How to Implement RSA Encryption in Python
- RSA Encryption in Python Using Plain Encryption
- RSA Encryption in Python Using Cryptographic Padding
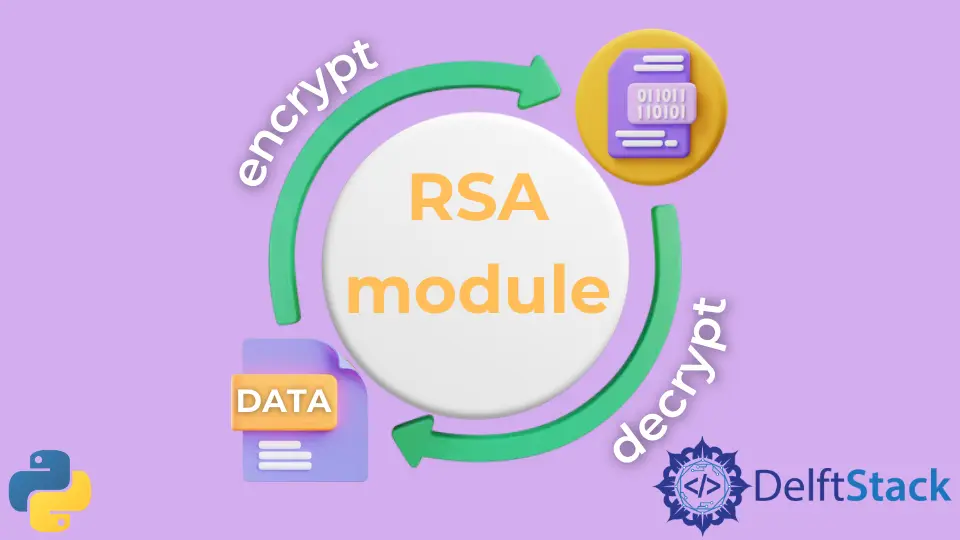
This article will explain different methods to encrypt and decrypt data in Python using the RSA
module.
The asymmetric cryptography method uses a pair of keys (public and private keys) for a secure conversation between two people. The advantage of asymmetric or public-key cryptography is that it also provides a method to ensure that the message is not tampered with and is authentic.
We can use asymmetric cryptography in Python using the RSA
module in the following ways.
RSA Encryption in Python Using Plain Encryption
There are two ways to perform asymmetric encryption using the RSA
module in Python: plain RSA encryption and a more proper and secure way by cryptographic padding.
In plain RSA encryption, we can generate a key pair and encrypt the data using the public key. We can encrypt the data using the _RSAobj.encrypt()
method, and then decrypt the encrypted message using the _RSAobj.decrypt()
method.
Both _RSAobj.encrypt()
and _RSAobj.decrypt()
methods take a byte string or long as the input and perform the plain RSA encryption and decryption on the input, respectively.
The below example code demonstrates how to encrypt and decrypt the data using the plain RSA encryption in Python.
import Crypto
from Crypto.PublicKey import RSA
import ast
keyPair = RSA.generate(1024)
pubKey = keyPair.publickey()
encryptedMsg = pubKey.encrypt(b"This is my secret msg", 32)
decryptedMsg = keyPair.decrypt(ast.literal_eval(str(encryptedMsg)))
print("Decrypted message:", decryptedMsg)
Crypto
module, you can install it by using the pip install pycrypto
command.RSA Encryption in Python Using Cryptographic Padding
We can perform the PKCS#1 OAEP encryption and decryption using Python’s PKCS1_OAEP
module. The OAEP is an Optimal Asymmetric Encryption Padding scheme published by RSA and is more secure than the plain and primitive RSA encryption.
To perform the OAEP scheme, we will first have to generate the PKCS1OAEP_Cipher
object and then call the PKCS1OAEP_Cipher.encrypt()
and PKCS1OAEP_Cipher.decrypt()
methods to encrypt or decrypt the text using this scheme. If the input text is a string type, we will first have to convert it into byte string, as string type is not a valid input type.
The code below shows the OAEP encryption using the PKCS1_OAEP
module in Python.
from Crypto.Cipher import PKCS1_OAEP
from Crypto.PublicKey import RSA
key = RSA.generate(2048)
privateKey = key.exportKey("PEM")
publicKey = key.publickey().exportKey("PEM")
message = "this is a top secret message!"
message = str.encode(message)
RSApublicKey = RSA.importKey(publicKey)
OAEP_cipher = PKCS1_OAEP.new(RSApublicKey)
encryptedMsg = OAEP_cipher.encrypt(message)
print("Encrypted text:", encryptedMsg)
RSAprivateKey = RSA.importKey(privateKey)
OAEP_cipher = PKCS1_OAEP.new(RSAprivateKey)
decryptedMsg = OAEP_cipher.decrypt(encryptedMsg)
print("The original text:", decryptedMsg)
Crypto
module, you can install it by using the pip install pycrypto
command.