How to Encrypt a Python String
-
Use
Simple Crypt
to Encrypt a String in Python -
Use the
cryptocode
Library to Encrypt a String in Python -
Use the
Cryptography
Package to Encrypt a String in Python -
Use the
RSA
Algorithm to Encrypt a String in Python
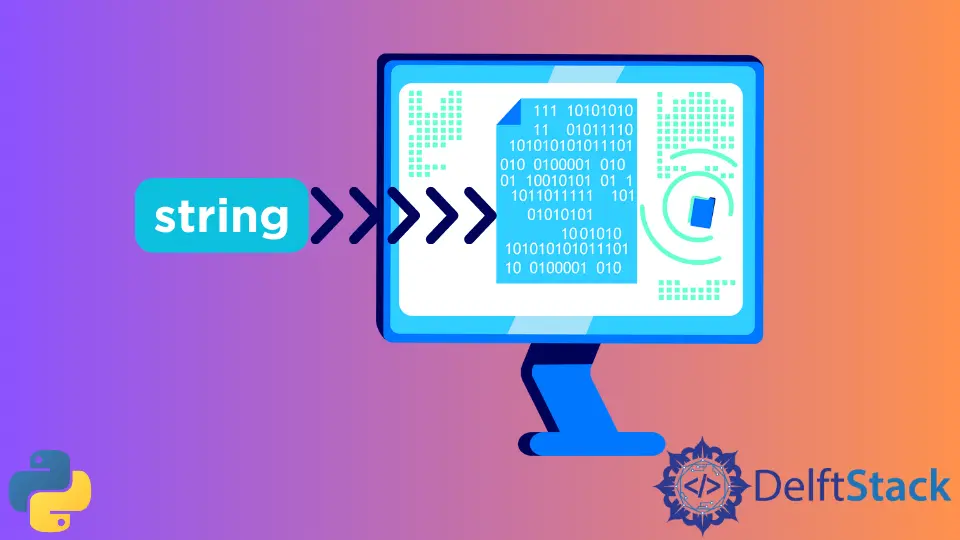
Encryption can be defined as the process that transforms plain text into ciphertext. Essentially, it’s used to encode the data. The encryption process requires a key, which can later be used to decrypt the original message.
This article will discuss the different methods you can utilize to encrypt a string in Python.
Use Simple Crypt
to Encrypt a String in Python
Using the Simple Crypt
module is the fastest and simplest way to achieve the encryption process for Python 2.7 and 3. This method converts plaintext into a ciphertext in seconds, with the help of a single line of code.
The PyCrypto
module supplies the algorithm implementation for this library, and it uses the AES256
cipher. This method incorporates an HMAC
check with the SHA256
cipher to notify when the ciphertext is altered or modified.
Simple Crypt
has two functions: encrypt
and decrypt
. It is essential to install both the pycrypto
and the simplecrypt
modules to use both these functions.
The following code uses simplecrypt
to encrypt a string in Python:
from simplecrypt import encrypt, decrypt
passkey = "wow"
str1 = "I am okay"
cipher = encrypt(passkey, str1)
print(cipher)
The output of this code would be a cluster of random letters.
To decode/decrypt the ciphertext, we will use the following command:
print(decrypt("wow", cipher))
Output:
I am okay
Use the cryptocode
Library to Encrypt a String in Python
The term cryptocode
is a simple library that lets us encrypt and decrypt strings securely and simply in Python 3 or above. Remember that this library needs to be manually installed; it can be done using the pip
command.
The program below uses the cryptocode
library to encrypt a string in Python:
import cryptocode
str_encoded = cryptocode.encrypt("I am okay", "wow")
# And then to decode it:
str_decoded = cryptocode.decrypt(str_encoded, "wow")
print(str_decoded)
Output:
I am okay
The first parameter in the function would be the string that needs to be encrypted. The second parameter needs to be the key, which will be used for the decryption purpose.
Use the Cryptography
Package to Encrypt a String in Python
Cryptography
is a Python package that can be used to achieve Symmetric-key Encryption. Symmetric-key Encryption is a way in which we use the same key for the encoding and decoding process.
The Cryptography
library needs to be installed in order to use this method for encryption; this can be done by using the pip
command.
The following code uses the cryptography
package functions to encrypt a string in Python:
from cryptography.fernet import Fernet
str1 = "I am okay"
key = Fernet.generate_key()
fernet = Fernet(key)
enctex = fernet.encrypt(str1.encode())
dectex = fernet.decrypt(enctex).decode()
print("The primordial string: ", str1)
print("The Encrypted message: ", enctex)
print("The Decrypted message: ", dectex)
Output:
The primordial string: I am okay
The Encrypted message: <a stack of random letters>
The Decrypted message: I am okay
In the code above, we import Fernet
from the cryptography.fernet
module. Then, we generate an encryption key that will be used for both encoding and decoding purposes. The Fernet class is instanced with the encryption key. The string is then encrypted with the Fernet instance. Finally, it is decrypted with the Fernet class instance.
Symmetric-key Encryption is an effortless way for encrypting a string. The only drawback is it’s comparatively less secure. Anyone with the key can read the encrypted text.
Use the RSA
Algorithm to Encrypt a String in Python
The RSA
algorithm in Python implements the Asymmetric-key Encryption. Asymmetric-key Encryption uses two different keys for the encryption and decryption process.
These two keys are the private key and public key. The Public Key is public and is used for the process of encryption. Anyone with the public key can encrypt and send the data; only the receiver has the private key. Additionally, someone with access to it can also decrypt the data.
The rsa
library needs to be installed first to use this method. You can use the general pip
command for the installation of this library.
The following code uses the RSA
algorithm to encrypt a string in Python:
import rsa
pubkey, privkey = rsa.newkeys(512)
str1 = "I am okay"
enctex = rsa.encrypt(str1.encode(), pubkey)
dectex = rsa.decrypt(enctex, privkey).decode()
print("The primordial string: ", str1)
print("The Encrypted message: ", enctex)
print("The Decrypted message: ", dectex)
Output:
The primordial string: I am okay
The Encrypted message: <a stack of random letters>
The Decrypted message: I am okay
In the above code, the rsa
module is imported, and the public and private keys are generated with the help of the rsa.newkeys()
function. Then, the string is encrypted using the public key. The string can then be decrypted by using only the private key. The public and private keys in the case of this program are pubkey
and privkey
, respectively.
Asymmetric-key Encryption provides better security than Symmetric-key Encryption. As in the former, a specific private key is only accessible to the receiver and is used for the decryption purpose. Whereas there exists only one key in the latter, making the decryption process easier and less secure from attacks by the third parties in the middle of the sender and the receiver.
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedInRelated Article - Python String
- How to Remove Commas From String in Python
- How to Check a String Is Empty in a Pythonic Way
- How to Convert a String to Variable Name in Python
- How to Remove Whitespace From a String in Python
- How to Extract Numbers From a String in Python
- How to Convert String to Datetime in Python