Python 中的 RSA 加密
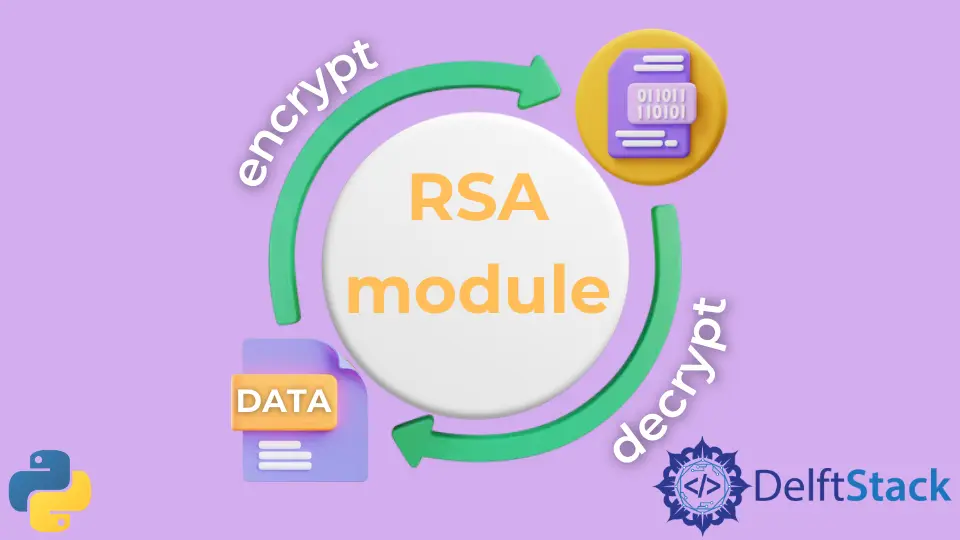
本文將解釋使用 RSA
模組在 Python 中加密和解密資料的不同方法。
非對稱加密方法使用一對金鑰(公鑰和私鑰)在兩個人之間進行安全對話。非對稱或公鑰密碼學的優點是它還提供了一種方法來確保訊息不被篡改並且是真實的。
我們可以通過以下方式使用 RSA
模組在 Python 中使用非對稱加密。
Python 中使用普通加密的 RSA 加密
在 Python 中使用 RSA
模組執行非對稱加密有兩種方法:純 RSA 加密和通過加密填充的更適當和安全的方法。
在純 RSA 加密中,我們可以生成一個金鑰對並使用公鑰加密資料。我們可以使用 _RSAobj.encrypt()
方法加密資料,然後使用 _RSAobj.decrypt()
方法解密加密的訊息。
_RSAobj.encrypt()
和 _RSAobj.decrypt()
方法都採用位元組字串或 long 作為輸入,並分別對輸入執行普通的 RSA 加密和解密。
下面的示例程式碼演示瞭如何使用 Python 中的普通 RSA 加密來加密和解密資料。
import Crypto
from Crypto.PublicKey import RSA
import ast
keyPair = RSA.generate(1024)
pubKey = keyPair.publickey()
encryptedMsg = pubKey.encrypt(b"This is my secret msg", 32)
decryptedMsg = keyPair.decrypt(ast.literal_eval(str(encryptedMsg)))
print("Decrypted message:", decryptedMsg)
Crypto
模組時出現錯誤,你可以使用 pip install pycrypto
命令安裝它。Python 中使用加密填充的 RSA 加密
我們可以使用 Python 的 PKCS1_OAEP
模組執行 PKCS#1 OAEP 加密和解密。OAEP 是 RSA 釋出的一種 Optimal Asymmetric Encryption Padding 方案,比普通和原始的 RSA 加密更安全。
要執行 OAEP 方案,我們首先必須生成 PKCS1OAEP_Cipher
物件,然後呼叫 PKCS1OAEP_Cipher.encrypt()
和 PKCS1OAEP_Cipher.decrypt()
方法來使用此方案加密或解密文字。如果輸入文字是字串型別,我們首先必須將其轉換為位元組字串,因為字串型別不是有效的輸入型別。
下面的程式碼顯示了在 Python 中使用 PKCS1_OAEP
模組的 OAEP 加密。
from Crypto.Cipher import PKCS1_OAEP
from Crypto.PublicKey import RSA
key = RSA.generate(2048)
privateKey = key.exportKey("PEM")
publicKey = key.publickey().exportKey("PEM")
message = "this is a top secret message!"
message = str.encode(message)
RSApublicKey = RSA.importKey(publicKey)
OAEP_cipher = PKCS1_OAEP.new(RSApublicKey)
encryptedMsg = OAEP_cipher.encrypt(message)
print("Encrypted text:", encryptedMsg)
RSAprivateKey = RSA.importKey(privateKey)
OAEP_cipher = PKCS1_OAEP.new(RSAprivateKey)
decryptedMsg = OAEP_cipher.decrypt(encryptedMsg)
print("The original text:", decryptedMsg)
Crypto
模組時出現錯誤,你可以使用 pip install pycrypto
命令安裝它。