AES Encryption in Python
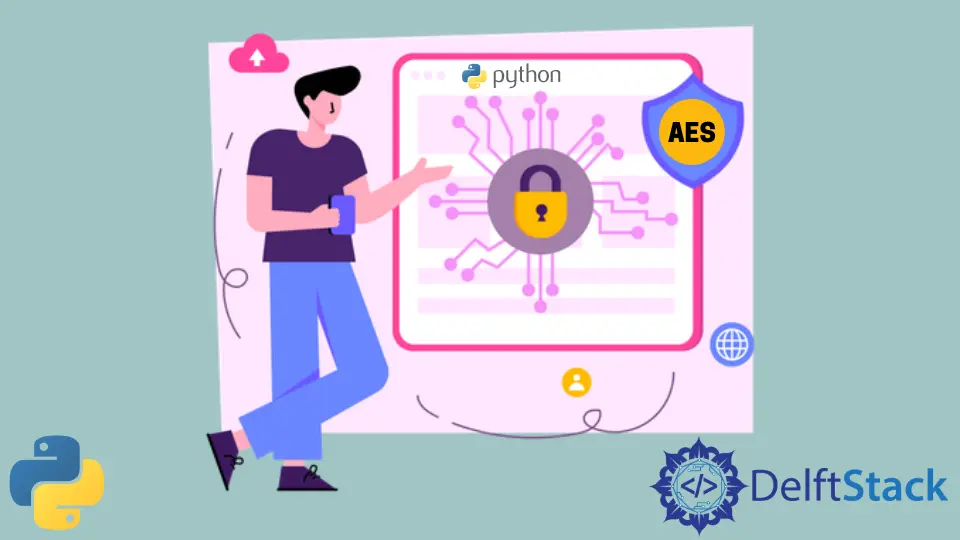
AES
(acronym Advanced Encryption Standard
) is one of the cryptography techniques that uses symmetric key encryption.
Difference Between Encryption and Decryption in Python
Encryption is the process of converting (original text that is meaningful) to ciphertext (meaningless text) for security so that an unauthorized party cannot see the original message.
Decryption converts the ciphertext
to plain text to get the original message.
The sender will encrypt
the message using a key, and the recipient will use the same key to decrypt
the message.
the AES 256
Using PyCrypto
in Python
PyCrypto
stands for Python Cryptography Toolkit
, a python module with built-in functionalities related to cryptography
.
If you are using anaconda
, you can install this module:
conda install -c anaconda pycrypto
Block size
is set to 16
because the input string should be a multiple of 16
in AES. Padding
is used to fill up the block by appending some additional bytes.
Padding is done before encryption. After decryption, we un-pad the ciphertext
to discard the additional bytes and get the original message.
Here, we have made two functions, encrypt
and decrypt
, which use the AES
technique.
# Python 3.x
import base64
import hashlib
from Crypto.Cipher import AES
from Crypto import Random
BLOCK_SIZE = 16
def pad(s):
return s + (BLOCK_SIZE - len(s) % BLOCK_SIZE) * chr(
BLOCK_SIZE - len(s) % BLOCK_SIZE
)
def unpad(s):
return s[: -ord(s[len(s) - 1 :])]
def encrypt(plain_text, key):
private_key = hashlib.sha256(key.encode("utf-8")).digest()
plain_text = pad(plain_text)
print("After padding:", plain_text)
iv = Random.new().read(AES.block_size)
cipher = AES.new(private_key, AES.MODE_CBC, iv)
return base64.b64encode(iv + cipher.encrypt(plain_text))
def decrypt(cipher_text, key):
private_key = hashlib.sha256(key.encode("utf-8")).digest()
cipher_text = base64.b64decode(cipher_text)
iv = cipher_text[:16]
cipher = AES.new(private_key, AES.MODE_CBC, iv)
return unpad(cipher.decrypt(cipher_text[16:]))
message = input("Enter message to encrypt: ")
key = input("Enter encryption key: ")
encrypted_msg = encrypt(message, key)
print("Encrypted Message:", encrypted_msg)
decrypted_msg = decrypt(encrypted_msg, key)
print("Decrypted Message:", bytes.decode(decrypted_msg))
Output:
#Python 3.x
Enter message to encrypt: hello
Enter encryption key: 12345
After padding: hello
Encrypted Message: b'r3V0A0Ssjw/4ZOKL42/hWSQOLKy7lt9bOVt7D75RA3E='
Decrypted Message: hello
We have asked the user to enter the key
and the message
to encrypt. In the output, the encrypted and decrypted message is shown.
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedIn