How to Create Random Letter Generator in Python
-
Use the
random
andstring
Modules to Generate a Random Letter in Python -
Use the
secrets
Module to Generate a Random Letter in Python -
Use the
random.randint()
Function to Generate a Random Letter in Python
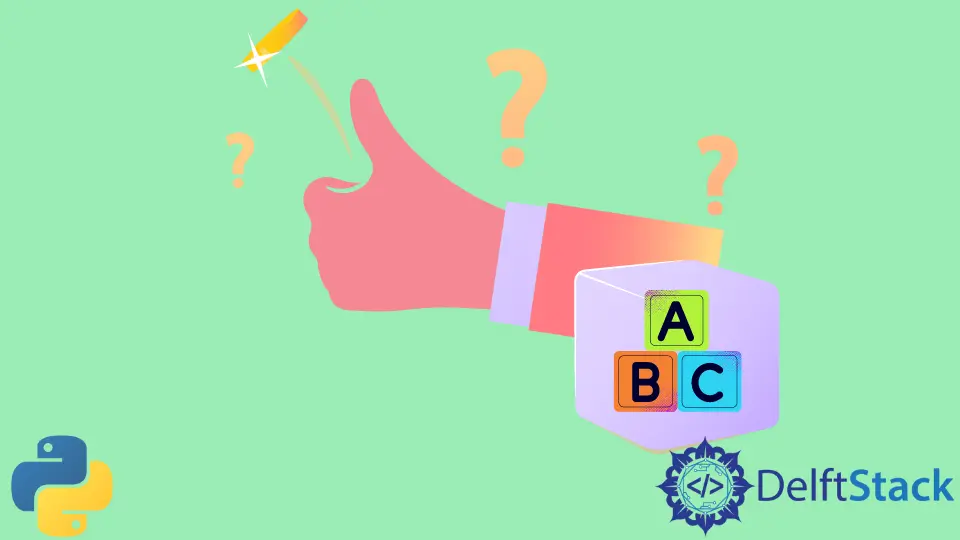
Python provides built-in modules that help with generating random numbers and letters. We can use multiple ways to implement these built-in modules to generate a random letter in Python.
This tutorial demonstrates the different ways available to generate a random letter in Python.
Use the random
and string
Modules to Generate a Random Letter in Python
Python contains the random
module, which can be imported to the Python program. It also includes some functions that you can use to generate random letters as per the programmer’s needs.
In this case, you can use the random.choice()
function contained within the random
module. The random.choice()
function is utilized to return an element that is randomly selected from a specified sequence.
The string
module provides functions that deal with the string. One particular constant, ascii.letters
is utilized to return a string that contains the ranges (A-Z)
and (a-z)
, which basically means the ranges of uppercase and lowercase letters.
The following code uses the random
and string
module to generate a random letter in Python.
import string
import random
if __name__ == "__main__":
rstr = random.choice(string.ascii_letters)
print(rstr)
The code above provides the following output.
v
Use the secrets
Module to Generate a Random Letter in Python
The secrets
module can be utilized to generate cryptographically stable, secure, and unpredictable random numbers. It is also majorly used in generating and maintaining important security-related data like passwords, account authentication, security tokens, and URLs.
It is the most secure way to generate random numbers in Python due to its major focus on security and is available to use in all Python versions after Python 3.6.
Similar to the random
module, the secrets
module also contains the choice()
function that can be utilized to generate a random letter in Python.
The following code uses the secrets
module to generate a random letter in Python.
import string
import secrets
if __name__ == "__main__":
rand = secrets.choice(string.ascii_letters)
print(rand)
The code above provides the following output:
c
Use the random.randint()
Function to Generate a Random Letter in Python
The random.randint()
function can be utilized to return a random number within a specified range; the programmer can specify the range. The random.randint()
function is contained within the built-in random
module provided by Python, which needs to be imported to the Python code in order to use this function.
The random.randint()
function is an alias for the random.randrange()
function and it contains two mandatory parameters: start
and stop
. These parameters specify the range between which we want to generate a random number or a letter.
To generate a random letter in Python, the same random.randint()
function can be implemented.
The following code uses the random.randint()
function to generate a random letter in Python.
import random
randlowercase = chr(random.randint(ord("a"), ord("z")))
randuppercase = chr(random.randint(ord("A"), ord("Z")))
print(randlowercase, randuppercase)
The code program provides the following output.
s K
As all the codes mentioned in this article are for generating a random letter in Python, the output will vary every time the code will get executed.
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn