How to Format a Floating Number to String in Python
-
Format Floating Numbers to a Fixed Width Using Format Specification and the
format()
Method - Format Floating Numbers in a List to a Fixed Width in Python
-
Format a Floating Number to a Fixed Width Using the
%
Operator in Python -
Format a Floating Number to a Fixed Width Using the
round()
Function in Python - Conclusion
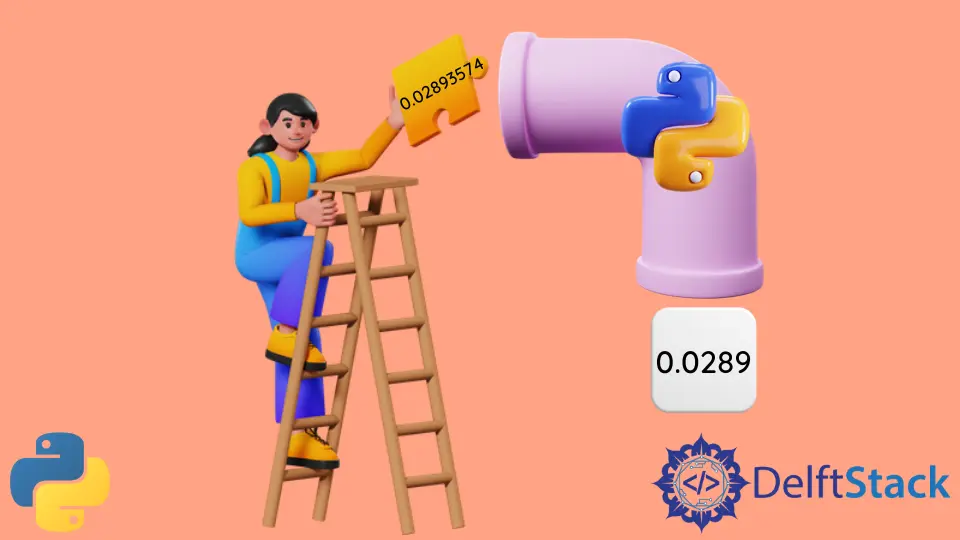
Formatting a floating-point number to a string in Python is a common task. This process involves converting a floating-point value to a string representation with specific formatting, such as a fixed number of decimal places.
This article will introduce some methods to format a floating number to a fixed width in Python.
Format Floating Numbers to a Fixed Width Using Format Specification and the format()
Method
Formatting floating-point numbers to a fixed width using format specification and the format()
method in Python involves employing the format()
function with a specific format specification.
The format specification is a string expression within curly braces ({}
) that defines the desired width, precision, and other formatting options. For the following example, we are using "{:.4f}"
as the format specification ensures that the floating-point number is displayed with four decimal places.
Example Code:
# python 3.x
num = 0.02893574
print("{:.4f}".format(num))
Output:
0.0289
Here, .4f
is called format specification, which denotes that the output should show only four places after the decimal. If we used .5f
instead, we’d get up to five digits after the decimal point, and the empty string before the colon :
is placed so that the argument provided to the format()
function takes that place.
In the above program, the argument we have provided is num
. So, whatever value we have in num
will be passed to the empty string before the :
and formatted accordingly.
In Python 3.6, we can also use f'{}'
to obtain the same output.
Example Code:
# python 3.x
num = 0.02893574
print(f"{num:.4f}")
Output:
0.0289
In this code, the print
statement utilizes an f-string
to format the number to four decimal places with the expression {num:.4f}
. This means that when we run the code, it will output the value of num
with exactly four digits after the decimal point.
The output will be 0.0289
, as the f-string
enforces the precision specified, rounding the number to four decimal places.
Format Floating Numbers in a List to a Fixed Width in Python
Using this process, we’ll iterate through the list and apply a specific format to each element. This format typically includes information about the desired width and precision of the floating-point numbers.
In the following example, we’re using the "{:.3f}"
format specification to ensure that each number in the list is displayed with three decimal places. This process allows for consistent and controlled formatting of floating-point numbers within a list.
Example Code:
# python 3.x
list = [18.292164, 52.452189, 999.1212732]
for numbers in list:
print("{:.3f}".format(numbers))
Output:
18.292
52.452
999.121
In the above code, each number inside the list is sent one by one inside the for
loop. The first element of the list
, i.e., list[0]
, gets assigned to the variable numbers
, and its formatted value, i.e., 18.293
, is printed.
It happened because we executed .3f
, which represents digits up to three places after the decimal point. Similarly, the second element, list[1]
and the third, list[2]
, are also passed to the loop as variable numbers
and are printed accordingly.
This program runs until all the elements in the list are executed. Let’s have another example using the f'{}'
function.
Example Code:
The list below contains the same items as the program above. We will use f'{}'
instead of the format()
function in this example.
# python 3.x
list = [18.292164, 52.452189, 999.1212732]
for numbers in list:
print(f"{numbers:9.3f}")
Output:
18.292
52.452
999.121
We can see that when we got the output when we used f'{}'
. We also got the desired output in an aligned manner.
For the same reason, it is generally better to use f'{}'
if we want to have the floating digits after the decimal aligned.
Format a Floating Number to a Fixed Width Using the %
Operator in Python
We can also set a fixed width for a floating number with the use of the %
operator. The code might look similar to the printf()
function in C programming.
The %
operator is applied to a format specifier, such as "%.4f"
, where %
is followed by the variable containing the floating-point number and the desired format for width and precision.
Example Code:
# python 3.x
num = 0.02893574
print("%.4f" % num)
Output:
0.0289
In this code, we have a floating-point number num
initialized with the value 0.02893574
. Using the %
operator and the format specifier "%.4f"
, we print the value of num
to the console with a fixed width of four decimal places.
The %
operator acts as a placeholder for the variable num
in the format string, and the .4f
specifies that we want to display exactly four digits after the decimal point. When we run the code, the output will be 0.0289
.
Here, the use of %num
has allowed us to print the desired value without any function or string formatting.
Format a Floating Number to a Fixed Width Using the round()
Function in Python
We can also use the round()
function to fix the number of digits after the decimal point. This function limits the number of digits after the decimal point on the input number, and it also rounds off the digit at which limit is set to its upper integral value if the digit is greater than value 5
.
Basic Syntax:
round(number, digits)
In the syntax for the round
function, the argument number
is compulsory, while the argument digits
is optional. The number
can also be put as a variable, and if nothing is passed to the argument digits
, only the integral part of the number
is taken as a result.
In the following example, let’s take a floating-point number and assign it to a variable num
. When we print, we will use the round()
function to limit the number of digits after the decimal point.
Example Code:
# python 3.x
num = 2.37682
print(round(num, 3))
Output:
2.377
In the above code, we passed the value 2.37682
to the first argument num
, and it was rounded to 3
places after the decimal point because we passed the value 3
to the second argument inside the round()
method.
Conclusion
This article demonstrates multiple methods for formatting floating-point numbers to a fixed width in Python. The primary techniques involve using the format()
method, f-strings
, and the %
operator.
The article also explores formatting numbers within a list and introduces the round()
function as an alternative. Python developers can choose the method that best suits their needs, whether prioritizing simplicity, readability, or alignment in output.