Como verificar se um arquivo existe em Python
-
try...except
para verificar a existência do arquivo (>=Python 2.x) -
os.path.isfile()
para verificar se o arquivo existe (>=Python 2.x) -
pathlib.Path.is_file()
para verificar se o arquivo existe (>=Python 3.4)
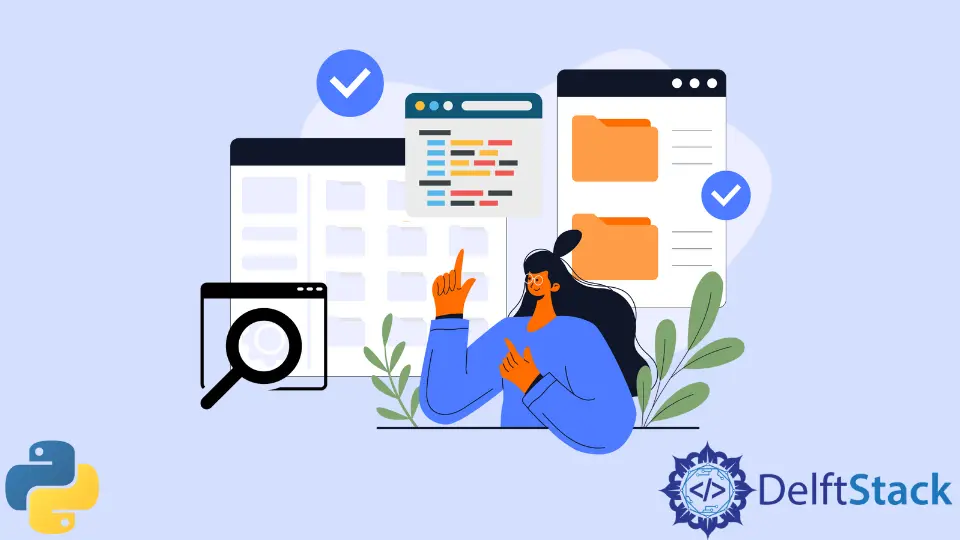
Este tutorial irá introduzir três soluções diferentes para verificar se um ficheiro existe em Python.
- Bloco
try...except
para verificar a existência do arquivo (>=Python 2.x) - Função
os.path.isfile
para verificar a existência do arquivo (>=Python 2.x) pathlib.Path.is_file()
verifica a existência do arquivo (>=Python 3.4)
try...except
para verificar a existência do arquivo (>=Python 2.x)
Podemos tentar abrir o arquivo e verificar se o arquivo existe ou não, dependendo se o IOError
(em Python 2.x) ou FileNotFoundError
(em Python 3.x) será lançado ou não.
def checkFileExistance(filePath):
try:
with open(filePath, "r") as f:
return True
except FileNotFoundError as e:
return False
except IOError as e:
return False
Nos exemplos acima, nós o tornamos compatível com Python 2/3 listando tanto o FileNotFoundError
quanto o IOError
na parte de captura de exceções.
os.path.isfile()
para verificar se o arquivo existe (>=Python 2.x)
import os
fileName = "temp.txt"
os.path.isfile(fileName)
Ele verifica se o arquivo fileName
existe.
Alguns desenvolvedores preferem utilizar os.path.exists()
para verificar se um arquivo existe. Mas não conseguia distinguir se o objeto é um arquivo ou um diretório.
import os
fileName = "temp.txt"
print(os.path.exists(fileName))
fileName = r"C:\Test"
print(os.path.exists(fileName))
Portanto, somente utilize os.path.isfile
se você quiser verificar se o file existe.
pathlib.Path.is_file()
para verificar se o arquivo existe (>=Python 3.4)
Desde Python 3.4, ele introduz um método orientado a objetos no módulo pathlib
para verificar se um arquivo existe.
from pathlib import Path
fileName = "temp.txt"
fileObj = Path(fileName)
print(fileObj.is_file())
Similarmente, ele também tem métodos is_dir()
e exists()
para verificar se um diretório ou um arquivo/diretório existe.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook