How to Replace String in File Using Python
-
Use the
replace()
Function When Input and Output Files Are Different -
Use the
replace()
Function When Only One File Is Used for Input and Output
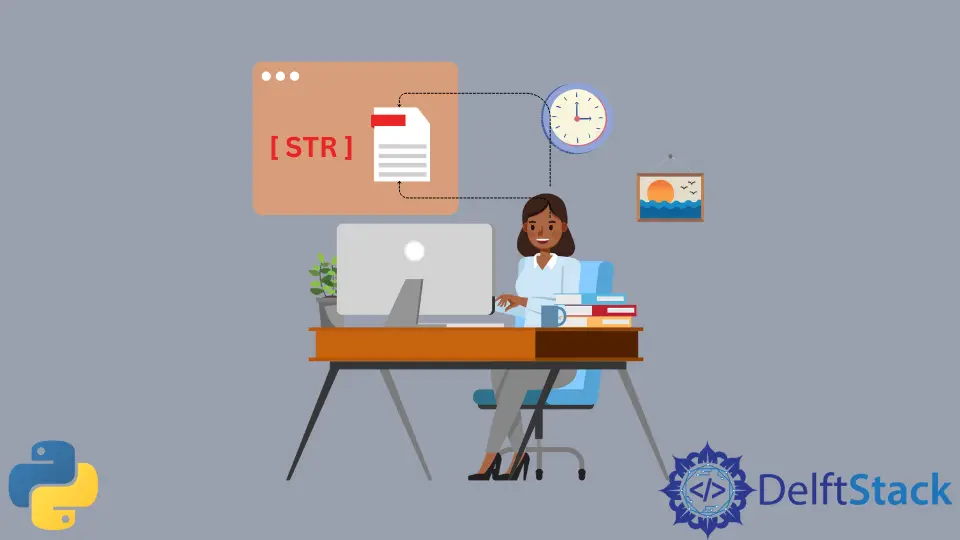
File handling is an essential aspect of any web application. Python, similar to other programming languages, supports file handling. It allows the programmers to deal with files and essentially perform some basic operations like reading, writing, and some other file handling options to operate on files.
The open()
function can be used to open a file in the Python program. The file can be opened in either text or binary mode which is decided by the user. The open()
function has several modes, all of which provide different accessibility options for the file to be opened in.
The term string in Python can be described as a cluster of Unicode characters enclosed within single or double quotes. Strings can be contained inside the text files that are to be opened in the Python code.
This tutorial will discuss different methods to replace a string in a file in Python.
Use the replace()
Function When Input and Output Files Are Different
The replace()
method in Python is utilized to search for a sub-string and replace it with another sub-string.
The replace()
function has three parameters, namely oldvalue
,newvalue
, and count
. Both oldvalue
and newvalue
are required values, and providing the function with the count
parameter is optional.
The following code uses the replace()
function to replace a string in Python when input and output files are different.
# the input file
fin = open("f1.txt", "rt")
# the output file which stores result
fout = open("f2.txt", "wt")
# iteration for each line in the input file
for line in fin:
# replacing the string and write to output file
fout.write(line.replace("gode", "God"))
# closing the input and output files
fin.close()
fout.close()
In the output of the above code, the string gode
in the file will be replaced by the word God
.
In the above code, we simultaneously work on two different files, f1.txt
and f2.txt
. f1.txt
is opened in the read text rt
mode and is referenced to fin
. f2.txt
is opened in the write text wt
mode and is referenced to fout
. Then the for
loop is iterated, and for every occurrence of the string gode
in the file, it gets replaced by the word God
. Both the files are then closed after the necessary operations with the help of the close()
function.
Use the replace()
Function When Only One File Is Used for Input and Output
In this method, the same file is used as both input and output.
We use the with
statement here along with the replace()
function. The with
context manager has a fundamental function: making the program shorter and much more readable.
When we use the with
statement with File handling, the file that we opened in the Python code doesn’t need to be manually closed; it automatically closes after the with
block terminates.
The following code uses the replace()
function to replace a string in Python when the input and output file is the same.
with open("file1.txt", "rt") as file:
x = file.read()
with open("file1.txt", "wt") as file:
x = x.replace("gode", "God")
fin.write(x)
The following code takes file1
as both the input and output file. Firstly, the file is opened in the read text rt
mode, and the contents of the file are read and stored in a variable. Then, the file is closed and opened again, but in the write text mode wt
this time. The string is replaced, and the data is written in this mode, and then the file is closed.
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedInRelated Article - Python File
- How to Get All the Files of a Directory
- How to Append Text to a File in Python
- How to Check if a File Exists in Python
- How to Find Files With a Certain Extension Only in Python
- How to Read Specific Lines From a File in Python
- How to Check if File Is Empty in Python