How to Check if a File Exists in Python
-
try...except
to Check the File Existance (>Python 2.x) -
os.path.isfile()
to Check if File Exists (>=Python 2.x) -
pathlib.Path.is_file()
to Check if File Exists (>=Python 3.4)
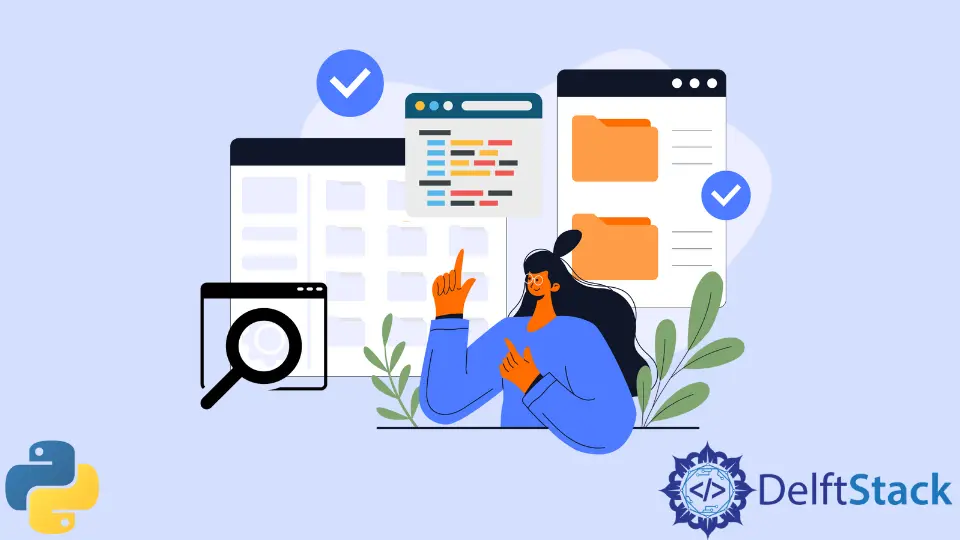
This tutorial will introduce three different solutions to check whether a file exists in Python.
try...except
block to check the file existance (>=Python 2.x)os.path.isfile
method to check the file existance (>=Python 2.x)pathlib.Path.is_file()
check the file existance (>=Python 3.4)
try...except
to Check the File Existance (>Python 2.x)
We could try to open the file and could check if file exists or not depending on whether the IOError
(in Python 2.x) or FileNotFoundError
(in Python 3.x) will be thrown or not.
def checkFileExistance(filePath):
try:
with open(filePath, "r") as f:
return True
except FileNotFoundError as e:
return False
except IOError as e:
return False
In the above example codes, we make it Python 2/3 compatible by listing both FileNotFoundError
and IOError
in the exception catching part.
os.path.isfile()
to Check if File Exists (>=Python 2.x)
import os
fileName = "temp.txt"
os.path.isfile(fileName)
It checks whether the file fileName
exists.
Some developers prefer to use the os.path.exists()
method to check whether a file exists. But it couldn’t distinguish whether the object is a file or a directory.
import os
fileName = "temp.txt"
print(os.path.exists(fileName))
fileName = r"C:\Test"
print(os.path.exists(fileName))
Therefore, only use os.path.isfile
if you want to check if the file exists.
pathlib.Path.is_file()
to Check if File Exists (>=Python 3.4)
Since Python 3.4, it introduces an object-oriented method in pathlib
module to check if a file exists.
from pathlib import Path
fileName = "temp.txt"
fileObj = Path(fileName)
print(fileObj.is_file())
Similarly, it has also is_dir()
and exists()
methods to check whether a directory or a file/directory exists.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook