.830952
Here, instead of passing a lambda
function, we have passed the function name as a string.
Example Codes: DataFrame.transform()
to Pass the List of Functions
import pandas as pd
dataframe = pd.DataFrame(
{"A": {0: 6, 1: 20, 2: 80, 3: 78, 4: 95}, "B": {0: 60, 1: 50, 2: 7, 3: 67, 4: 54}}
)
dataframe1 = dataframe.transform(func=["sqrt", "exp"])
print(dataframe1)
Output:
A B
sqrt exp sqrt exp
0 2.449490 4.034288e+02 7.745967 1.142007e+26
1 4.472136 4.851652e+08 7.071068 5.184706e+21
2 8.944272 5.540622e+34 2.645751 1.096633e+03
3 8.831761 7.498417e+33 8.185353 1.252363e+29
4 9.746794 1.811239e+41 7.348469 2.830753e+23
We have passed a list of two function names i.e ['sqrt', 'exp']
as the func
. The returned DataFrame
contains two extra columns due to an extra function.
DataFrame.apply()
vs DataFrame.transform()
Function
We can also achieve the above results using DataFrame.apply()
function. But if we compare these two functions, we will say that the DataFrame.transform()
function is more efficient to handle the complex manipulations.