Python 中的随机字母生成器
-
在 Python 中使用
random
和string
模块生成随机字母 -
在 Python 中使用
secrets
模块生成随机字母 -
在 Python 中使用
random.randint()
函数生成随机字母
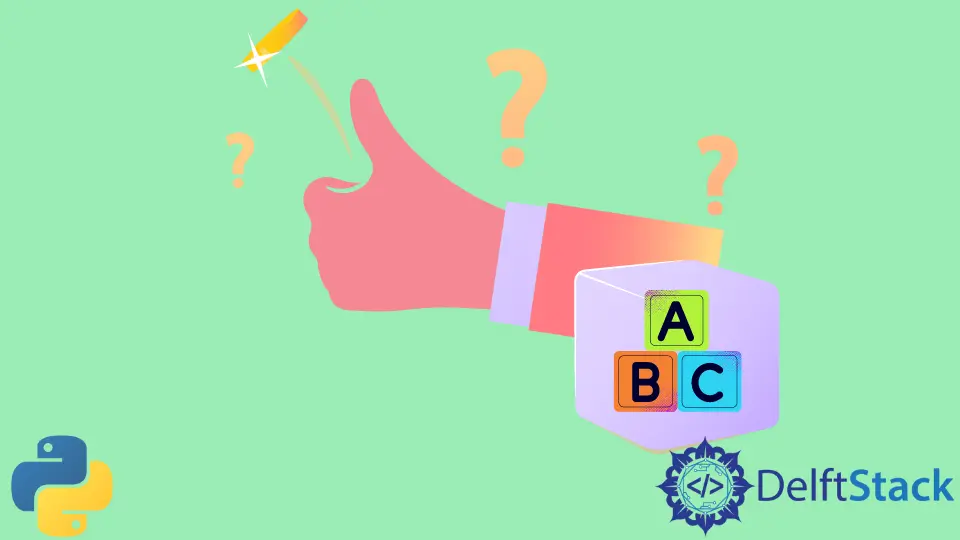
Python 提供了有助于生成随机数和字母的内置模块。我们可以通过多种方式实现这些内置模块,从而在 Python 中生成随机字母。
本教程演示了在 Python 中生成随机字母的不同方法。
在 Python 中使用 random
和 string
模块生成随机字母
Python 包含 random
模块,可以将其导入 Python 程序。它还包括一些函数,你可以使用这些函数根据程序员的需要生成随机字母。
在这种情况下,你可以使用 random
模块中包含的 random.choice()
函数。random.choice()
函数用于返回从指定序列中随机选择的元素。
string
模块提供处理字符串的函数。一个特定的常量 ascii.letters
用于返回包含范围 (A-Z)
和 (a-z)
的字符串,这基本上意味着大写和小写字母的范围。
以下代码使用 random
和 string
模块在 Python 中生成随机字母。
import string
import random
if __name__ == "__main__":
rstr = random.choice(string.ascii_letters)
print(rstr)
上面的代码提供了以下输出。
v
在 Python 中使用 secrets
模块生成随机字母
secrets
模块可用于生成加密稳定、安全和不可预测的随机数。它还主要用于生成和维护重要的安全相关数据,如密码、帐户身份验证、安全令牌和 URL。
由于其主要关注安全性,因此它是在 Python 中生成随机数的最安全方法,并且可用于 Python 3.6 之后的所有 Python 版本。
与 random
模块类似,secrets
模块也包含可用于在 Python 中生成随机字母的 choice()
函数。
以下代码使用 secrets
模块在 Python 中生成随机字母。
import string
import secrets
if __name__ == "__main__":
rand = secrets.choice(string.ascii_letters)
print(rand)
上面的代码提供了以下输出:
c
在 Python 中使用 random.randint()
函数生成随机字母
random.randint()
函数可用于返回指定范围内的随机数;程序员可以指定范围。random.randint()
函数包含在 Python 提供的内置 random
模块中,需要将其导入 Python 代码才能使用此函数。
random.randint()
函数是 random.randrange()
函数的别名,它包含两个强制参数:start
和 stop
。这些参数指定了我们想要生成随机数或字母的范围。
要在 Python 中生成随机字母,可以实现相同的 random.randint()
函数。
以下代码使用 random.randint()
函数在 Python 中生成一个随机字母。
import random
randlowercase = chr(random.randint(ord("a"), ord("z")))
randuppercase = chr(random.randint(ord("A"), ord("Z")))
print(randlowercase, randuppercase)
代码程序提供以下输出。
s K
由于本文中提到的所有代码都是用于在 Python 中生成随机字母,因此每次执行代码时输出都会有所不同。
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn