How to Calculate the Time Difference Between Two Time Strings in Python
-
Calculate the Time Difference Between Two Time Strings in Python Using
datetime.strptime()
-
Calculate the Time Difference Between Two Time Strings in Python Using
time.sleep()
-
Calculate the Time Difference Between Two Time Strings in Python Using
datetime.timedelta()
-
Calculate the Time Difference Between Two Time Strings in Python Using
datetime.fromisoformat()
-
Calculate the Time Difference Between Two Time Strings in Python Using
dateutil.parser
- Conclusion
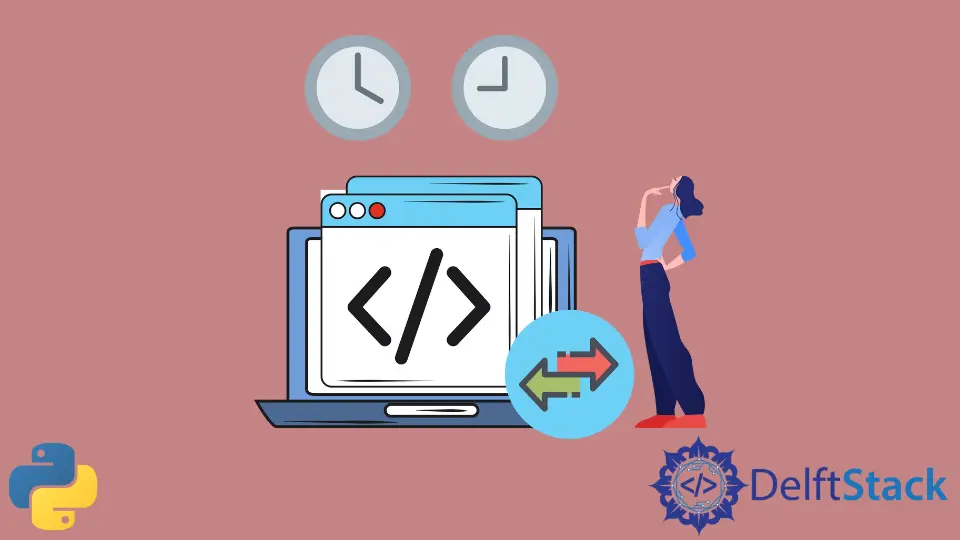
In Python programming, dealing with time-related challenges is a common occurrence. When it comes to calculating the time interval between two time strings, Python provides various methods to tackle the task.
This article explores different approaches, each offering its strengths. From the widely-used datetime.strptime()
method to the convenient time.sleep()
function and the efficient datetime.timedelta()
class, we’ll dive into these methods with practical examples.
Additionally, we’ll explore parsing time strings in ISO 8601 format using datetime.fromisoformat()
and the flexibility offered by the dateutil.parser
module. The goal is to provide Python developers with a clear understanding of how to calculate time intervals effectively.
Calculate the Time Difference Between Two Time Strings in Python Using datetime.strptime()
Python provides a powerful module called datetime
to handle date and time-related operations. When dealing with time intervals between two time strings, the datetime.strptime()
function becomes particularly handy.
The datetime.strptime()
method in Python is part of the datetime
module and is used for parsing a string representing a date and time into a datetime
object. The term strptime
stands for string parse time
.
This function takes two arguments: the string to be parsed and a format string specifying the expected format of the input string. The datetime.strptime()
method follows the syntax:
from datetime import datetime
datetime_object = datetime.strptime(time_string, format)
datetime
: The class within thedatetime
module.time_string
: The input time string to be converted.format
: The format of the input time string.
The datetime.strptime()
method converts a string representing a date and time into a datetime
object based on the specified format. The format should include directives like %Y
for year, %m
for month, %d
for day, %H
for hour, %M
for minute, and %S
for second.
Code Example: Calculating Time Interval Using datetime.strptime()
Here’s an example demonstrating how to calculate the time interval between two time strings in Python using datetime.strptime()
:
from datetime import datetime
time_str_1 = "2022-01-01 12:00:00"
time_str_2 = "2022-01-02 15:30:00"
time_format = "%Y-%m-%d %H:%M:%S"
time_1 = datetime.strptime(time_str_1, time_format)
time_2 = datetime.strptime(time_str_2, time_format)
time_interval = time_2 - time_1
print(time_interval)
In this example, we start by defining two time strings, time_str_1
and time_str_2
. The time_format
variable specifies the format of these time strings, where %Y
, %m
, %d
, %H
, %M
, and %S
represents the year, month, day, hour, minute, and second, respectively.
Next, we use datetime.strptime()
to convert the time strings into datetime
objects (time_1
and time_2
). The time_interval
is then calculated by subtracting time_1
from time_2
.
Finally, the result is printed, showcasing the time interval between the two specified time strings.
This code exemplifies the simplicity and effectiveness of using datetime.strptime()
for calculating time intervals, making it a valuable approach for handling date and time-related tasks in Python.
Code Output:
1 day, 3:30:00
The output represents the time difference between the two specified time strings, displaying the days, hours, and minutes involved.
Calculate the Time Difference Between Two Time Strings in Python Using time.sleep()
Another approach to calculating the time interval between two time strings in Python using the time.sleep()
function.
The time.sleep()
function is part of the time
module in Python and is primarily used to pause the execution of a program for a specified number of seconds. Although its primary purpose is to introduce delays, we can cleverly manipulate it to calculate time intervals between two time strings.
The time.sleep()
method follows the syntax:
import time
time.sleep(seconds)
time
: The module provides time-related functionalities.seconds
: The duration for which the code execution will be suspended.
The time.sleep()
function suspends the execution of the current code block for the specified duration, allowing us to create a time delay. By capturing the time before and after the sleep duration, we can calculate the time interval between the two points.
Code Example: Calculating Time Interval Using time.sleep()
Here’s an example demonstrating how to calculate the time interval between two time strings in Python using time.sleep()
:
import time
time_1 = time.time()
time.sleep(20)
time_2 = time.time()
time_interval = time_2 - time_1
print(time_interval)
In this example, we import the time
module and capture the starting time using the time.time()
function, which returns the current time in seconds since the epoch (January 1, 1970). We then introduce a 20-second sleep using time.sleep(20)
. After the sleep, we capture the ending time.
The time interval is calculated by subtracting the starting time (time_1
) from the ending time (time_2
). The resulting value in time_interval
represents the time difference between the two points in the program.
Code Output:
20.005916118621826
The output displays the time interval between capturing the starting time and capturing the ending time after the 20-second sleep.
Calculate the Time Difference Between Two Time Strings in Python Using datetime.timedelta()
Yet another method to calculate the time interval between two time strings in Python is the datetime.timedelta()
class. This approach is particularly versatile, allowing us to represent specific time durations or differences between two dates and times.
The datetime.timedelta()
method follows the syntax:
from datetime import timedelta
time_difference = timedelta(days=days, hours=hours, minutes=minutes, seconds=seconds)
timedelta
: The class within thedatetime
module.days
,hours
,minutes
,seconds
: Arguments representing the time duration.
The datetime.timedelta()
class allows us to create a timedelta object representing a specific duration. We can specify the duration using arguments such as days, hours, minutes, and seconds.
By subtracting one timedelta
object from another, we can easily calculate the time difference between two points in time.
Code Example: Calculating Time Interval Using datetime.timedelta()
Below is an example demonstrating how to calculate the time interval between two time strings in Python using datetime.timedelta()
:
from datetime import timedelta
time_1 = timedelta(hours=10, minutes=20, seconds=30)
time_2 = timedelta(hours=20, minutes=30, seconds=45)
time_interval = time_2 - time_1
print(time_interval)
In this example, we start by defining two timedelta
objects, time_1
and time_2
, representing specific durations of 10 hours, 20 minutes, and 30 seconds, and 20 hours, 30 minutes, and 45 seconds, respectively.
The time_interval
is then calculated by subtracting time_1
from time_2
. This straightforward approach enables us to precisely measure the time difference between the two specified durations.
Code Output:
10:10:15
The output illustrates the time interval between the two specified timedelta objects, displaying the hours, minutes, and seconds involved.
Calculate the Time Difference Between Two Time Strings in Python Using datetime.fromisoformat()
Another effective method for calculating the time interval between two time strings in Python is by utilizing the datetime.fromisoformat()
method.
The datetime.fromisoformat()
method is part of the datetime
module in Python and is specifically designed to parse date and time strings in the ISO 8601 format. This format follows the YYYY-MM-DDTHH:MM:SS.ssssss
pattern, where T
separates the date and time components.
This method simplifies the process of converting ISO 8601 strings into datetime
objects.
This method follows the following syntax:
from datetime import datetime
datetime_object = datetime.fromisoformat(time_string)
datetime
: The class within thedatetime
module.time_string
: The input time string in ISO 8601 format.
The datetime.fromisoformat()
method creates a datetime
object from the provided ISO-formatted time string, allowing us to perform operations and calculations on the resulting datetime objects.
Code Example: Calculating Time Interval Using datetime.fromisoformat()
Consider the following example demonstrating how to calculate the time interval between two time strings in Python using datetime.timedelta()
:
from datetime import datetime
time_str_1 = "2022-01-01T12:00:00"
time_str_2 = "2022-01-02T15:30:00"
time_1 = datetime.fromisoformat(time_str_1)
time_2 = datetime.fromisoformat(time_str_2)
time_interval = time_2 - time_1
print(time_interval)
In this example, we start by defining two time strings, time_str_1
and time_str_2
, in ISO 8601 format. We then use datetime.fromisoformat()
to convert these time strings into datetime
objects (time_1
and time_2
). The time interval is calculated by subtracting time_1
from time_2
.
This method is especially beneficial when dealing with standardized time representations, such as those required by ISO 8601. It simplifies the process of parsing time strings, ensuring accuracy and ease of use in Python.
Code Output:
1 day, 3:30:00
The output illustrates the time interval between the two specified time strings, displaying the days, hours, and minutes involved.
Calculate the Time Difference Between Two Time Strings in Python Using dateutil.parser
An alternative method for calculating the time interval between two time strings in Python is by leveraging the dateutil.parser
module.
The dateutil.parser
module is not part of the standard library, but it’s a popular third-party library that simplifies the parsing of date and time strings. It’s capable of handling a wide range of formats, making it a versatile tool for dealing with diverse time representations.
To use dateutil.parser
, you need to install the python-dateutil
library first. You can install it using pip
:
pip install python-dateutil
The syntax for parsing time strings using dateutil.parser
is as follows:
from dateutil import parser
datetime_object = parser.parse(time_string)
parser
: The module within thedateutil
library.time_string
: The input time string to be parsed.
The dateutil.parser.parse()
method automatically detects the format of the input time string and creates a datetime
object accordingly.
Code Example: Calculating Time Interval Using dateutil.parser
Take a look at the example below:
from dateutil import parser
time_str_1 = "2022-01-01 12:00:00"
time_str_2 = "2022-01-02 15:30:00"
time_1 = parser.parse(time_str_1)
time_2 = parser.parse(time_str_2)
time_interval = time_2 - time_1
print(time_interval)
Here, we start by defining two time strings, time_str_1
and time_str_2
. We then use parser.parse()
to convert these time strings into datetime
objects (time_1
and time_2
). The time interval is calculated by subtracting time_1
from time_2
.
The dateutil.parser
module excels at automatically recognizing and handling different date and time formats. This makes it a convenient choice for scenarios where the exact format of the time string may vary.
Code Output:
1 day, 3:30:00
The output represents the time interval between the two specified time strings, displaying the days, hours, and minutes involved.
Conclusion
Calculating time intervals between two time strings is a valuable skill for Python developers working on projects involving scheduling, event management, and data analysis. This article has provided insights into various methods, including datetime.strptime()
, time.sleep()
, datetime.timedelta()
, datetime.fromisoformat()
, and dateutil.parser
.
Each approach comes with its advantages, offering flexibility in handling diverse time string formats and addressing specific programming requirements. By incorporating these techniques into their toolkit, developers can efficiently manage time-related challenges, ensuring accurate and precise calculations for a wide range of applications.
As Python continues to evolve, understanding these methodologies will empower you to navigate the complexities of time manipulation with confidence and precision.
Lakshay Kapoor is a final year B.Tech Computer Science student at Amity University Noida. He is familiar with programming languages and their real-world applications (Python/R/C++). Deeply interested in the area of Data Sciences and Machine Learning.
LinkedIn