Python 中的 RSA 加密
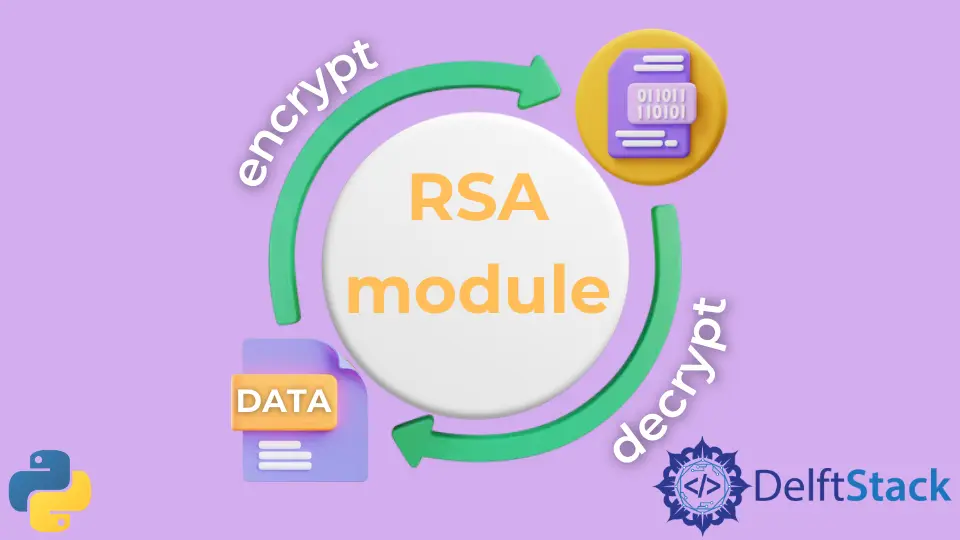
本文将解释使用 RSA
模块在 Python 中加密和解密数据的不同方法。
非对称加密方法使用一对密钥(公钥和私钥)在两个人之间进行安全对话。非对称或公钥密码学的优点是它还提供了一种方法来确保消息不被篡改并且是真实的。
我们可以通过以下方式使用 RSA
模块在 Python 中使用非对称加密。
Python 中使用普通加密的 RSA 加密
在 Python 中使用 RSA
模块执行非对称加密有两种方法:纯 RSA 加密和通过加密填充的更适当和安全的方法。
在纯 RSA 加密中,我们可以生成一个密钥对并使用公钥加密数据。我们可以使用 _RSAobj.encrypt()
方法加密数据,然后使用 _RSAobj.decrypt()
方法解密加密的消息。
_RSAobj.encrypt()
和 _RSAobj.decrypt()
方法都采用字节字符串或 long 作为输入,并分别对输入执行普通的 RSA 加密和解密。
下面的示例代码演示了如何使用 Python 中的普通 RSA 加密来加密和解密数据。
import Crypto
from Crypto.PublicKey import RSA
import ast
keyPair = RSA.generate(1024)
pubKey = keyPair.publickey()
encryptedMsg = pubKey.encrypt(b"This is my secret msg", 32)
decryptedMsg = keyPair.decrypt(ast.literal_eval(str(encryptedMsg)))
print("Decrypted message:", decryptedMsg)
Crypto
模块时出现错误,你可以使用 pip install pycrypto
命令安装它。Python 中使用加密填充的 RSA 加密
我们可以使用 Python 的 PKCS1_OAEP
模块执行 PKCS#1 OAEP 加密和解密。OAEP 是 RSA 发布的一种 Optimal Asymmetric Encryption Padding 方案,比普通和原始的 RSA 加密更安全。
要执行 OAEP 方案,我们首先必须生成 PKCS1OAEP_Cipher
对象,然后调用 PKCS1OAEP_Cipher.encrypt()
和 PKCS1OAEP_Cipher.decrypt()
方法来使用此方案加密或解密文本。如果输入文本是字符串类型,我们首先必须将其转换为字节字符串,因为字符串类型不是有效的输入类型。
下面的代码显示了在 Python 中使用 PKCS1_OAEP
模块的 OAEP 加密。
from Crypto.Cipher import PKCS1_OAEP
from Crypto.PublicKey import RSA
key = RSA.generate(2048)
privateKey = key.exportKey("PEM")
publicKey = key.publickey().exportKey("PEM")
message = "this is a top secret message!"
message = str.encode(message)
RSApublicKey = RSA.importKey(publicKey)
OAEP_cipher = PKCS1_OAEP.new(RSApublicKey)
encryptedMsg = OAEP_cipher.encrypt(message)
print("Encrypted text:", encryptedMsg)
RSAprivateKey = RSA.importKey(privateKey)
OAEP_cipher = PKCS1_OAEP.new(RSAprivateKey)
decryptedMsg = OAEP_cipher.decrypt(encryptedMsg)
print("The original text:", decryptedMsg)
Crypto
模块时出现错误,你可以使用 pip install pycrypto
命令安装它。