How to Calculate the Arithmetic Mean in Python
- Method 1: Using the Mathematical Formula
- Method 2: Using the Statistics Library
- Method 3: Using NumPy
- Method 4: Using SciPy
- Conclusion
- FAQ
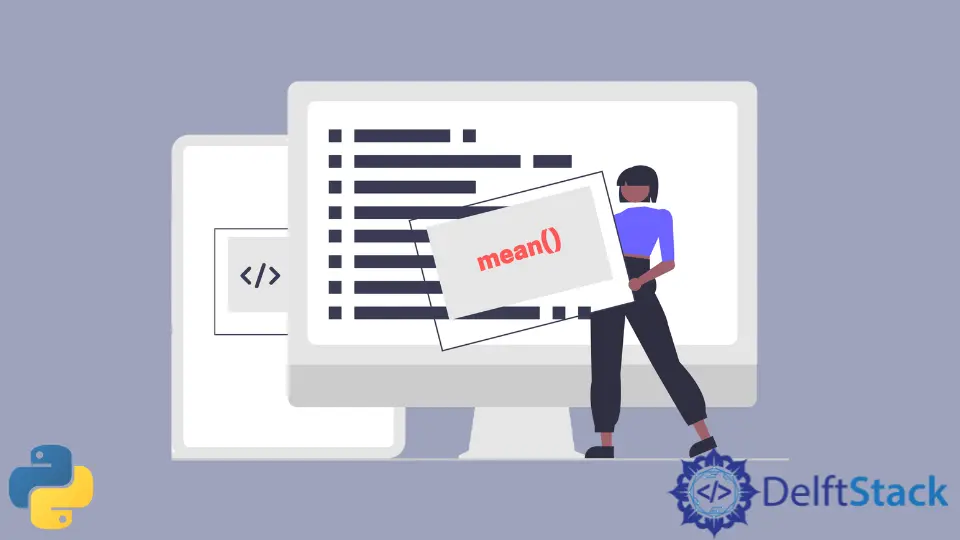
Calculating the arithmetic mean is a fundamental concept in statistics and data analysis. In Python, there are multiple ways to compute the mean of a dataset, which can be particularly useful for data scientists and analysts. Whether you prefer using mathematical formulas or leveraging Python’s powerful libraries like NumPy, Statistics, and SciPy, this article will guide you through the various methods. By the end, you’ll have a solid understanding of how to calculate the mean in Python, enabling you to apply this knowledge to your own projects. Let’s dive in!
Method 1: Using the Mathematical Formula
Calculating the arithmetic mean using the mathematical formula is straightforward. The formula for the mean is the sum of all numbers divided by the count of the numbers. Here’s how you can implement this in Python:
data = [10, 20, 30, 40, 50]
mean = sum(data) / len(data)
print(mean)
Output:
30.0
In this code snippet, we first define a list called data
containing five numerical values. We then calculate the mean by using the sum()
function to get the total of the numbers in the list and divide that by the length of the list, which we obtain using len()
. Finally, we print the result. This method is simple yet effective for smaller datasets, allowing you to quickly find the mean without relying on external libraries.
Method 2: Using the Statistics Library
Python’s built-in statistics
library offers a convenient way to calculate the mean. This method is particularly useful for larger datasets or when you want to ensure accuracy with built-in functions. Here’s how you can use it:
import statistics
data = [10, 20, 30, 40, 50]
mean = statistics.mean(data)
print(mean)
Output:
30
In this example, we start by importing the statistics
library. The mean()
function from this library takes a list of numbers as input and returns the arithmetic mean. This method is not only concise but also handles edge cases, such as empty lists, more gracefully than a manual implementation. By using the statistics
library, you can ensure that your calculations are both efficient and reliable, making it a preferred choice for many Python developers.
Method 3: Using NumPy
NumPy is one of the most popular libraries for numerical computations in Python. It provides a highly efficient way to calculate the mean, especially when working with large datasets. Here’s how you can compute the mean using NumPy:
import numpy as np
data = [10, 20, 30, 40, 50]
mean = np.mean(data)
print(mean)
Output:
30.0
In this code, we first import the NumPy library as np
. The np.mean()
function is then used to calculate the mean of the data
list. One of the significant advantages of using NumPy is its performance with large arrays. It can handle multi-dimensional data structures, making it an essential tool for data analysis and scientific computing. If you’re working with extensive datasets or require advanced mathematical operations, NumPy is definitely the way to go.
Method 4: Using SciPy
SciPy is another powerful library in Python that builds on NumPy and provides additional functionalities for scientific and technical computing. While it is often used for more complex mathematical operations, you can also calculate the mean using SciPy. Here’s how:
from scipy import stats
data = [10, 20, 30, 40, 50]
mean = stats.tmean(data)
print(mean)
Output:
30.0
In this example, we import the stats
module from the SciPy library. The tmean()
function computes the arithmetic mean, and it can also handle data with specific conditions. While it may seem like overkill for simply calculating the mean, SciPy provides a robust framework for more complex statistical analyses. If you’re already using SciPy for other tasks, it makes sense to utilize its capabilities for calculating the mean as well.
Conclusion
Calculating the arithmetic mean in Python can be done through various methods, each with its own advantages. Whether you choose to implement the mathematical formula directly or use libraries like Statistics, NumPy, or SciPy, understanding these methods will enhance your data analysis skills. By mastering these techniques, you can efficiently analyze datasets and derive meaningful insights, which is essential in today’s data-driven world. Now that you have the tools at your disposal, it’s time to put them into practice!
FAQ
-
What is the arithmetic mean?
The arithmetic mean is the sum of a set of numbers divided by the count of numbers in the set. -
Which Python library is best for calculating the mean?
It depends on your needs. For simple calculations, the statistics library is sufficient. For larger datasets, NumPy is recommended. -
Can I calculate the mean of a list with non-numeric values?
No, the mean can only be calculated for numeric values. Attempting to do so will result in an error. -
Is there a way to calculate the mean without importing any libraries?
Yes, you can use the mathematical formula by summing the values and dividing by the count, as shown in the first method. -
Does the mean always represent the data accurately?
Not always. The mean can be skewed by outliers, so it’s important to consider other measures of central tendency, like the median.