How to Generate a List of Random Numbers in Python
-
Use the
random.sample()
Function to Generate Random Integers in Python -
Use the
random.randint()
Function to Generate Random Integers in Python -
Use the
numpy.random.randint()
Function to Generate Random Integers in Python -
Use the
numpy.random.uniform()
Function to Generate Random Float Numbers in Python
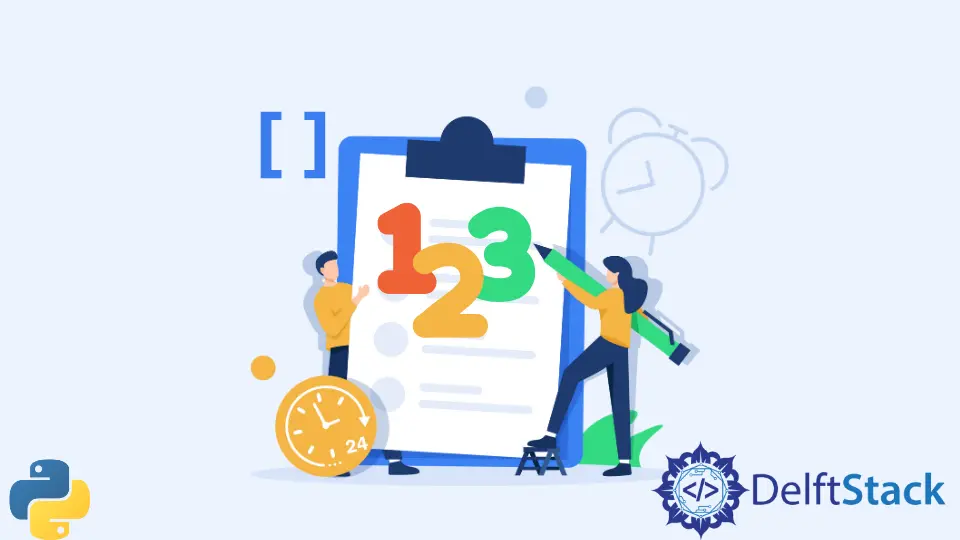
This article introduces different methods you can follow to generate a list of random numbers in Python. Check this list below.
Use the random.sample()
Function to Generate Random Integers in Python
Use the random.sample()
function to generate random numbers in Python. The result is a Python list containing the random numbers. Check this syntax below.
random.sample(population_sequence, total_count_of_random_numbers)
The total number of random numbers generated is total_count_of_random_numbers
. Check this example below.
import random
random_numbers = random.sample(range(6), 3)
print(random_numbers)
type(random_numbers)
Here, 3 numbers are generated in the range of numbers from 0 to 6.
Output:
[3, 0, 1]
list
Use the random.randint()
Function to Generate Random Integers in Python
Use a list comprehension using the random.randint()
function to generate random numbers in Python. The result is a Python list containing the random numbers. The syntax is the following below.
random_numbers = [random.randint( < min_value > , < min_value > ) for x in range(total_count_of_random_numbers)]
Here’s an example.
import random
random_numbers = [random.randint(0, 6) for x in range(3)]
print(random_numbers)
Here, 3 numbers are generated in the range of numbers from 0 to 6.
Output:
[1, 6, 6]
Use the numpy.random.randint()
Function to Generate Random Integers in Python
Use the numpy.random.randint()
function to generate random integers. The result is a Python list containing the random numbers. For this, we first import the NumPy
library. You can use this syntax.
np.random.randint(low=min_val, high=max_val, size=count).tolist()
Take this code snippet, for example.
import numpy as np
random_numbers = np.random.randint(low=0, high=6, size=3).tolist()
print(random_numbers)
Here, 3 numbers are generated in the range of numbers from 0 to 6.
Output:
[2, 4, 2]
Use the numpy.random.uniform()
Function to Generate Random Float Numbers in Python
Use numpy.random.uniform
to generate random float numbers in Python.
First, import the NumPy
library to utilize the function. The syntax is the following.
random.uniform(low=minvalue, high=maxvalue, size=count_of_numbers)
Follow this example.
import numpy
random_numbers = numpy.random.uniform(low=0, high=6, size=10).tolist()
print(random_numbers)
Here, 10 float numbers are generated in the range of numbers from 0 to 6.
Output:
[0.3077335256902074,
4.305975943414238,
4.487914411717991,
1.141532770555624,
2.849062698503963,
3.7662017493968922,
2.822739788956107,
4.5291155985333065,
3.5138714366365296,
3.7069530642450745]