Comment vérifier si un fichier existe en Python
-
try...except
pour vérifier l’existence du fichier (>Python 2.x) -
os.path.isfile()
pour vérifier si le fichier existe (>=Python 2.x) -
pathlib.Path.is_file()
pour vérifier si le fichier existe (>=Python 3.4)
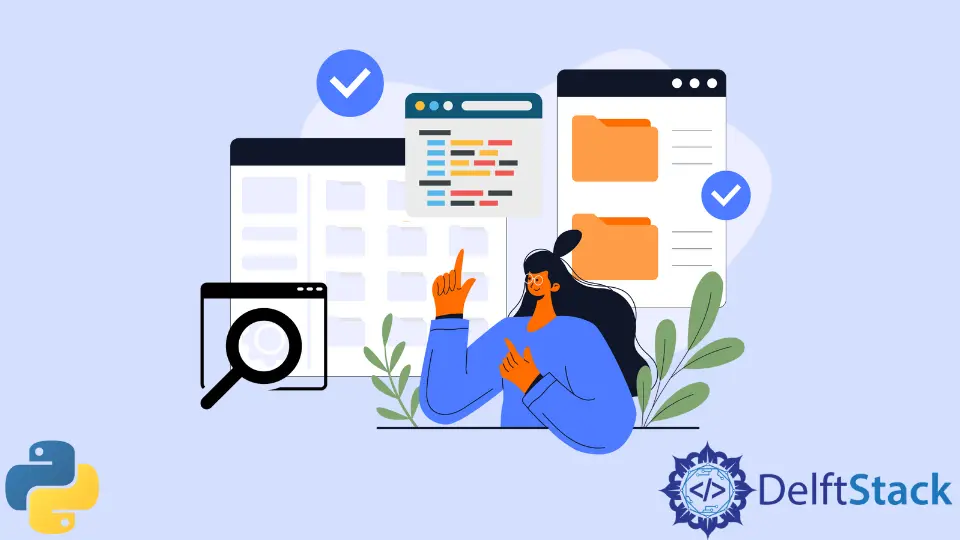
Ce tutoriel présente trois solutions différentes pour vérifier si un fichier existe en Python.
- Bloc
try...except
pour vérifier l’existence du fichier (>=Python 2.x) - Fonction
os.path.isfile
pour vérifier l’existence du fichier (>=Python 2.x) pathlib.Path.is_file()
vérifie l’existence du fichier (>=Python 3.4)
try...except
pour vérifier l’existence du fichier (>Python 2.x)
Nous pourrions essayer d’ouvrir le fichier et vérifier si le fichier existe ou non selon que IOError
(en Python 2.x) ou FileNotFoundError
(en Python 3.x) sera lancé ou non.
def checkFileExistance(filePath):
try:
with open(filePath, "r") as f:
return True
except FileNotFoundError as e:
return False
except IOError as e:
return False
Dans l’exemple de code ci-dessus, nous le rendons compatible avec Python 2/3 en listant à la fois FileNotFoundError
et IOError
dans la partie de capture des exceptions.
os.path.isfile()
pour vérifier si le fichier existe (>=Python 2.x)
import os
fileName = "temp.txt"
os.path.isfile(fileName)
Il vérifie si le fichier fileName
existe.
Certains développeurs préfèrent utiliser os.path.exists()
pour vérifier si un fichier existe. Mais il ne peut pas distinguer si l’objet est un fichier ou un répertoire.
import os
fileName = "temp.txt"
print(os.path.exists(fileName))
fileName = r"C:\Test"
print(os.path.exists(fileName))
Par conséquent, n’utilisez os.path.isfile
que si vous voulez vérifier si le fichier existe.
pathlib.Path.is_file()
pour vérifier si le fichier existe (>=Python 3.4)
Depuis Python 3.4, il introduit une méthode orientée objet dans le module pathlib
pour vérifier si un fichier existe.
from pathlib import Path
fileName = "temp.txt"
fileObj = Path(fileName)
print(fileObj.is_file())
De même, il a aussi les méthodes is_dir()
et exists()
pour vérifier si un répertoire ou un fichier/répertoire existe.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook