How to Convert String to ASCII Value in Python
-
Use the
for
Loop With theord()
Function to Get the ASCII Value of a String in Python -
Use List Comprehension and the
ord()
Function to Get the ASCII Value of a String in Python -
Use a User-Defined Function
to_ascii()
to Get the ASCII Value of a String in Python -
Use
map()
andlambda
Functions to Get the ASCII Value of a String in Python - Conclusion
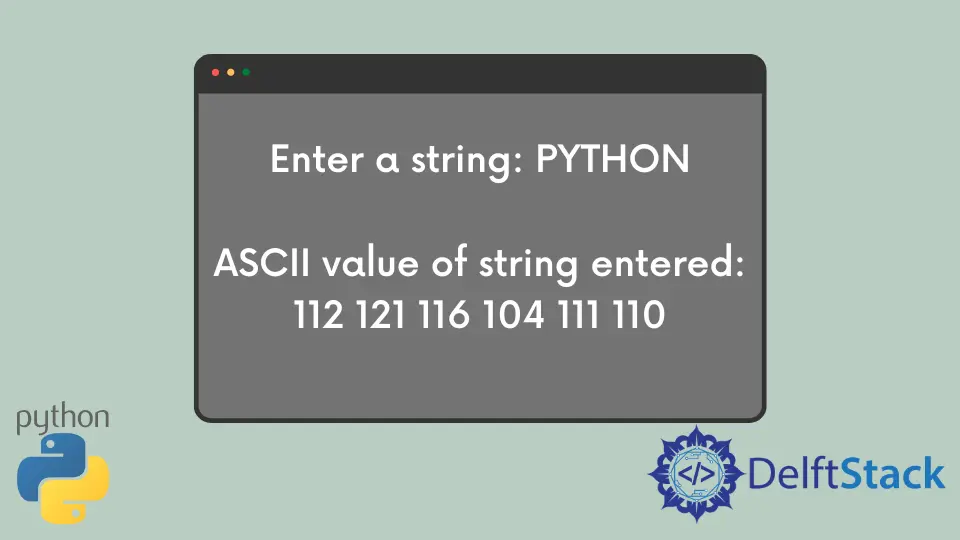
A string is one of the most utilized datatypes in any programming language, so much so that it can be mostly seen in almost every Python code. In Python, the ASCII value of a character is its numerical representation. It is a 7-bit number that is used to represent characters in computers.
Converting a string to ASCII values is a common task in Python programming, due to which there are multiple ways, be it direct or indirect, that can be utilized to complete this task.
Understanding how to perform this conversion allows you to work with the numerical representation of characters, enabling various text-processing operations. This tutorial will explore the numerous methods available to convert a string to ASCII in Python.
Use the for
Loop With the ord()
Function to Get the ASCII Value of a String in Python
We can use the for
loop and the ord()
function to get the ASCII value of the string. The ord()
function returns the Unicode of the passed string. It accepts 1
as the length of the string.
A for
loop is used for iterating over a sequence: a list, a tuple, a dictionary, a set, or a string. Therefore, we can use the for
loop to parse every string character and convert it into ASCII values.
In the code below, text
is a variable that holds the user input. The ascii_values
is an empty list initially, which will hold the ASCII values of each character in the string later.
Once the loop has completed its cycle, we will display the contents of ascii_values
as output to the user. After each iteration, the append()
function adds a new item to the list ascii_values
.
When we run this program, the user is prompted with a string, and once the user provides a string, it will be stored in a variable text
. In the example, the input is the string hello
. The ASCII value of each character of the string is printed.
Example Code:
text = input("enter a string to convert into ascii values:")
ascii_values = []
for character in text:
ascii_values.append(ord(character))
print(ascii_values)
Output:
Use List Comprehension and the ord()
Function to Get the ASCII Value of a String in Python
We can use the list comprehension to achieve the same result. List comprehension in Python is an easy and compact syntax for creating a list from a string or another list.
It is a concise way to create a new list by operating on each item in the existing list. The list comprehension is considerably faster than processing a list using the for
loop.
In the code below, there is no for
or while
loop externally. But within list comprehension, we use the for
loop to get each character
of the text
.
Example Code:
text = input("enter a string to convert into ascii values: ")
ascii_values = [ord(character) for character in text]
print(ascii_values)
Output:
Use a User-Defined Function to_ascii()
to Get the ASCII Value of a String in Python
Another way of writing the code to accomplish the same goal is to use a user-defined function. User-defined functions are functions you use to organize your code in the body of a policy.
Once you define a function, you can call it similar to the built-in action and parser functions. Variables passed to a function are passed by reference rather than by value.
In the code below, we use a user-defined function to_ascii
to take text
as a parameter. Block operation is defined inside the function, and the keyword return
transfers the result.
When the function to_ascii
is called from the main module providing text
as parameter control transfers to the to_ascii
function and the code block is executed, we get ASCII values of the given string in a list.
Example Code:
def to_ascii(text):
ascii_values = [ord(character) for character in text]
return ascii_values
text = input("Enter a string: ")
print(to_ascii(text))
Output:
Use map()
and lambda
Functions to Get the ASCII Value of a String in Python
The map()
function can be utilized to return a map object of the net result subsequently post applying the given function on every item of an iterable, and the lambda
function is an anonymous function that is capable of taking in many arguments but only relies on a single expression.
The map()
function, in combination with a lambda
function, allows you to apply the ord()
function to each character in a string.
Example Code:
str1 = "LeBron James"
ls1 = list(map(lambda x: ord(x), str1))
print(ls1)
Output:
Conclusion
Converting a string to ASCII values is a valuable skill in Python programming, enabling various text-processing operations. This comprehensive guide explored different methods for converting a string to ASCII, including using the ord()
function, list comprehension, creating a user-defined function, and mapping with map()
and lambda
functions.
Each method has advantages, and the choice depends on your requirements. By mastering these techniques, you can efficiently work with the numerical representation of characters and perform text-processing tasks in your Python projects.
With these methods in your toolkit, you are well-equipped to handle string-to-ASCII conversions and build more robust and dynamic applications.
Related Article - Python ASCII
- How to Convert Int to ASCII in Python
- How to Convert Hex to ASCII in Python
- How to Get ASCII Value of a Character in Python