Python 中的 AES 加密
Fariba Laiq
2023年10月10日
Python
Python Encryption
Python Decryption
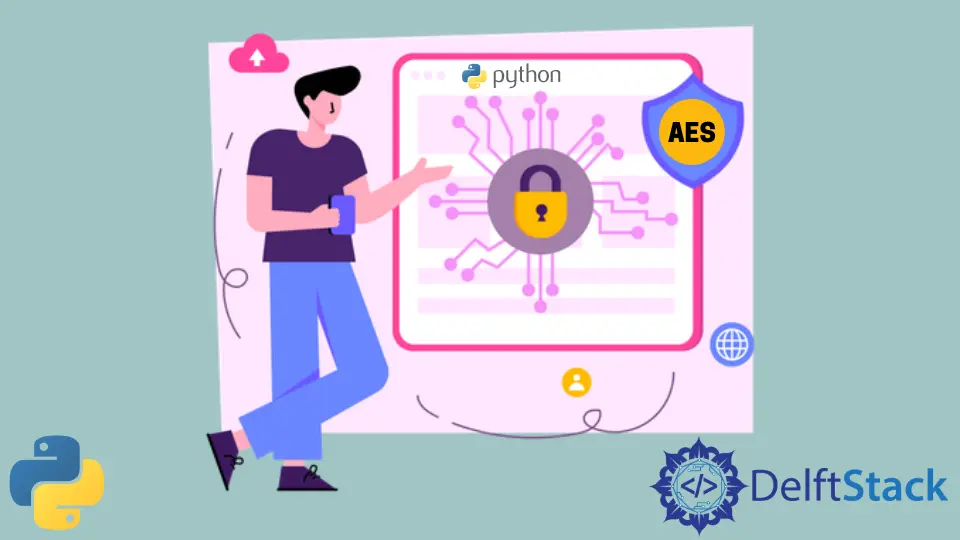
AES
(首字母縮寫詞 Advanced Encryption Standard
)是使用對稱金鑰加密的加密技術之一。
Python 中加密和解密的區別
加密是為了安全起見將(有意義的原始文字)轉換為密文(無意義的文字)的過程,以便未經授權的一方無法看到原始訊息。
解密將密文
轉換為純文字以獲取原始訊息。
傳送者將使用金鑰加密
訊息,接收者將使用相同的金鑰解密
訊息。
在 Python 中 AES 256
使用 PyCrypto
PyCrypto
代表 Python Cryptography Toolkit
,一個帶有與 cryptography
相關的內建功能的 Python 模組。
如果你使用的是 anaconda
,你可以安裝此模組:
conda install -c anaconda pycrypto
Block size
設定為 16
,因為在 AES 中輸入字串應該是 16
的倍數。Padding
用於通過附加一些額外的位元組來填充塊。
填充是在加密之前完成的。解密後,我們取消填充密文
以丟棄額外的位元組並獲取原始訊息。
在這裡,我們建立了兩個函式,encrypt
和 decrypt
,它們使用 AES
技術。
# Python 3.x
import base64
import hashlib
from Crypto.Cipher import AES
from Crypto import Random
BLOCK_SIZE = 16
def pad(s):
return s + (BLOCK_SIZE - len(s) % BLOCK_SIZE) * chr(
BLOCK_SIZE - len(s) % BLOCK_SIZE
)
def unpad(s):
return s[: -ord(s[len(s) - 1 :])]
def encrypt(plain_text, key):
private_key = hashlib.sha256(key.encode("utf-8")).digest()
plain_text = pad(plain_text)
print("After padding:", plain_text)
iv = Random.new().read(AES.block_size)
cipher = AES.new(private_key, AES.MODE_CBC, iv)
return base64.b64encode(iv + cipher.encrypt(plain_text))
def decrypt(cipher_text, key):
private_key = hashlib.sha256(key.encode("utf-8")).digest()
cipher_text = base64.b64decode(cipher_text)
iv = cipher_text[:16]
cipher = AES.new(private_key, AES.MODE_CBC, iv)
return unpad(cipher.decrypt(cipher_text[16:]))
message = input("Enter message to encrypt: ")
key = input("Enter encryption key: ")
encrypted_msg = encrypt(message, key)
print("Encrypted Message:", encrypted_msg)
decrypted_msg = decrypt(encrypted_msg, key)
print("Decrypted Message:", bytes.decode(decrypted_msg))
輸出:
#Python 3.x
Enter message to encrypt: hello
Enter encryption key: 12345
After padding: hello
Encrypted Message: b'r3V0A0Ssjw/4ZOKL42/hWSQOLKy7lt9bOVt7D75RA3E='
Decrypted Message: hello
我們已要求使用者輸入 key
和 message
進行加密。在輸出中,顯示了加密和解密的訊息。
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Fariba Laiq
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedIn