Imaginary Numbers in Python
- Initialize a Complex Number in Python
- Use the Complex Number Attributes and Functions in Python
- Use the Regular Mathematical Operations on a Complex Number in Python
-
Use the
cmath
Module Functions on Complex Numbers -
Use the
numpy.array()
Function to Store Imaginary Numbers in Arrays in Python
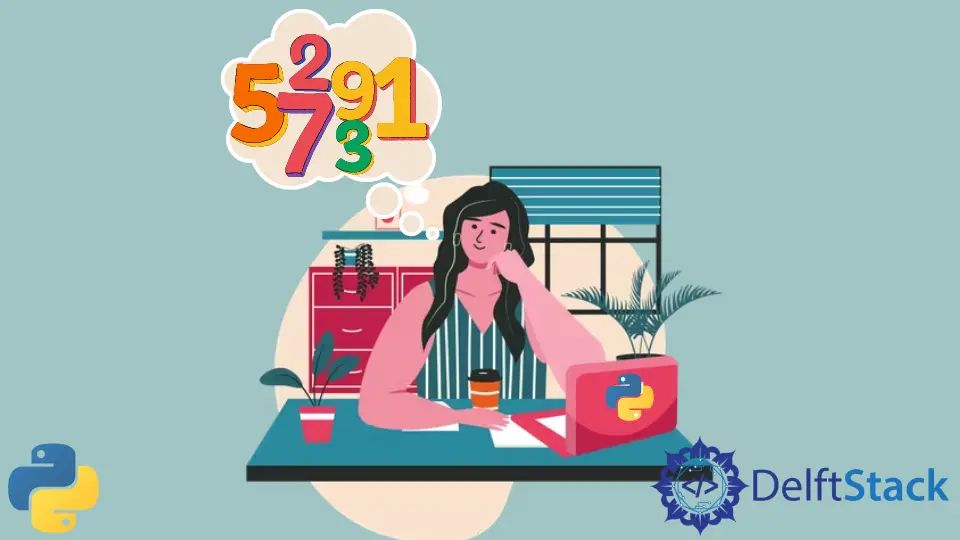
Python is a very versatile language for dealing with numerical data. It also supports working on both real and imaginary numbers.
In this tutorial, you’ll learn more about imaginary numbers and how to work with them in Python.
Initialize a Complex Number in Python
Complex numbers are comprised of a real part and an imaginary part. In Python, the imaginary part can be expressed by just adding a j
or J
after the number.
A complex number can be created easily: by directly assigning the real and imaginary part to a variable. The example code below demonstrates how you can create a complex number in Python:
a = 8 + 5j
print(type(a))
Output:
<class 'complex'>
We can also use the built-in complex()
function to convert the two given real numbers into a complex number.
a = 8
b = 5
c = complex(8, 5)
print(type(c))
Output:
<class 'complex'>
Now, the other half of the article will focus more on working with imaginary numbers in Python.
Use the Complex Number Attributes and Functions in Python
Complex numbers have a few built-in accessors that can be used for general information.
For example, to access the real part of a complex number, we can use the built-in real()
function and similarly use the imag()
function to access the imaginary part. Additionally, we can also find the conjugate of a complex number using the conjugate()
function.
a = 8 + 5j
print("Real Part = ", a.real)
print("Imaginary Part = ", a.imag)
print("Conjugate = ", a.conjugate())
Output:
Real Part = 8.0
Imaginary Part = 5.0
Conjugate = (8-5j)
Use the Regular Mathematical Operations on a Complex Number in Python
You can do basic mathematical operations like addition and multiplication on complex numbers in Python. The following code implements simple mathematical procedures on two given complex numbers.
a = 8 + 5j
b = 10 + 2j
# Adding imaginary part of both numbers
c = a.imag + b.imag
print(c)
# Simple multiplication of both complex numbers
print("after multiplication = ", a * b)
Output:
7.0
after multiplication = (70+66j)
Use the cmath
Module Functions on Complex Numbers
The cmath
module is a special module that provides access to several functions meant to be used on complex numbers. This module consists of a wide variety of functions. Some notable ones are the phase of a complex number, power and log functions, trigonometric functions, and hyperbolic functions.
The cmath
module also includes a couple of constants like pi
, tau
, Positive infinity
, and some more constants used in the calculations.
The following code implements some of the cmath
module functions on the complex number in Python:
import cmath
a = 8 + 5j
ph = cmath.phase(a)
print("Phase:", ph)
print("e^a is:", cmath.exp(a))
print("sine value of complex no.:\n", cmath.sin(a))
print("Hyperbolic sine is: \n", cmath.sinh(a))
Output:
Phase: 0.5585993153435624
e^a is: (845.5850573783163-2858.5129755252788j)
sine value of complex no.:
(73.42022455449552-10.796569647775932j)
Hyperbolic sine is:
(422.7924811101271-1429.2566486042679j)
Use the numpy.array()
Function to Store Imaginary Numbers in Arrays in Python
The term NumPy
is an abbreviation for Numerical Python. It’s a library provided by Python that deals with arrays and provides functions for operating on these arrays. As its name suggests, the numpy.array()
function is used in the creation of an array. The program below demonstrates how you can create an array of complex numbers in Python:
import numpy as np
arr = np.array([8 + 5j, 10 + 2j, 4 + 3j])
print(arr)
Output:
[8.+5.j 10.+2.j 4.+3.j]
Complex numbers are one of the three ways in which Python allows the storage and implementation of numerical data. It’s also considered an essential part of Python Programming. You can perform a wide variety of operations on complex numbers with Python Programming Language.
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn