How to Get Weighted Random Choice Using Python
-
Use the
random.choices()
Function to Generate Weighted Random Choices -
Use the
numpy.random.choice()
Function to Generate Weighted Random Choices
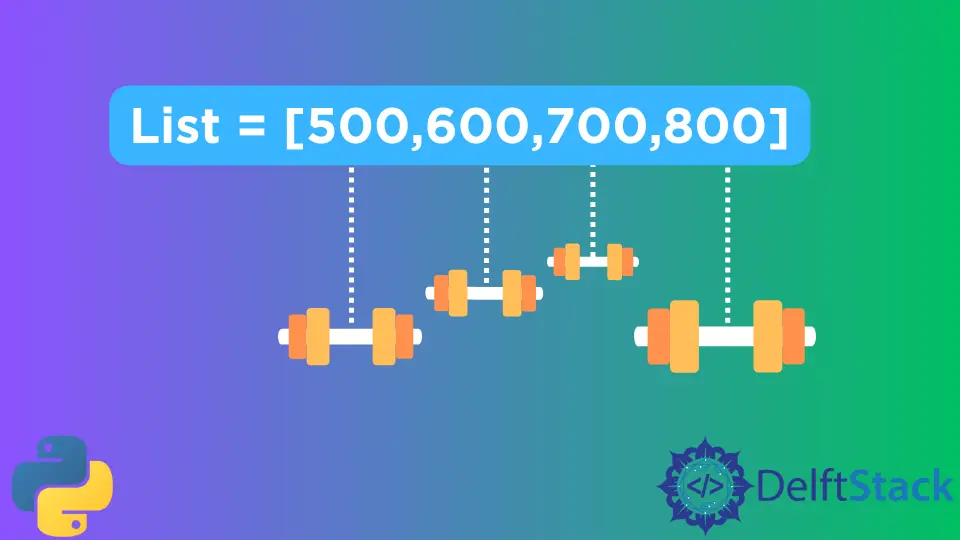
In Python, we can easily generate random numbers using Random and NumPy libraries.
Selecting random elements from a list or an array by the probable outcome of the element is known as Weighted Random Choices. The selection of an element is determined by assigning a probability to each element present. Sometimes more than one element is also selected from the list of the elements made.
In this tutorial, we will discuss how to generate weighted random choices in Python.
Use the random.choices()
Function to Generate Weighted Random Choices
Here, the random
module of Python is used to make random numbers.
In the choices()
function, weighted random choices are made with a replacement. It is also known as the weighted random sample with replacement. Also, in this function, weights play an essential role. Weights define the probable outcome of the selection of each element. There are two types of weights:
- Relative Weights
- Cumulative Weights
Choose Elements With Relative Weights
The weights
parameter defines the relative weights. The probable outcome is different for each element in the list. If the probable outcome for each element has been fixed using the relative weights, then the selections are made based on the relative weights only.
Here is an example:
import random
List = [12, 24, 36, 48, 60, 72, 84]
print(random.choices(List, weights=(30, 40, 50, 60, 70, 80, 90), k=7))
Here each element in the list is given its own weight i.e, probable outcome. Also, k in the above example is the number of elements needed from the given list.
Output:
[60, 84, 36, 72, 84, 84, 60]
Here, the total sum of weights is not 100 because they are relative weights and not percentages. The number 84 has occurred three times as it has the highest weight of all weights. So the probability of its occurrence will be the highest.
Choose Elements With Cumulative Weights
The cum_weight
parameter is used to define the cumulative weights. The cumulative weight of an element is determined by the weight of the preceding element plus the relative weight of that element. For example, the relative weights [10, 20, 30, 40] are equivalent to the cumulative weights [10, 30, 60, 100]
Here is an example:
import random
List = [13, 26, 39, 52, 65]
print(random.choices(List, cum_weights=(10, 30, 60, 100, 150), k=5))
Output:
[65, 65, 39, 13, 52]
Here also, the number 65 occurs more than any other number as it has the highest weight.
Use the numpy.random.choice()
Function to Generate Weighted Random Choices
For generating random weighted choices, NumPy is generally used when a user is using the Python version less than 3.6.
Here, numpy.random.choice
is used to determine the probability distribution. In this method, random elements of 1D array are taken, and random elements of a numpy array are returned using the choice()
function.
import numpy as np
List = [500, 600, 700, 800]
sNumbers = np.random.choice(List, 4, p=[0.10, 0.20, 0.30, 0.40])
print(sNumbers)
Here, the probability should be equal to 1. The number 4 represents the size of the list.
Output:
[800 500 600 800]
Lakshay Kapoor is a final year B.Tech Computer Science student at Amity University Noida. He is familiar with programming languages and their real-world applications (Python/R/C++). Deeply interested in the area of Data Sciences and Machine Learning.
LinkedIn