Python 中的 AES 加密
Fariba Laiq
2023年10月10日
Python
Python Encryption
Python Decryption
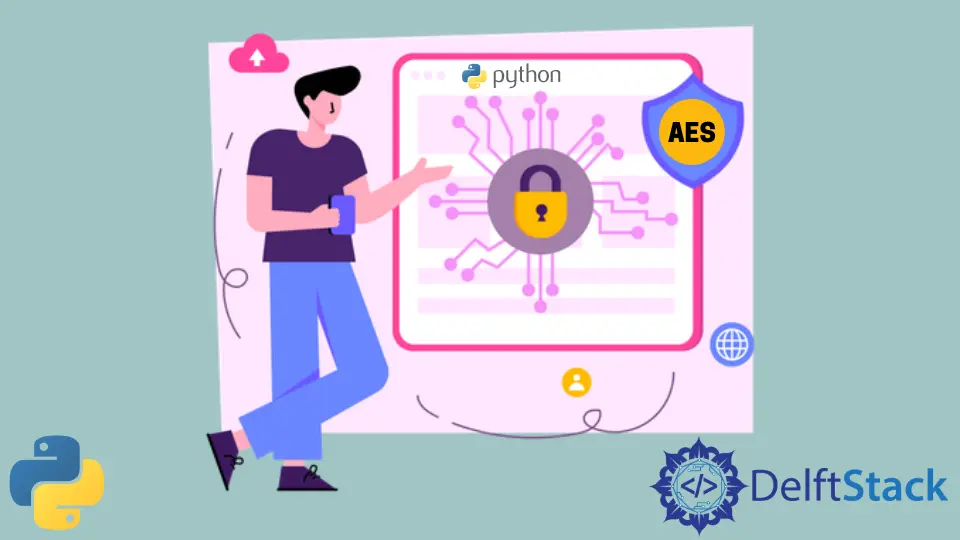
AES
(首字母缩写词 Advanced Encryption Standard
)是使用对称密钥加密的加密技术之一。
Python 中加密和解密的区别
加密是为了安全起见将(有意义的原始文本)转换为密文(无意义的文本)的过程,以便未经授权的一方无法看到原始消息。
解密将密文
转换为纯文本以获取原始消息。
发送者将使用密钥加密
消息,接收者将使用相同的密钥解密
消息。
在 Python 中 AES 256
使用 PyCrypto
PyCrypto
代表 Python Cryptography Toolkit
,一个带有与 cryptography
相关的内置功能的 Python 模块。
如果你使用的是 anaconda
,你可以安装此模块:
conda install -c anaconda pycrypto
Block size
设置为 16
,因为在 AES 中输入字符串应该是 16
的倍数。Padding
用于通过附加一些额外的字节来填充块。
填充是在加密之前完成的。解密后,我们取消填充密文
以丢弃额外的字节并获取原始消息。
在这里,我们创建了两个函数,encrypt
和 decrypt
,它们使用 AES
技术。
# Python 3.x
import base64
import hashlib
from Crypto.Cipher import AES
from Crypto import Random
BLOCK_SIZE = 16
def pad(s):
return s + (BLOCK_SIZE - len(s) % BLOCK_SIZE) * chr(
BLOCK_SIZE - len(s) % BLOCK_SIZE
)
def unpad(s):
return s[: -ord(s[len(s) - 1 :])]
def encrypt(plain_text, key):
private_key = hashlib.sha256(key.encode("utf-8")).digest()
plain_text = pad(plain_text)
print("After padding:", plain_text)
iv = Random.new().read(AES.block_size)
cipher = AES.new(private_key, AES.MODE_CBC, iv)
return base64.b64encode(iv + cipher.encrypt(plain_text))
def decrypt(cipher_text, key):
private_key = hashlib.sha256(key.encode("utf-8")).digest()
cipher_text = base64.b64decode(cipher_text)
iv = cipher_text[:16]
cipher = AES.new(private_key, AES.MODE_CBC, iv)
return unpad(cipher.decrypt(cipher_text[16:]))
message = input("Enter message to encrypt: ")
key = input("Enter encryption key: ")
encrypted_msg = encrypt(message, key)
print("Encrypted Message:", encrypted_msg)
decrypted_msg = decrypt(encrypted_msg, key)
print("Decrypted Message:", bytes.decode(decrypted_msg))
输出:
#Python 3.x
Enter message to encrypt: hello
Enter encryption key: 12345
After padding: hello
Encrypted Message: b'r3V0A0Ssjw/4ZOKL42/hWSQOLKy7lt9bOVt7D75RA3E='
Decrypted Message: hello
我们已要求用户输入 key
和 message
进行加密。在输出中,显示了加密和解密的消息。
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Fariba Laiq
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedIn