How to Generate Random Integers in Range in Python
-
Use the
random.randint()
Function to Generate Random Integers Between a Specific Range in Python -
Use the
random.randrange()
Function to Generate Random Integers Between a Specific Range in Python -
Use the
random.sample()
Function to Generate Random Integers Between a Specific Range in Python -
Use the
NumPy
Module to Generate Random Integers Between a Specific Range in Python
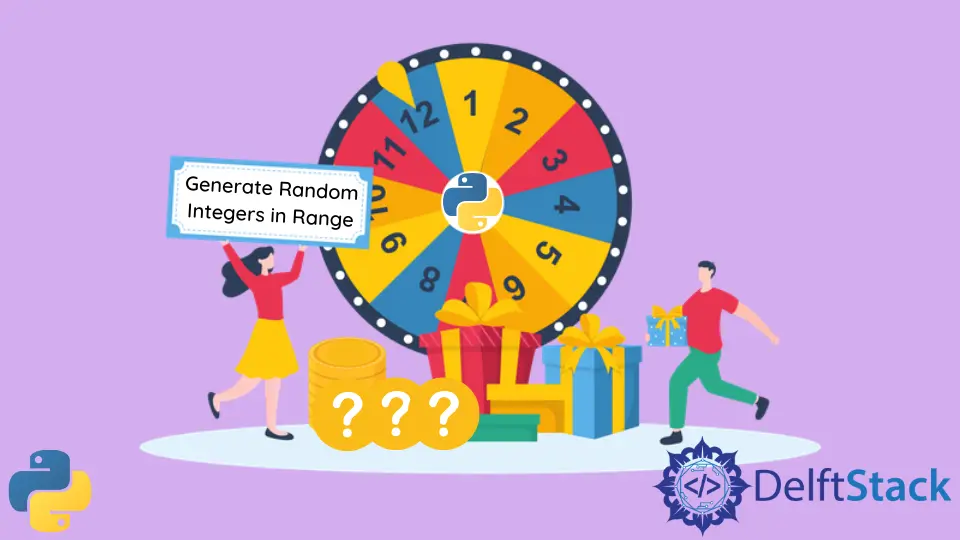
Python is a very highly useful tool for Data Analysis. When we deal with real-world scenarios, we have to generate random values to simulate situations and work on them.
Python has the random
and the NumPy
module available, which have efficient methods available for working and generating random numbers with ease.
In this tutorial, we will generate some random integers between a specific range in Python.
Use the random.randint()
Function to Generate Random Integers Between a Specific Range in Python
The randint()
function is used to generate random integers between a specified range. The start and end positions are passed to the function as parameters.
For example,
import random
x = random.randint(0, 10)
print(x)
Output:
8
To generate a list of random numbers with this function, we can use the list comprehension method with the for
loop as shown below:
import random
x = [random.randint(0, 9) for p in range(0, 10)]
print(x)
Output:
[1, 6, 6, 5, 8, 8, 5, 5, 8, 4]
Note that this method only accepts integer values.
Use the random.randrange()
Function to Generate Random Integers Between a Specific Range in Python
The randrange()
function also returns a random number within a range and accepts only integer values, but here we have the option to specify a very useful parameter called step
. The step
parameter allows us to find a random number that is divisible by a specific number. By default, this parameter is 1.
For example,
import random
x = random.randrange(0, 10, 2)
print(x)
Output:
4
Note that the output is divisible by 2. Using the same list comprehension method, we can generate a list of random numbers with this function, as shown below.
import random
x = [random.randrange(0, 10, 2) for p in range(0, 10)]
print(x)
Output:
[8, 0, 6, 2, 0, 6, 8, 6, 0, 4]
Use the random.sample()
Function to Generate Random Integers Between a Specific Range in Python
With this function, we can specify the range and the total number of random numbers we want to generate. It also ensures no duplicate values are present. The following example shows how to use this function.
import random
x = random.sample(range(10), 5)
print(x)
Output:
[7, 8, 5, 9, 6]
Use the NumPy
Module to Generate Random Integers Between a Specific Range in Python
The NumPy
module also has three functions available to achieve this task and generate the required number of random integers and store them in a numpy array.
These functions are numpy.random.randint()
, numpy.random.choice()
, and numpy.random.uniform()
. The following code shows how to use these functions.
For example,
import numpy as np
x1 = np.random.randint(low=0, high=10, size=(5,))
print(x1)
x2 = np.random.uniform(low=0, high=10, size=(5,)).astype(int)
print(x2)
x3 = np.random.choice(a=10, size=5)
print(x3)
Output:
[3 2 2 2 8]
[8 7 9 2 9]
[0 7 4 1 4]
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn