加密 Python 字符串
-
在 Python 中使用
Simple Crypt
加密字符串 -
在 Python 中使用
cryptocode
库加密字符串 -
在 Python 中使用
Cryptography
包加密字符串 -
在 Python 中使用
RSA
算法加密字符串
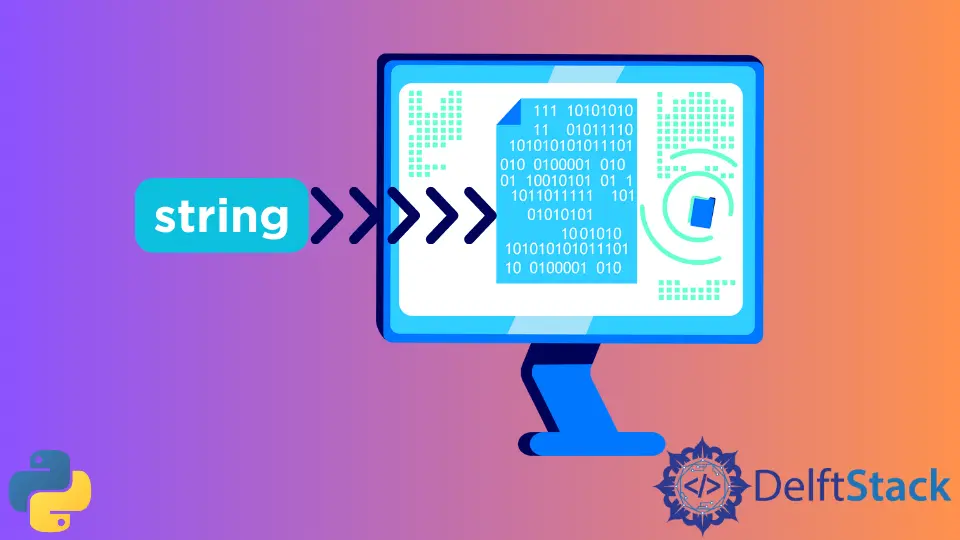
加密可以定义为将纯文本转换为密文的过程。本质上,它用于对数据进行编码。加密过程需要一个密钥,以后可以用它来解密原始消息。
本文将讨论可用于在 Python 中加密字符串的不同方法。
在 Python 中使用 Simple Crypt
加密字符串
使用 Simple Crypt
模块是实现 Python 2.7 和 3 加密过程的最快和最简单的方法。这种方法在几秒钟内将明文转换为密文,只需一行代码。
PyCrypto
模块为此库提供算法实现,它使用 AES256
密码。此方法将 HMAC
检查与 SHA256
密码结合在一起,以在密文被更改或修改时发出通知。
Simple Crypt
有两个函数:encrypt
和 decrypt
。必须同时安装 pycrypto
和 simplecrypt
模块才能使用这两个功能。
以下代码使用 simplecrypt
在 Python 中加密字符串:
from simplecrypt import encrypt, decrypt
passkey = "wow"
str1 = "I am okay"
cipher = encrypt(passkey, str1)
print(cipher)
此代码的输出将是一组随机字母。
要解码/解密密文,我们将使用以下命令:
print(decrypt("wow", cipher))
输出:
I am okay
在 Python 中使用 cryptocode
库加密字符串
术语 cryptocode
是一个简单的库,它允许我们在 Python 3 或更高版本中安全且简单地加密和解密字符串。记住这个库需要手动安装;可以使用 pip
命令来完成。
下面的程序使用 cryptocode
库来加密 Python 中的字符串:
import cryptocode
str_encoded = cryptocode.encrypt("I am okay", "wow")
# And then to decode it:
str_decoded = cryptocode.decrypt(str_encoded, "wow")
print(str_decoded)
输出:
I am okay
函数中的第一个参数是需要加密的字符串。第二个参数需要是密钥,用于解密目的。
在 Python 中使用 Cryptography
包加密字符串
Cryptography
是一个 Python 包,可用于实现对称密钥加密。对称密钥加密是我们在编码和解码过程中使用相同密钥的一种方式。
需要安装 Cryptography
库才能使用此方法进行加密;这可以通过使用 pip
命令来完成。
以下代码使用 cryptography
包函数在 Python 中加密字符串:
from cryptography.fernet import Fernet
str1 = "I am okay"
key = Fernet.generate_key()
fernet = Fernet(key)
enctex = fernet.encrypt(str1.encode())
dectex = fernet.decrypt(enctex).decode()
print("The primordial string: ", str1)
print("The Encrypted message: ", enctex)
print("The Decrypted message: ", dectex)
输出:
The primordial string: I am okay
The Encrypted message: <a stack of random letters>
The Decrypted message: I am okay
在上面的代码中,我们从 cryptography.fernet
模块中导入了 Fernet
。然后,我们生成将用于编码和解码目的的加密密钥。Fernet 类使用加密密钥进行实例化。然后使用 Fernet 实例对字符串进行加密。最后,用 Fernet 类实例解密。
对称密钥加密是加密字符串的一种轻松方式。唯一的缺点是它的安全性相对较低。任何拥有密钥的人都可以阅读加密文本。
在 Python 中使用 RSA
算法加密字符串
Python 中的 RSA
算法实现了非对称密钥加密。非对称密钥加密在加密和解密过程中使用两个不同的密钥。
这两个密钥是公钥和私钥。公钥是公开的,用于加密过程。任何拥有公钥的人都可以加密和发送数据;只有接收者拥有私钥。此外,有权访问它的人也可以解密数据。
需要先安装 rsa
库才能使用此方法。你可以使用一般的 pip
命令来安装这个库。
以下代码使用 RSA
算法在 Python 中加密字符串:
import rsa
pubkey, privkey = rsa.newkeys(512)
str1 = "I am okay"
enctex = rsa.encrypt(str1.encode(), pubkey)
dectex = rsa.decrypt(enctex, privkey).decode()
print("The primordial string: ", str1)
print("The Encrypted message: ", enctex)
print("The Decrypted message: ", dectex)
输出:
The primordial string: I am okay
The Encrypted message: <a stack of random letters>
The Decrypted message: I am okay
在上面的代码中,导入了 rsa
模块,并在 rsa.newkeys()
函数的帮助下生成了公钥和私钥。然后,使用 public key
对字符串进行加密。然后可以仅使用私钥解密该字符串。在这个程序的情况下,公钥和私钥分别是 pubkey
和 privkey
。
非对称密钥加密提供比对称密钥加密更好的安全性。与前者一样,特定的私钥只能由接收者访问并用于解密目的。而后者中只有一个密钥,这使得解密过程更容易,并且在发送方和接收方中间受到第三方攻击的安全性较低。
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn相关文章 - Python String
- 在 Python 中从字符串中删除逗号
- 如何以 Pythonic 的方式检查字符串是否为空
- 在 Python 中将字符串转换为变量名
- Python 如何去掉字符串中的空格/空白符
- 如何在 Python 中从字符串中提取数字
- Python 如何将字符串转换为时间日期 datetime 格式