在 Python 中生成範圍內的隨機整數
-
在 Python 中使用
random.randint()
函式在特定範圍之間生成隨機整數 -
在 Python 中使用
random.randrange()
函式在特定範圍之間生成隨機整數 -
在 Python 中使用
random.sample()
函式在特定範圍之間生成隨機整數 -
在 Python 中使用
NumPy
模組在特定範圍之間生成隨機整數
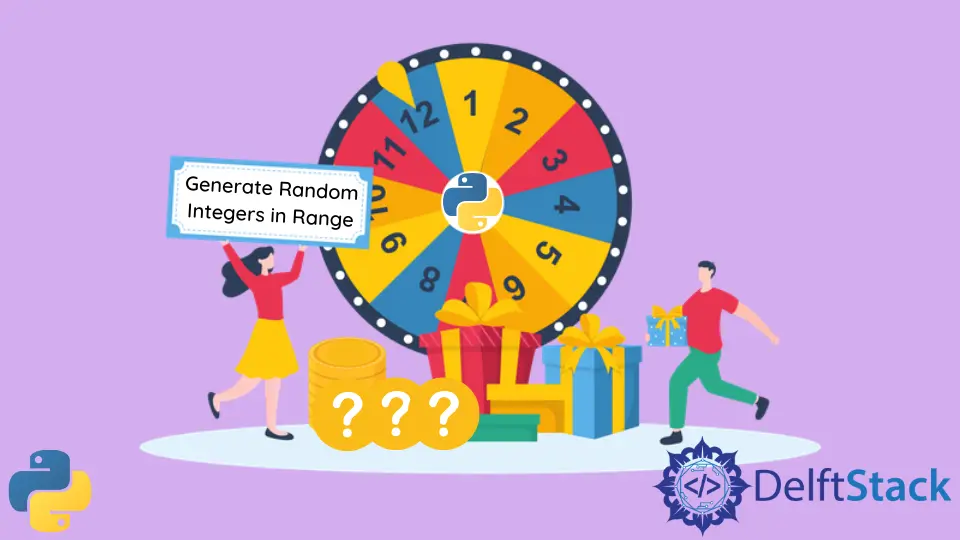
Python 是用於資料分析的非常有用的工具。當我們處理現實情況時,我們必須生成隨機值以模擬情況並對其進行處理。
Python 具有可用的 random
和 NumPy
模組,它們具有有效的方法,可輕鬆地工作和生成隨機數。
在本教程中,我們將在 Python 的特定範圍之間生成一些隨機整數。
在 Python 中使用 random.randint()
函式在特定範圍之間生成隨機整數
randint()
函式用於生成指定範圍之間的隨機整數。起點和終點位置作為引數傳遞給函式。
例如,
import random
x = random.randint(0, 10)
print(x)
輸出:
8
要使用此函式生成一個隨機數列表,我們可以將列表推導方法與 for
迴圈一起使用,如下所示:
import random
x = [random.randint(0, 9) for p in range(0, 10)]
print(x)
輸出:
[1, 6, 6, 5, 8, 8, 5, 5, 8, 4]
請注意,此方法僅接受整數值。
在 Python 中使用 random.randrange()
函式在特定範圍之間生成隨機整數
randrange()
函式還返回一個範圍內的隨機數,並且僅接受整數值,但是這裡我們可以選擇指定一個非常有用的引數稱為 step
。step
引數使我們能夠找到可被特定數字整除的隨機數字。預設情況下,該引數為 1。
例如,
import random
x = random.randrange(0, 10, 2)
print(x)
輸出:
4
請注意,輸出可被 2 整除。使用相同的列表推導方法,我們可以使用此函式生成一個隨機數列表,如下所示。
import random
x = [random.randrange(0, 10, 2) for p in range(0, 10)]
print(x)
輸出:
[8, 0, 6, 2, 0, 6, 8, 6, 0, 4]
在 Python 中使用 random.sample()
函式在特定範圍之間生成隨機整數
使用此函式,我們可以指定要生成的隨機數的範圍和總數。它還可以確保不存在重複的值。以下示例顯示瞭如何使用此函式。
import random
x = random.sample(range(10), 5)
print(x)
輸出:
[7, 8, 5, 9, 6]
在 Python 中使用 NumPy
模組在特定範圍之間生成隨機整數
NumPy
模組還具有三個函式可用於完成此任務,並生成所需數量的隨機整數並將其儲存在 numpy 陣列中。
這些函式是 numpy.random.randint()
,numpy.random.choice()
和 numpy.random.uniform()
。以下程式碼顯示瞭如何使用這些函式。
例如,
import numpy as np
x1 = np.random.randint(low=0, high=10, size=(5,))
print(x1)
x2 = np.random.uniform(low=0, high=10, size=(5,)).astype(int)
print(x2)
x3 = np.random.choice(a=10, size=5)
print(x3)
輸出:
[3 2 2 2 8]
[8 7 9 2 9]
[0 7 4 1 4]
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn