How to Generate Random 4 Digit Number in Python
- Generate Random Numbers in Python
-
Use the
random
Module to Generate Random Numbers in Python - Use an Alternative Method to Generate Random Numbers in Python
- Conclusion
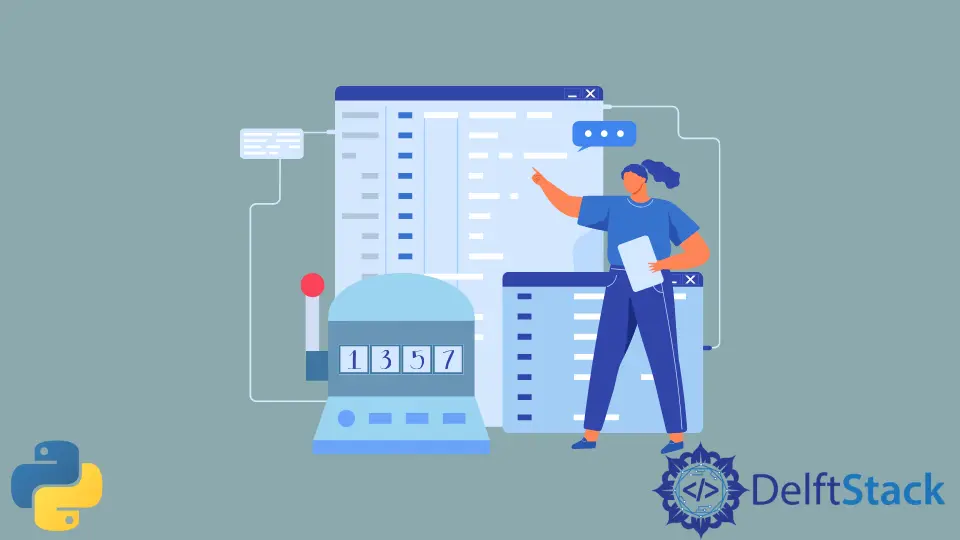
Python is a high-level and interpreted programming language that most programmers use worldwide. It is prevalent in object-oriented programming (OOP).
Moreover, Python serves most IT sectors, such as Artificial Intelligence, Machine Learning, Computer Vision, Web application development, Data visualization, analysis, etc. Also, there are so many libraries that we can use to fulfill various requirements, and debugging has become easy with the libraries that Python has.
Generate Random Numbers in Python
As mentioned above, we can do various things using Python libraries, like creating virtual environments, unit testing, creating sudoku solvers, etc. Another simple activity we can do using Python is generating random numbers.
Sometimes when coding, we might need random numbers with a different numbers of digits. We can use it for passwords, security pins for devices, etc.
Use the random
Module to Generate Random Numbers in Python
To achieve those goals, Python provides us with the random()
module. random()
is an in-built Python module created to generate random numbers.
It has a lot of functions such as seed()
, randrange()
, randint()
, choice()
, choices()
, and shuffle()
to complete various tasks.
This article discusses how to generate a four-digit number using the randint()
and randrange()
methods. Also, we discuss an alternative path to have a random four-digit number.
Use the random.randint()
Method
There are many ways to generate a random four-digit number, but Python provides a simple path. Using the randint()
method of the random
module, we can easily create a random four-digit number.
The syntax for the randint()
method is below.
randint(range1, range2)
Here, the range1
is the starting value of the range. range2
is the end value of the range.
Let’s try to generate a random number using this method. First, we should import the random
module since it includes the randint()
method.
import random
Now we can generate a random number using this method.
randomNumber = random.randint(1000, 9999)
Since we need a random four-digit number, we have put 1000
as the starting value and 9999
as the end value. Now, let’s print the value using a print
function.
print(randomNumber)
Full code:
import random
randomNumber = random.randint(1000, 9999)
print(randomNumber)
Output:
As you can see, it delivers us a random four-digit number each time we run the code.
Use the random.randrange()
Method
randrange()
method is also similar to the randint()
method. Through this method, we can generate a random number.
Syntax:
randrange(range1, range2)
Like the randint()
method, range1
is the starting value, and range2
is the end value of the range.
To generate a random four-digit number, first, we have to import the random
module, and then we should give the range and assign it to a variable. Then we can print the output.
import random
randomNumber = random.randrange(1000, 9999)
print(randomNumber)
After running the code, we get the below result.
As you can see, we get a random four-digit number each time we run the code.
Use an Alternative Method to Generate Random Numbers in Python
Other than the randint()
and randrange()
methods, we can build a code to generate random four-digit numbers simply with the help of the Python string
module, digits
function in the string
module, join
operation, the choice
function of the random
module, and a for
loop.
First, we can import the choice
method from the random
module.
from random import choice
In the next step, we need to get numbers from 0 to 9 to generate a random four-digit number. To do that, we can use the digits
method of the string
module.
The digits()
method provides a text string, including the numbers from 0 to 9.
First, we import the string
module to the code.
import string
Then we use the string.digits()
method to get the numbers. We can assign it to a variable for ease of use.
numbers = string.digits
Now we can select four random numbers from the numbers
variable using the choice()
method and join them as a string. We use the join
method, choice
method, and a for
loop.
randomNumber = "".join(choice(numbers) for _ in range(4))
Here the value 4
in the for
loop is the number of digits of the output we want. If we change it to five, we get a random number with five digits.
But in our case, we only need to generate four-digit numbers, so we have added four as the value.
Then we can print the value using a print
function.
print(randomNumber)
Full code:
from random import choice
import string
numbers = string.digits
randomNumber = "".join(choice(numbers) for _ in range(4))
print(randomNumber)
Output:
As in the above output, we will get a random four-digit number every time we execute the code.
Conclusion
Throughout this article, we briefly introduced Python and the random
module in Python. Also, we discussed how to use the randint()
and randrange()
methods to generate random four-digit numbers.
Then we learned an alternative path to achieve this task along with some modules and methods: string
, digits
, choice
, and join
.
We can generate these kinds of random numbers using lists and for
loops, but randint()
and randrange()
are the easiest ways to do this.
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.