What Does // Mean in Python
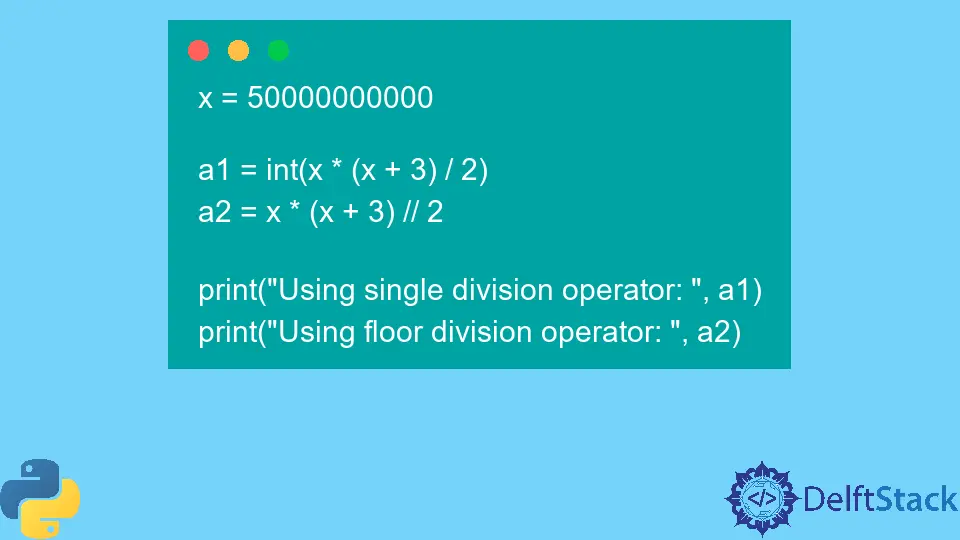
Operators exist in all programming languages and have a fundamental function to perform various operations on values and variables.
Although there are many types of operators in Python, we will focus only on one type of operator in this article: the arithmetic operator.
Arithmetic operators can be utilized to perform simple mathematical operations like addition, multiplication, subtraction, etc.
This tutorial will discuss one such arithmetic operator, the floor division operator.
Use the //
Operator in Python for the Floor Division
The //
operator, also known as a floor division operator, comes under the category of arithmetic operators and is nothing but a division operator that provides an integer as the output and discards the remainder.
The integer division process in Python is not particularly the same as in other popular programming languages, considering it rounds towards negative infinity instead of rounding towards zero.
The //
operator was introduced to the programmers in Python 3 to provide a steadily behaving integer-division operator. In Python 3 and above, the /
or single division operator carries out float-point division, even if the arguments taken are integers.
In the versions of Python before the //
operator was launched, when two integers were divided, the floor division was carried out by default by using the /
operator.
The following code shows the use of the floor division operator.
x = 15
y = 4
ans = x // y
print(ans)
Output:
3
The single division operator tends to behave abnormally and generates inaccurate responses when dealing with huge numbers. The introduction of the floor division or the //
operator masks this drawback of the single division or /
operator.
The following code depicts the difference between both operators when dealing with a large number.
x = 50000000000
a1 = int(x * (x + 3) / 2)
a2 = x * (x + 3) // 2
print("Using single division operator: ", a1)
print("Using floor division operator: ", a2)
Output:
Using single division operator: 1250000000074999922688
Using floor division operator: 1250000000075000000000
In this case, the floor division operator returns the correct result, while the single division operator fails to provide accurate values.
Additionally, the //
operator provides up to 3 times better performance than the /
operator. It helps in significantly boosting the execution of the code.
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn