Pandas DataFrame DataFrame.max() Function
-
Syntax of
pandas.DataFrame.max()
: -
Example Codes:
DataFrame.max()
Method to Find Max Along Column Axis -
Example Codes:
DataFrame.max()
Method to Find Max Along Row Axis -
Example Codes:
DataFrame.max()
Method to Get Max IgnoringNaN
Values
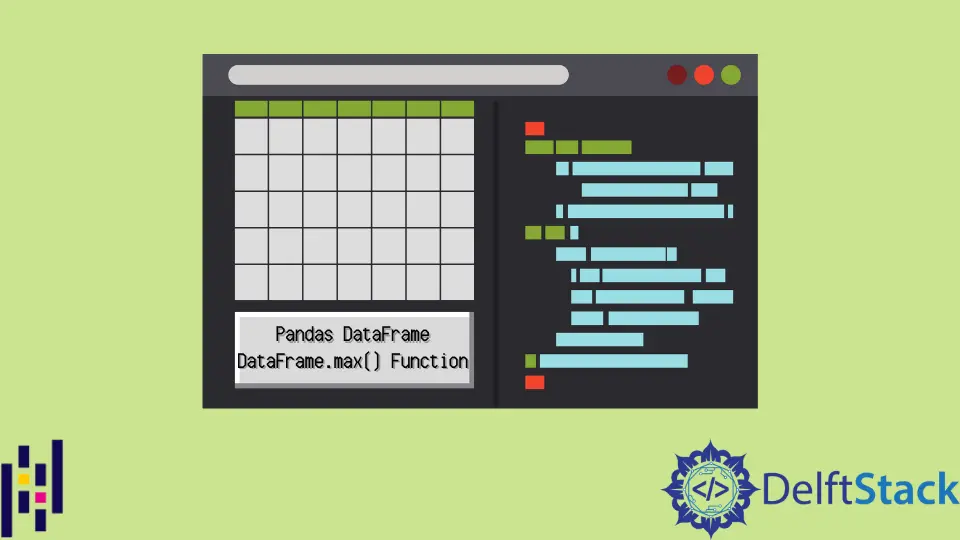
Python Pandas DataFrame.max()
function calculates max value of values of DataFrame object over the specified axis.
Syntax of pandas.DataFrame.max()
:
DataFrame.max(axis=None, skipna=None, level=None, numeric_only=None, **kwargs)
Parameters
axis |
find max along the row (axis=0) or column (axis=1) |
skipna |
Boolean. Exclude NaN values (skipna=True ) or include NaN values (skipna=False ) |
level |
Count along with particular level if the axis is MultiIndex |
numeric_only |
Boolean. For numeric_only=True , include only float , int and boolean columns |
**kwargs |
Additional keyword arguments to the function. |
Return
If the level
is not specified, return Series
of the maximum of the values for the requested axis, else return DataFrame
of max values.
Example Codes: DataFrame.max()
Method to Find Max Along Column Axis
import pandas as pd
df = pd.DataFrame({'X': [1, 2, 2, 3],
'Y': [4, 3, 8, 4]})
print("DataFrame:")
print(df)
maxs = df.max()
print("Max of Each Column:")
print(maxs)
Output:
DataFrame:
X Y
0 1 4
1 2 3
2 2 8
3 3 4
Max of Each Column:
X 3
Y 8
dtype: int64
It gets the max value for both columns X
and Y
and finally returns a Series
object with the max of each column.
To find the max of a particular column of DataFrame
in Pandas, we call the max()
function for that column only.
import pandas as pd
df = pd.DataFrame({'X': [1, 2, 2, 3],
'Y': [4, 3, 8, 4]})
print("DataFrame:")
print(df)
maxs = df["X"].max()
print("Max of Each Column:")
print(maxs)
Output:
DataFrame:
X Y
0 1 4
1 2 3
2 2 8
3 3 4
Max of Each Column:
3
It only gives the max of values of column X
in the DataFrame
.
Example Codes: DataFrame.max()
Method to Find Max Along Row Axis
import pandas as pd
df = pd.DataFrame({'X': [1, 2, 7, 5, 10],
'Y': [4, 3, 8, 2, 9],
'Z': [2, 7, 6, 10, 5]})
print("DataFrame:")
print(df)
maxs=df.max(axis=1)
print("Max of Each Row:")
print(maxs)
Output:
DataFrame:
X Y Z
0 1 4 2
1 2 3 7
2 7 8 6
3 5 2 10
4 10 9 5
Max of Each Row:
0 4
1 7
2 8
3 10
4 10
dtype: int64
It calculates the max for all the rows and finally returns a Series
object with the max of each row.
Example Codes: DataFrame.max()
Method to Get Max Ignoring NaN
Values
We use the default value of skipna
parameter i.e. skipna=True
to find the max of DataFrame
along the specified axis ignoring NaN
values.
import pandas as pd
df = pd.DataFrame({'X': [1, 2, None, 3],
'Y': [4, 3, 7, 4]})
print("DataFrame:")
print(df)
maxs=df.max(skipna=True)
print("Max of Columns")
print(maxs)
Output:
DataFrame:
X Y
0 1.0 4.0
1 2.0 3.0
2 NaN 7.0
3 3.0 4.0
Max of Columns
X 3.0
Y 7.0
dtype: float64
If we set skipna=True
, it ignores the NaN
in the dataframe. It allows us to calculate the max of DataFrame
along column axis ignoring NaN
values.
import pandas as pd
df = pd.DataFrame({'X': [1, 2, None, 3],
'Y': [4, 3, 7, 4]})
print("DataFrame:")
print(df)
maxs=df.max(skipna=False)
print("Max of Columns")
print(maxs)
Output:
DataFrame:
X Y
0 1.0 4
1 2.0 3
2 NaN 7
3 3.0 4
Max of Columns
X NaN
Y 7.0
dtype: float64
Here, we get NaN
value for the max value of column X
as column X
has NaN
value present in it.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook