Matplotlib 中 cla()、clf()和 close()方法之间的区别
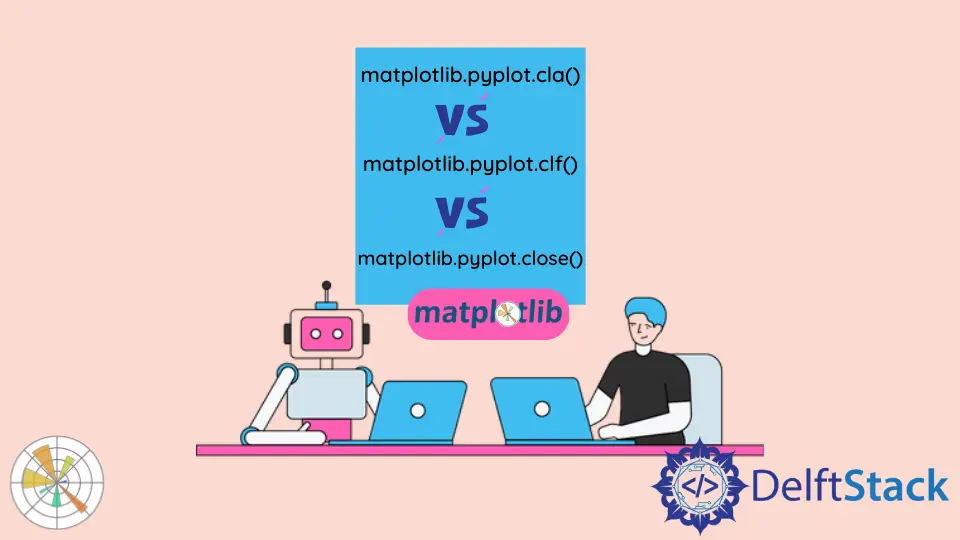
matplotlib.pyplot.cla()
方法清除当前坐标轴,matplotlib.pyplot.clf()
方法清除当前图形,matplotlib.pyplot.close()
方法关闭整个窗口。
import math
import numpy as np
import matplotlib.pyplot as plt
x = np.linspace(0, 2 * math.pi, 100)
y1 = np.sin(x)
y2 = np.cos(x)
fig, ax = plt.subplots(2, 1)
ax[0].plot(x, y1)
ax[0].set_xlabel("x")
ax[0].set_ylabel("sinx")
ax[0].set_title("Plot of sinx")
ax[1].plot(x, y2)
ax[1].set_xlabel("x")
ax[1].set_ylabel("cosx")
ax[1].set_title("Plot of cosx")
fig.suptitle("Plot of sinx and cosx", fontsize=16)
fig.tight_layout(pad=3.0)
plt.show()
输出:
我们将用这个图来解释以下函数:cla()
、clf()
和 close()
。该图由一个图和两个子图组成,上排的子图是 sinx
函数的图,下排的子图表示 cosx
函数的图。
matplotlib.pyplot.cla()
matplotlib.pyplot.cla()
命令用于清除 Matplotlib 中的当前坐标轴。Axes
只是一个图的一部分,通常是一个子图及其细节。
例如:matplotlib.pyplot.cla()
import math
import numpy as np
import matplotlib.pyplot as plt
x = np.linspace(0, 2 * math.pi, 100)
y1 = np.sin(x)
y2 = np.cos(x)
fig, ax = plt.subplots(2, 1)
ax[0].plot(x, y1)
ax[0].set_xlabel("x")
ax[0].set_ylabel("sinx")
ax[0].set_title("Plot of sinx")
ax[1].plot(x, y2)
ax[1].set_xlabel("x")
ax[1].set_ylabel("cosx")
ax[1].set_title("Plot of cosx")
ax[1].cla()
fig.suptitle("Plot of sinx and cosx", fontsize=16)
fig.tight_layout(pad=3.0)
plt.show()
输出:
在这里,我们可以看到,cla()
方法清除了 ax[1]
轴,即子图的第二行。清除轴意味着删除子图及其细节,如 xlabel
、ylabel
和 title
;但是,轴 ax[0]
或顶部的子图没有被该方法改变,因为 cla()
只被 ax[1]
轴调用。
matplotlib.pyplot.cla()
matplotlib.pyplot.clf()
可以清除 Matplotlib 中的整个图。图形可以看作是一个画图的超集,由画图中的每一个细节组成,如子图、子轴、标题和图例。
例:matplotlib.pyplot.clf()
import math
import numpy as np
import matplotlib.pyplot as plt
x = np.linspace(0, 2 * math.pi, 100)
y1 = np.sin(x)
y2 = np.cos(x)
fig, ax = plt.subplots(2, 1)
ax[0].plot(x, y1)
ax[0].set_xlabel("x")
ax[0].set_ylabel("sinx")
ax[0].set_title("Plot of sinx")
ax[1].plot(x, y2)
ax[1].set_xlabel("x")
ax[1].set_ylabel("cosx")
ax[1].set_title("Plot of cosx")
fig.suptitle("Plot of sinx and cosx", fontsize=16)
fig.tight_layout(pad=3.0)
plt.clf()
plt.show()
输出:
在这里,我们可以看到 clf()
方法清除了绘图中的所有内容。这个过程包括了所有的坐标轴;但是,绘图窗口仍然存在,它可以被重新使用来生成其他的图形。
请注意,我们不能对每个轴使用 clf()
方法。
matplotlib.pyplot.close()
matplotlib.pyplot.close()
只是关闭了 Matplotlib 中的图窗口,我们在调用 plt.show()
方法时不会看到任何东西。
例:matplotlib.pyplot.close()
import math
import numpy as np
import matplotlib.pyplot as plt
x = np.linspace(0, 2 * math.pi, 100)
y1 = np.sin(x)
y2 = np.cos(x)
fig, ax = plt.subplots(2, 1)
ax[0].plot(x, y1)
ax[0].set_xlabel("x")
ax[0].set_ylabel("sinx")
ax[0].set_title("Plot of sinx")
ax[1].plot(x, y2)
ax[1].set_xlabel("x")
ax[1].set_ylabel("cosx")
ax[1].set_title("Plot of cosx")
fig.suptitle("Plot of sinx and cosx", fontsize=16)
fig.tight_layout(pad=3.0)
plt.close()
plt.show()
脚本不会产生任何输出,因为 close()
方法会清除图形并关闭窗口;我们使用 plt.show()
过程不会看到任何东西。
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn